here some pics
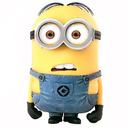
michlb1982
@michlb1982
Best posts made by michlb1982
-
RE: Magnetic field detection
Hello
thanks for the input...
well i did it...
i was able to build an Wind-direction-MYSENSOR... well i'm sure, its a little bit
awkward and much to complicated but it works quite OK...
the next step is to build a windspeed - sensor.. with the AS5040..here the Arduino nano Code...:
#include <SPI.h> #include <MySensor.h> const int ledPin = 13; //LED connected to digital pin 13 const int clockPin = 5; //output to clock const int CSnPin = 4; //output to chip select const int inputPin = 6; //read AS5040 int inputstream = 0; //one bit read from pin long packeddata = 0; //two bytes concatenated from inputstream long angle = 0; //holds processed angle value long anglemask = 65472; //0x1111111111000000: mask to obtain first 10 digits with position info long statusmask = 63; //0x000000000111111; mask to obtain last 6 digits containing status info long statusbits; //holds status/error information int DECn; //bit holding decreasing magnet field error data int INCn; //bit holding increasing magnet field error data int OCF; //bit holding startup-valid bit int COF; //bit holding cordic DSP processing error data int LIN; //bit holding magnet field displacement error data int debug = 0; //SET THIS TO 0 TO DISABLE PRINTING OF ERROR CODES int shortdelay = 1000; // this is the microseconds of delay in the data clock int longdelay =1000; // this is the milliseconds between readings #define CHILD_ID_DIR 0 //#define INTERRUPT inputPin MySensor gw; MyMessage msgDIR(CHILD_ID_DIR, V_DIRECTION); void setup() { gw.begin(NULL, AUTO, true, AUTO); Serial.begin(250000); pinMode(ledPin, OUTPUT); // visual signal of I/O to chip pinMode(clockPin, OUTPUT); // SCK pinMode(CSnPin, OUTPUT); // CSn -- has to toggle high and low to signal chip to start data transfer pinMode(inputPin, INPUT); // SDA // Send the Sketch Version Information to the Gateway gw.sendSketchInfo("Winddirection", "1.0"); // Register all sensors to gw (they will be created as child devices) gw.present(CHILD_ID_DIR, S_WIND); } void loop() { // CSn needs to cycle from high to low to initiate transfer. Then clock cycles. As it goes high // again, data will appear on sda digitalWrite(CSnPin, HIGH); // CSn high digitalWrite(clockPin, HIGH); // CLK high delay(longdelay);// time between readings digitalWrite(ledPin, HIGH); // signal start of transfer with LED digitalWrite(CSnPin, LOW); // CSn low: start of transfer delayMicroseconds(shortdelay); // delay for chip initialization digitalWrite(clockPin, LOW); // CLK goes low: start clocking delayMicroseconds(shortdelay); // hold low for (int x=0; x <16; x++) // clock signal, 16 transitions, output to clock pin { digitalWrite(clockPin, HIGH); //clock goes high delayMicroseconds(shortdelay); // inputstream =digitalRead(inputPin); // read one bit of data from pin //Serial.print(inputstream, DEC); packeddata = ((packeddata << 1) + inputstream);// left-shift summing variable, add pin value digitalWrite(clockPin, LOW); delayMicroseconds(shortdelay); // end of one clock cycle } // end of entire clock cycle //Serial.println(" "); digitalWrite(ledPin, LOW); // signal end of transmission // lots of diagnostics for verifying bitwise operations //Serial.print("packed:"); //Serial.println(packeddata,DEC); //Serial.print("pack bin: "); //Serial.println(packeddata,BIN); angle = packeddata & anglemask; // mask rightmost 6 digits of packeddata to zero, into angle. //Serial.print("mask: "); //Serial.println(anglemask, BIN); //Serial.print("bin angle:"); //Serial.println(angle, BIN); //Serial.print("angle: "); //Serial.println(angle, DEC); angle = (angle >> 6); // shift 16-digit angle right 6 digits to form 10-digit value //Serial.print("angleshft:"); //Serial.println(angle, BIN); //Serial.print("angledec: "); //Serial.println(angle, DEC); angle = angle * 0.3515; // angle * (360/1024) == actual degrees Serial.print("angle: "); // and, finally, print it. Serial.println(angle, DEC); //Serial.println("--------------------"); //Serial.print("raw: "); // this was the prefix for the bit-by-bit diag output inside the loop. if (debug) { statusbits = packeddata & statusmask; DECn = statusbits & 2; // goes high if magnet moved away from IC INCn = statusbits & 4; // goes high if magnet moved towards IC LIN = statusbits & 8; // goes high for linearity alarm COF = statusbits & 16; // goes high for cordic overflow: data invalid OCF = statusbits & 32; // this is 1 when the chip startup is finished. if (DECn && INCn) { Serial.println("magnet moved out of range"); } else { if (DECn) { Serial.println("magnet moved away from chip"); } if (INCn) { Serial.println("magnet moved towards chip"); } } if (LIN) { Serial.println("linearity alarm: magnet misaligned? Data questionable."); } if (COF) { Serial.println("cordic overflow: magnet misaligned? Data invalid."); } } float V_DIRECTION = angle; gw.send(msgDIR.set(V_DIRECTION, DEC)); packeddata = 0; // reset both variables to zero so they don't just accumulate angle = 0; gw.process(); //gw.sleep(INTERRUPT,CHANGE); gw.sleep(longdelay); }
the code for FHEM:
define MYSENSOR_111 MYSENSORS_DEVICE 111 attr MYSENSOR_111 IODev SerialGate attr MYSENSOR_111 alias Windrichtung attr MYSENSOR_111 group Sensoren attr MYSENSOR_111 mapReading_direction 0 direction attr MYSENSOR_111 mapReading_gust 0 gust attr MYSENSOR_111 mapReading_wind 0 wind attr MYSENSOR_111 mode repeater attr MYSENSOR_111 room 03_Umwelt attr MYSENSOR_111 stateFormat {sprintf("%.0f",ReadingsVal("MYSENSOR_111","direction",0))."°"}
a curcuit will follow in the next days...
greets..
Mike -
RE: DHT12 + 2Relay + 2Button - Combining Problem
@rejoe2
thanks for the link.. i will try... and report if it worked.. -
RE: Mysensort mit DHT12 (i2C)
Well i did it... i've got a working MYSENSOR with DHT12...
here the Code ... its not nice but it works...// Enable debug prints #define MY_DEBUG // Define Node ID #define MY_NODE_ID 128 //#define MY_REPEATER_FEATURE // Enable and select radio type attached #define MY_RADIO_NRF24 #include <SPI.h> #include <MySensors.h> #include <DHT12.h> #include <Wire.h> DHT12 dht12; #define SENSOR_TEMP_OFFSET 0 // Sleep time between sensor updates (in milliseconds) // Must be >1000ms for DHT22 and >2000ms for DHT11 static const uint64_t UPDATE_INTERVAL = 30000; static const uint8_t FORCE_UPDATE_N_READS = 10; #define CHILD_ID_HUM 0 #define CHILD_ID_TEMP 1 float lastTemp; float lastHum; uint8_t nNoUpdatesTemp; uint8_t nNoUpdatesHum; bool metric = true; MyMessage msgHum(CHILD_ID_HUM, V_HUM); MyMessage msgTemp(CHILD_ID_TEMP, V_TEMP); void presentation() { // Send the sketch version information to the gateway sendSketchInfo("DHT12", "1.1"); // Register all sensors to gw (they will be created as child devices) present(CHILD_ID_HUM, S_HUM); present(CHILD_ID_TEMP, S_TEMP); //metric = getControllerConfig().isMetric; } void setup() { Wire.begin(); } void loop() { // Force reading sensor, so it works also after sleep() //dht12.readSensor(true); // Get temperature from DHT library float temperature = dht12.readTemperature(); if (isnan(temperature)) { Serial.println("Failed reading temperature from DHT!"); } else if (temperature != lastTemp || nNoUpdatesTemp == FORCE_UPDATE_N_READS) { // Only send temperature if it changed since the last measurement or if we didn't send an update for n times lastTemp = temperature; // apply the offset before converting to something different than Celsius degrees temperature += SENSOR_TEMP_OFFSET; if (!metric) { temperature = dht12.readTemperature(); } // Reset no updates counter nNoUpdatesTemp = 0; send(msgTemp.set(temperature, 1)); #ifdef MY_DEBUG Serial.print("T: "); Serial.println(temperature); #endif } else { // Increase no update counter if the temperature stayed the same nNoUpdatesTemp++; } // Get humidity from DHT library float humidity = dht12.readHumidity(); if (isnan(humidity)) { Serial.println("Failed reading humidity from DHT"); } else if (humidity != lastHum || nNoUpdatesHum == FORCE_UPDATE_N_READS) { // Only send humidity if it changed since the last measurement or if we didn't send an update for n times lastHum = humidity; // Reset no updates counter nNoUpdatesHum = 0; send(msgHum.set(humidity, 1)); #ifdef MY_DEBUG Serial.print("H: "); Serial.println(humidity); #endif } else { // Increase no update counter if the humidity stayed the same nNoUpdatesHum++; } // Sleep for a while to save energy sleep(UPDATE_INTERVAL); //wait(UPDATE_INTERVAL); //for repeaters }
i Used this library: https://github.com/Bobadas/DHT12_library_Arduino/blob/master/README.md
here the datasheet of the dht12: http://www.robototehnika.ru/file/DHT12.pdf
-
RE: Magnetic field detection
well, the promised curcuit...Windrichtung-AS5040-Mysensor.pdf
Windrichtung.fzz
-
RE: DHT12 + 2Relay + 2Button - Combining Problem
@mfalkvidd you are so right!!! the "wait" is the problem... i changed the script.. i use a if to check if the update-interval is over and then i go through the dht loop....
now the bounce are also working..!here my code...
#define MY_DEBUG #define MY_RADIO_NRF24 //#define MY_REPEATER_FEATURE // Define Node ID #define MY_NODE_ID 101 #define RELAY_ON 1 #define RELAY_OFF 0 #define SSR_A_ID 1 // Id of the sensor child #define SSR_B_ID 2 // Id of the sensor child #define CHILD_ID_HUM 0 #define CHILD_ID_TEMP 0//1 #define SENSOR_TEMP_OFFSET 0 #include <SPI.h> #include <MySensors.h> #include <Bounce2.h> #include <DHT12.h> #include <Wire.h> const int buttonPinA = 7; const int buttonPinB = 8; const int relayPinA = 6; const int relayPinB = 5; int oldValueA = 0; int oldValueB = 0; unsigned long startMillis; unsigned long currentMillis; bool stateA = false; bool stateB = false; float lastTemp; float lastHum; uint8_t nNoUpdatesTemp; uint8_t nNoUpdatesHum; bool metric = true; static const uint8_t FORCE_UPDATE_N_READS = 10;//10 static const uint64_t UPDATE_INTERVAL = 30000;//30000 Bounce debouncerA = Bounce(); Bounce debouncerB = Bounce(); MyMessage msgA(SSR_A_ID, V_STATUS); MyMessage msgB(SSR_B_ID, V_STATUS); MyMessage msgHum(CHILD_ID_HUM, V_HUM); MyMessage msgTemp(CHILD_ID_TEMP, V_TEMP); DHT12 dht12; void setup() { //Serial.begin(115200); Wire.begin(); pinMode(buttonPinA, INPUT_PULLUP); // Setup the button Activate internal pull-up pinMode(buttonPinB, INPUT_PULLUP); // Setup the button Activate internal pull-up // After setting up the buttons, setup debouncer debouncerA.attach(buttonPinA); debouncerA.interval(5); //5 debouncerB.attach(buttonPinB); debouncerB.interval(5);//5 // Make sure relays are off when starting up digitalWrite(relayPinA, RELAY_ON); digitalWrite(relayPinB, RELAY_ON); // Then set relay pins in output mode pinMode(relayPinA, OUTPUT); pinMode(relayPinB, OUTPUT); } void presentation() { // Send the sketch version information to the gateway and Controller sendSketchInfo("2xRelais,2xButton,DHT12", "1.2a"); // Register all sensors to gw (they will be created as child devices) present(SSR_A_ID, S_LIGHT); present(SSR_B_ID, S_LIGHT); present(CHILD_ID_HUM, S_HUM); present(CHILD_ID_TEMP, S_TEMP); metric = getControllerConfig().isMetric; } /* Example on how to asynchronously check for new messages from gw */ void loop() { currentMillis = millis(); debouncerA.update(); // Get the update value int valueA = debouncerA.read(); if (valueA != oldValueA) { send(msgA.set(stateA ? false : true), true); // Send new state and request ack back oldValueA = valueA; } debouncerB.update(); // Get the update value int valueB = debouncerB.read(); if (valueB != oldValueB) { send(msgB.set(stateB ? false : true), true); // Send new state and request ack back oldValueB = valueB; } // Force reading sensor, so it works also after sleep() // dht12.readSensor(true); if (currentMillis - startMillis >= UPDATE_INTERVAL) { // Get temperature from DHT library float temperature = dht12.readTemperature(); if (isnan(temperature)) { Serial.println("Failed reading temperature from DHT!"); } else if (temperature != lastTemp || nNoUpdatesTemp == FORCE_UPDATE_N_READS) { // Only send temperature if it changed since the last measurement or if we didn't send an update for n times lastTemp = temperature; // apply the offset before converting to something different than Celsius degrees temperature += SENSOR_TEMP_OFFSET; if (!metric) { temperature = dht12.readTemperature(); } // Reset no updates counter nNoUpdatesTemp = 0; send(msgTemp.set(temperature, 1)); #ifdef MY_DEBUG Serial.print("T: "); Serial.println(temperature); #endif } else { // Increase no update counter if the temperature stayed the same nNoUpdatesTemp++; } // Get humidity from DHT library float humidity = dht12.readHumidity(); if (isnan(humidity)) { Serial.println("Failed reading humidity from DHT"); } else if (humidity != lastHum || nNoUpdatesHum == FORCE_UPDATE_N_READS) { // Only send humidity if it changed since the last measurement or if we didn't send an update for n times lastHum = humidity; // Reset no updates counter nNoUpdatesHum = 0; send(msgHum.set(humidity, 1)); #ifdef MY_DEBUG Serial.print("H: "); Serial.println(humidity); #endif } else { // Increase no update counter if the humidity stayed the same nNoUpdatesHum++; } startMillis = currentMillis; Serial.print("Startzeit: "); Serial.println(startMillis); } // Sleep for a while to save energy //wait(UPDATE_INTERVAL); } void receive(const MyMessage &message) { // We only expect one type of message from controller. But we better check anyway. if (message.type == V_STATUS) { switch (message.sensor) { case 1: stateA = message.getBool(); digitalWrite(message.sensor + 4, stateA ? RELAY_ON : RELAY_OFF); break; case 2: stateB = message.getBool(); digitalWrite(message.sensor + 4, stateB ? RELAY_ON : RELAY_OFF); break; } // Write some debug info Serial.print("Incoming change for sensor:"); Serial.println(message.sensor); //Serial.print("from node:"); //Serial.println(message.sender); //Serial.print(", New status: "); Serial.println(message.getBool()); } }
Latest posts made by michlb1982
-
RE: DHT12 + 2Relay + 2Button - Combining Problem
@mfalkvidd you are so right!!! the "wait" is the problem... i changed the script.. i use a if to check if the update-interval is over and then i go through the dht loop....
now the bounce are also working..!here my code...
#define MY_DEBUG #define MY_RADIO_NRF24 //#define MY_REPEATER_FEATURE // Define Node ID #define MY_NODE_ID 101 #define RELAY_ON 1 #define RELAY_OFF 0 #define SSR_A_ID 1 // Id of the sensor child #define SSR_B_ID 2 // Id of the sensor child #define CHILD_ID_HUM 0 #define CHILD_ID_TEMP 0//1 #define SENSOR_TEMP_OFFSET 0 #include <SPI.h> #include <MySensors.h> #include <Bounce2.h> #include <DHT12.h> #include <Wire.h> const int buttonPinA = 7; const int buttonPinB = 8; const int relayPinA = 6; const int relayPinB = 5; int oldValueA = 0; int oldValueB = 0; unsigned long startMillis; unsigned long currentMillis; bool stateA = false; bool stateB = false; float lastTemp; float lastHum; uint8_t nNoUpdatesTemp; uint8_t nNoUpdatesHum; bool metric = true; static const uint8_t FORCE_UPDATE_N_READS = 10;//10 static const uint64_t UPDATE_INTERVAL = 30000;//30000 Bounce debouncerA = Bounce(); Bounce debouncerB = Bounce(); MyMessage msgA(SSR_A_ID, V_STATUS); MyMessage msgB(SSR_B_ID, V_STATUS); MyMessage msgHum(CHILD_ID_HUM, V_HUM); MyMessage msgTemp(CHILD_ID_TEMP, V_TEMP); DHT12 dht12; void setup() { //Serial.begin(115200); Wire.begin(); pinMode(buttonPinA, INPUT_PULLUP); // Setup the button Activate internal pull-up pinMode(buttonPinB, INPUT_PULLUP); // Setup the button Activate internal pull-up // After setting up the buttons, setup debouncer debouncerA.attach(buttonPinA); debouncerA.interval(5); //5 debouncerB.attach(buttonPinB); debouncerB.interval(5);//5 // Make sure relays are off when starting up digitalWrite(relayPinA, RELAY_ON); digitalWrite(relayPinB, RELAY_ON); // Then set relay pins in output mode pinMode(relayPinA, OUTPUT); pinMode(relayPinB, OUTPUT); } void presentation() { // Send the sketch version information to the gateway and Controller sendSketchInfo("2xRelais,2xButton,DHT12", "1.2a"); // Register all sensors to gw (they will be created as child devices) present(SSR_A_ID, S_LIGHT); present(SSR_B_ID, S_LIGHT); present(CHILD_ID_HUM, S_HUM); present(CHILD_ID_TEMP, S_TEMP); metric = getControllerConfig().isMetric; } /* Example on how to asynchronously check for new messages from gw */ void loop() { currentMillis = millis(); debouncerA.update(); // Get the update value int valueA = debouncerA.read(); if (valueA != oldValueA) { send(msgA.set(stateA ? false : true), true); // Send new state and request ack back oldValueA = valueA; } debouncerB.update(); // Get the update value int valueB = debouncerB.read(); if (valueB != oldValueB) { send(msgB.set(stateB ? false : true), true); // Send new state and request ack back oldValueB = valueB; } // Force reading sensor, so it works also after sleep() // dht12.readSensor(true); if (currentMillis - startMillis >= UPDATE_INTERVAL) { // Get temperature from DHT library float temperature = dht12.readTemperature(); if (isnan(temperature)) { Serial.println("Failed reading temperature from DHT!"); } else if (temperature != lastTemp || nNoUpdatesTemp == FORCE_UPDATE_N_READS) { // Only send temperature if it changed since the last measurement or if we didn't send an update for n times lastTemp = temperature; // apply the offset before converting to something different than Celsius degrees temperature += SENSOR_TEMP_OFFSET; if (!metric) { temperature = dht12.readTemperature(); } // Reset no updates counter nNoUpdatesTemp = 0; send(msgTemp.set(temperature, 1)); #ifdef MY_DEBUG Serial.print("T: "); Serial.println(temperature); #endif } else { // Increase no update counter if the temperature stayed the same nNoUpdatesTemp++; } // Get humidity from DHT library float humidity = dht12.readHumidity(); if (isnan(humidity)) { Serial.println("Failed reading humidity from DHT"); } else if (humidity != lastHum || nNoUpdatesHum == FORCE_UPDATE_N_READS) { // Only send humidity if it changed since the last measurement or if we didn't send an update for n times lastHum = humidity; // Reset no updates counter nNoUpdatesHum = 0; send(msgHum.set(humidity, 1)); #ifdef MY_DEBUG Serial.print("H: "); Serial.println(humidity); #endif } else { // Increase no update counter if the humidity stayed the same nNoUpdatesHum++; } startMillis = currentMillis; Serial.print("Startzeit: "); Serial.println(startMillis); } // Sleep for a while to save energy //wait(UPDATE_INTERVAL); } void receive(const MyMessage &message) { // We only expect one type of message from controller. But we better check anyway. if (message.type == V_STATUS) { switch (message.sensor) { case 1: stateA = message.getBool(); digitalWrite(message.sensor + 4, stateA ? RELAY_ON : RELAY_OFF); break; case 2: stateB = message.getBool(); digitalWrite(message.sensor + 4, stateB ? RELAY_ON : RELAY_OFF); break; } // Write some debug info Serial.print("Incoming change for sensor:"); Serial.println(message.sensor); //Serial.print("from node:"); //Serial.println(message.sender); //Serial.print(", New status: "); Serial.println(message.getBool()); } }
-
RE: DHT12 + 2Relay + 2Button - Combining Problem
well i can't find anything strange in the node debug messages..
90243 TSF:MSG:READ,0-0-101,s=2,c=1,t=2,pt=0,l=1,sg=0:1 08:25:35.541 -> Incoming change for sensor:2 08:25:35.541 -> 1 92163 TSF:MSG:READ,0-0-101,s=1,c=1,t=2,pt=0,l=1,sg=0:0 08:25:37.478 -> Incoming change for sensor:1 08:25:37.478 -> 0 92843 TSF:MSG:READ,0-0-101,s=2,c=1,t=2,pt=0,l=1,sg=0:0 08:25:38.139 -> Incoming change for sensor:2 08:25:38.139 -> 0 93059 TSF:MSG:READ,0-0-101,s=2,c=1,t=2,pt=0,l=1,sg=0:1 08:25:38.375 -> Incoming change for sensor:2 08:25:38.375 -> 1 93458 TSF:MSG:READ,0-0-101,s=2,c=1,t=2,pt=0,l=1,sg=0:0 08:25:38.769 -> Incoming change for sensor:2 08:25:38.769 -> 0 93674 TSF:MSG:READ,0-0-101,s=2,c=1,t=2,pt=0,l=1,sg=0:1 08:25:38.963 -> Incoming change for sensor:2 08:25:39.003 -> 1 94012 TSF:MSG:READ,0-0-101,s=2,c=1,t=2,pt=0,l=1,sg=0:0 08:25:39.315 -> Incoming change for sensor:2 08:25:39.315 -> 0 94229 TSF:MSG:READ,0-0-101,s=2,c=1,t=2,pt=0,l=1,sg=0:1 08:25:39.524 -> Incoming change for sensor:2 08:25:39.557 -> 1 94721 TSF:MSG:READ,0-0-101,s=2,c=1,t=2,pt=0,l=1,sg=0:0 08:25:40.049 -> Incoming change for sensor:2 08:25:40.049 -> 0 94893 TSF:MSG:SEND,101-101-0-0,s=0,c=1,t=0,pt=7,l=5,sg=0,ft=0,st=OK:23.5 08:25:40.213 -> T: 23.50 94973 TSF:MSG:SEND,101-101-0-0,s=0,c=1,t=1,pt=7,l=5,sg=0,ft=0,st=OK:40.6 08:25:40.284 -> H: 40.60 08:25:40.284 -> 94981 TSF:MSG:READ,0-0-101,s=2,c=1,t=2,pt=0,l=1,sg=0:1 08:25:40.284 -> Incoming change for sensor:2 08:25:40.284 -> 1 95182 TSF:MSG:READ,0-0-101,s=2,c=1,t=2,pt=0,l=1,sg=0:0 08:25:40.495 -> Incoming change for sensor:2 08:25:40.495 -> 0 95396 TSF:MSG:READ,0-0-101,s=2,c=1,t=2,pt=0,l=1,sg=0:1 08:25:40.702 -> Incoming change for sensor:2 08:25:40.702 -> 1 97184 TSF:MSG:READ,0-0-101,s=1,c=1,t=2,pt=0,l=1,sg=0:1 08:25:42.505 -> Incoming change for sensor:1 08:25:42.505 -> 1 97225 TSF:MSG:READ,0-0-101,s=1,c=1,t=2,pt=0,l=1,sg=0:1 08:25:42.547 -> Incoming change for sensor:1 08:25:42.547 -> 1
and the acting time delay is only sometimes... i don't know jet how to reproduce it....
ok with the time delay i can live... but the buttons would be great if the could work...
any ideas left? -
RE: DHT12 + 2Relay + 2Button - Combining Problem
@mfalkvidd you are right, but in the original Relay-Scetch there is also no digitalWrite command in the loop-section... https://www.mysensors.org/build/relay
only in the viod receive message section is a digitalwrite command....there is also another problmem...sometimes (i can't say when) there is the RElay not working or works lately.. .maybe it is when the DHT is measuring? i can't explain...
-
RE: DHT12 + 2Relay + 2Button - Combining Problem
Hello again.. long time ago.. but still here.
well thankss for the tip... but now i have a other problem...
the relay will work when the order comes from the gateway ... but when i press the button, nothing happens...
any ideas?here the code:
#define MY_DEBUG #define MY_RADIO_NRF24 #define MY_REPEATER_FEATURE // Define Node ID #define MY_NODE_ID 101 #define RELAY_ON 1 #define RELAY_OFF 0 #define SSR_A_ID 1 // Id of the sensor child #define SSR_B_ID 2 // Id of the sensor child #define CHILD_ID_HUM 0 #define CHILD_ID_TEMP 0//1 #define SENSOR_TEMP_OFFSET 0 #include <SPI.h> #include <MySensors.h> #include <Bounce2.h> #include <DHT12.h> #include <Wire.h> const int buttonPinA = 7; const int buttonPinB = 8; const int relayPinA = 6; const int relayPinB = 5; int oldValueA = 0; int oldValueB = 0; bool stateA = false; bool stateB = false; float lastTemp; float lastHum; uint8_t nNoUpdatesTemp; uint8_t nNoUpdatesHum; bool metric = true; static const uint8_t FORCE_UPDATE_N_READS = 10;//10 static const uint64_t UPDATE_INTERVAL = 30000;//30000 Bounce debouncerA = Bounce(); Bounce debouncerB = Bounce(); MyMessage msgA(SSR_A_ID, V_STATUS); MyMessage msgB(SSR_B_ID, V_STATUS); MyMessage msgHum(CHILD_ID_HUM, V_HUM); MyMessage msgTemp(CHILD_ID_TEMP, V_TEMP); DHT12 dht12; void setup() { //Serial.begin(115200); Wire.begin(); pinMode(buttonPinA, INPUT_PULLUP); // Setup the button Activate internal pull-up pinMode(buttonPinB, INPUT_PULLUP); // Setup the button Activate internal pull-up // After setting up the buttons, setup debouncer debouncerA.attach(buttonPinA); debouncerA.interval(5); //5 debouncerB.attach(buttonPinB); debouncerB.interval(5);//5 // Make sure relays are off when starting up digitalWrite(relayPinA, RELAY_OFF); digitalWrite(relayPinB, RELAY_OFF); // Then set relay pins in output mode pinMode(relayPinA, OUTPUT); pinMode(relayPinB, OUTPUT); } void presentation() { // Send the sketch version information to the gateway and Controller sendSketchInfo("2xRelais,2xButton,DHT12", "1.2"); // Register all sensors to gw (they will be created as child devices) present(SSR_A_ID, S_LIGHT); present(SSR_B_ID, S_LIGHT); present(CHILD_ID_HUM, S_HUM); present(CHILD_ID_TEMP, S_TEMP); metric = getControllerConfig().isMetric; } /* Example on how to asynchronously check for new messages from gw */ void loop() { debouncerA.update(); // Get the update value int valueA = debouncerA.read(); if (valueA != oldValueA) { send(msgA.set(stateA ? false : true), true); // Send new state and request ack back oldValueA = valueA; } debouncerB.update(); // Get the update value int valueB = debouncerB.read(); if (valueB != oldValueB) { send(msgB.set(stateB ? false : true), true); // Send new state and request ack back oldValueB = valueB; } // Force reading sensor, so it works also after sleep() // dht12.readSensor(true); // Get temperature from DHT library float temperature = dht12.readTemperature(); if (isnan(temperature)) { Serial.println("Failed reading temperature from DHT!"); } else if (temperature != lastTemp || nNoUpdatesTemp == FORCE_UPDATE_N_READS) { // Only send temperature if it changed since the last measurement or if we didn't send an update for n times lastTemp = temperature; // apply the offset before converting to something different than Celsius degrees temperature += SENSOR_TEMP_OFFSET; if (!metric) { temperature = dht12.readTemperature(); } // Reset no updates counter nNoUpdatesTemp = 0; send(msgTemp.set(temperature, 1)); #ifdef MY_DEBUG Serial.print("T: "); Serial.println(temperature); #endif } else { // Increase no update counter if the temperature stayed the same nNoUpdatesTemp++; } // Get humidity from DHT library float humidity = dht12.readHumidity(); if (isnan(humidity)) { Serial.println("Failed reading humidity from DHT"); } else if (humidity != lastHum || nNoUpdatesHum == FORCE_UPDATE_N_READS) { // Only send humidity if it changed since the last measurement or if we didn't send an update for n times lastHum = humidity; // Reset no updates counter nNoUpdatesHum = 0; send(msgHum.set(humidity, 1)); #ifdef MY_DEBUG Serial.print("H: "); Serial.println(humidity); #endif } else { // Increase no update counter if the humidity stayed the same nNoUpdatesHum++; } // Sleep for a while to save energy wait(UPDATE_INTERVAL); } void receive(const MyMessage &message) { // We only expect one type of message from controller. But we better check anyway. if (message.type == V_STATUS) { switch (message.sensor) { case 1: stateA = message.getBool(); digitalWrite(message.sensor + 4, stateA ? RELAY_ON : RELAY_OFF); break; case 2: stateB = message.getBool(); digitalWrite(message.sensor + 4, stateB ? RELAY_ON : RELAY_OFF); break; } // Write some debug info Serial.print("Incoming change for sensor:"); Serial.println(message.sensor); //Serial.print("from node:"); //Serial.println(message.sender); //Serial.print(", New status: "); Serial.println(message.getBool()); } }
-
RE: Mysensort mit DHT12 (i2C)
Well i did it... i've got a working MYSENSOR with DHT12...
here the Code ... its not nice but it works...// Enable debug prints #define MY_DEBUG // Define Node ID #define MY_NODE_ID 128 //#define MY_REPEATER_FEATURE // Enable and select radio type attached #define MY_RADIO_NRF24 #include <SPI.h> #include <MySensors.h> #include <DHT12.h> #include <Wire.h> DHT12 dht12; #define SENSOR_TEMP_OFFSET 0 // Sleep time between sensor updates (in milliseconds) // Must be >1000ms for DHT22 and >2000ms for DHT11 static const uint64_t UPDATE_INTERVAL = 30000; static const uint8_t FORCE_UPDATE_N_READS = 10; #define CHILD_ID_HUM 0 #define CHILD_ID_TEMP 1 float lastTemp; float lastHum; uint8_t nNoUpdatesTemp; uint8_t nNoUpdatesHum; bool metric = true; MyMessage msgHum(CHILD_ID_HUM, V_HUM); MyMessage msgTemp(CHILD_ID_TEMP, V_TEMP); void presentation() { // Send the sketch version information to the gateway sendSketchInfo("DHT12", "1.1"); // Register all sensors to gw (they will be created as child devices) present(CHILD_ID_HUM, S_HUM); present(CHILD_ID_TEMP, S_TEMP); //metric = getControllerConfig().isMetric; } void setup() { Wire.begin(); } void loop() { // Force reading sensor, so it works also after sleep() //dht12.readSensor(true); // Get temperature from DHT library float temperature = dht12.readTemperature(); if (isnan(temperature)) { Serial.println("Failed reading temperature from DHT!"); } else if (temperature != lastTemp || nNoUpdatesTemp == FORCE_UPDATE_N_READS) { // Only send temperature if it changed since the last measurement or if we didn't send an update for n times lastTemp = temperature; // apply the offset before converting to something different than Celsius degrees temperature += SENSOR_TEMP_OFFSET; if (!metric) { temperature = dht12.readTemperature(); } // Reset no updates counter nNoUpdatesTemp = 0; send(msgTemp.set(temperature, 1)); #ifdef MY_DEBUG Serial.print("T: "); Serial.println(temperature); #endif } else { // Increase no update counter if the temperature stayed the same nNoUpdatesTemp++; } // Get humidity from DHT library float humidity = dht12.readHumidity(); if (isnan(humidity)) { Serial.println("Failed reading humidity from DHT"); } else if (humidity != lastHum || nNoUpdatesHum == FORCE_UPDATE_N_READS) { // Only send humidity if it changed since the last measurement or if we didn't send an update for n times lastHum = humidity; // Reset no updates counter nNoUpdatesHum = 0; send(msgHum.set(humidity, 1)); #ifdef MY_DEBUG Serial.print("H: "); Serial.println(humidity); #endif } else { // Increase no update counter if the humidity stayed the same nNoUpdatesHum++; } // Sleep for a while to save energy sleep(UPDATE_INTERVAL); //wait(UPDATE_INTERVAL); //for repeaters }
i Used this library: https://github.com/Bobadas/DHT12_library_Arduino/blob/master/README.md
here the datasheet of the dht12: http://www.robototehnika.ru/file/DHT12.pdf
-
RE: DHT12 + 2Relay + 2Button - Combining Problem
@rejoe2
thanks for the link.. i will try... and report if it worked.. -
DHT12 + 2Relay + 2Button - Combining Problem
Hello
I tried to combine a DHT12 + 2 Relay incl. Buttons.

Well it works (somtimes longer.. sometimes not..), i combined the Scetches of both but often i have the problme, that the Relays don't act anymore and the buttons didn't either since the beginning...i found something on google that i have to change the sleep to wait command so it works little better but after some time it also even quits working.
what is my mistake???
#define MY_DEBUG #define MY_RADIO_NRF24 #define MY_REPEATER_FEATURE #define RELAY_ON 1 #define RELAY_OFF 0 #define SSR_A_ID 1 // Id of the sensor child #define SSR_B_ID 2 // Id of the sensor child #define CHILD_ID_HUM 0 #define CHILD_ID_TEMP 0//1 #define SENSOR_TEMP_OFFSET 0 #include <SPI.h> #include <MySensors.h> #include <Bounce2.h> #include <DHT12.h> #include <Wire.h> const int buttonPinA = 7; const int buttonPinB = 8; const int relayPinA = 6; const int relayPinB = 5; int oldValueA = 0; int oldValueB = 0; bool stateA = false; bool stateB = false; float lastTemp; float lastHum; uint8_t nNoUpdatesTemp; uint8_t nNoUpdatesHum; bool metric = true; static const uint8_t FORCE_UPDATE_N_READS = 10;//10 static const uint64_t UPDATE_INTERVAL = 30000;//30000 Bounce debouncerA = Bounce(); Bounce debouncerB = Bounce(); MyMessage msgA(SSR_A_ID, V_STATUS); MyMessage msgB(SSR_B_ID, V_STATUS); MyMessage msgHum(CHILD_ID_HUM, V_HUM); MyMessage msgTemp(CHILD_ID_TEMP, V_TEMP); DHT12 dht12; void setup() { //Serial.begin(115200); Wire.begin(); pinMode(buttonPinA, INPUT_PULLUP); // Setup the button Activate internal pull-up pinMode(buttonPinB, INPUT_PULLUP); // Setup the button Activate internal pull-up // After setting up the buttons, setup debouncer debouncerA.attach(buttonPinA); debouncerA.interval(5); //5 debouncerB.attach(buttonPinB); debouncerB.interval(5);//5 // Make sure relays are off when starting up digitalWrite(relayPinA, RELAY_OFF); digitalWrite(relayPinB, RELAY_OFF); // Then set relay pins in output mode pinMode(relayPinA, OUTPUT); pinMode(relayPinB, OUTPUT); } void presentation() { // Send the sketch version information to the gateway and Controller sendSketchInfo("2xRelais,2xButton,DHT12", "1.2"); // Register all sensors to gw (they will be created as child devices) present(SSR_A_ID, S_LIGHT); present(SSR_B_ID, S_LIGHT); present(CHILD_ID_HUM, S_HUM); present(CHILD_ID_TEMP, S_TEMP); metric = getControllerConfig().isMetric; } /* Example on how to asynchronously check for new messages from gw */ void loop() { debouncerA.update(); // Get the update value int valueA = debouncerA.read(); if (valueA != oldValueA) { send(msgA.set(stateA ? false : true), true); // Send new state and request ack back oldValueA = valueA; } debouncerB.update(); // Get the update value int valueB = debouncerB.read(); if (valueB != oldValueB) { send(msgB.set(stateB ? false : true), true); // Send new state and request ack back oldValueB = valueB; } // Force reading sensor, so it works also after sleep() // dht12.readSensor(true); // Get temperature from DHT library float temperature = dht12.readTemperature(); if (isnan(temperature)) { Serial.println("Failed reading temperature from DHT!"); } else if (temperature != lastTemp || nNoUpdatesTemp == FORCE_UPDATE_N_READS) { // Only send temperature if it changed since the last measurement or if we didn't send an update for n times lastTemp = temperature; // apply the offset before converting to something different than Celsius degrees temperature += SENSOR_TEMP_OFFSET; if (!metric) { temperature = dht12.readTemperature(); } // Reset no updates counter nNoUpdatesTemp = 0; send(msgTemp.set(temperature, 1)); #ifdef MY_DEBUG Serial.print("T: "); Serial.println(temperature); #endif } else { // Increase no update counter if the temperature stayed the same nNoUpdatesTemp++; } // Get humidity from DHT library float humidity = dht12.readHumidity(); if (isnan(humidity)) { Serial.println("Failed reading humidity from DHT"); } else if (humidity != lastHum || nNoUpdatesHum == FORCE_UPDATE_N_READS) { // Only send humidity if it changed since the last measurement or if we didn't send an update for n times lastHum = humidity; // Reset no updates counter nNoUpdatesHum = 0; send(msgHum.set(humidity, 1)); #ifdef MY_DEBUG Serial.print("H: "); Serial.println(humidity); #endif } else { // Increase no update counter if the humidity stayed the same nNoUpdatesHum++; } // Sleep for a while to save energy wait(UPDATE_INTERVAL); } void receive(const MyMessage &message) { // We only expect one type of message from controller. But we better check anyway. if (message.type == V_STATUS) { switch (message.sensor) { case 1: stateA = message.getBool(); digitalWrite(message.sensor + 4, stateA ? RELAY_ON : RELAY_OFF); break; case 2: stateB = message.getBool(); digitalWrite(message.sensor + 4, stateB ? RELAY_ON : RELAY_OFF); break; } // Write some debug info Serial.print("Incoming change for sensor:"); Serial.println(message.sensor); //Serial.print("from node:"); //Serial.println(message.sender); //Serial.print(", New status: "); Serial.println(message.getBool()); } }
Thanks for help!
-
RE: Mini Pro + DHT12 + NRF24L01+ SMD
@gohan thanks for the info.... you were right!
i soldered the smd radio like in the building instruktions descripted,and because of that, that there were no infos about the voltage of the smd radio i used the 3,3v regulator ... that was the mistake!!!
so i thaught, if it's not workimg, let's try tu use the 5v, worst case the radio will go to hell....
so... testet with 5v on themradio and ... heureka it works.... now i have the dht12 with nrf24 smd on a mini pro.... so the sensor is very small and easy coded....
i will make a build and wire diagramm and rewrite the code... who can i send it for publication on the mysensors site? -
RE: Mini Pro + DHT12 + NRF24L01+ SMD
@mfalkvidd Thanks for the link... got it..
here the output from the debug..
pi@SmartPi:~/MySensors $ sudo ./bin/mysgw -d mysgw: Starting gateway... mysgw: Protocol version - 2.1.0-beta mysgw: MCO:BGN:INIT GW,CP=RNNG--Q,VER=2.1.0-beta mysgw: TSF:LRT:OK mysgw: TSM:INIT mysgw: TSF:WUR:MS=0 mysgw: !TSM:INIT:TSP FAIL mysgw: TSM:FAIL:CNT=1 mysgw: TSM:FAIL:PDT mysgw: TSM:FAIL:RE-INIT mysgw: TSM:INIT mysgw: !TSM:INIT:TSP FAIL mysgw: TSM:FAIL:CNT=2 mysgw: TSM:FAIL:PDT mysgw: TSM:FAIL:RE-INIT mysgw: TSM:INIT mysgw: !TSM:INIT:TSP FAIL mysgw: TSM:FAIL:CNT=3 mysgw: TSM:FAIL:PDT mysgw: TSM:FAIL:RE-INIT mysgw: TSM:INIT mysgw: !TSM:INIT:TSP FAIL mysgw: TSM:FAIL:CNT=4 mysgw: TSM:FAIL:PDT mysgw: TSM:FAIL:RE-INIT mysgw: TSM:INIT mysgw: !TSM:INIT:TSP FAIL mysgw: TSM:FAIL:CNT=5 mysgw: TSM:FAIL:PDT mysgw: TSM:FAIL:RE-INIT mysgw: TSM:INIT mysgw: !TSM:INIT:TSP FAIL mysgw: TSM:FAIL:CNT=6 mysgw: TSM:FAIL:PDT mysgw: TSM:FAIL:RE-INIT mysgw: TSM:INIT mysgw: !TSM:INIT:TSP FAIL mysgw: TSM:FAIL:CNT=7 mysgw: TSM:FAIL:PDT mysgw: TSM:FAIL:RE-INIT mysgw: TSM:INIT mysgw: TSM:INIT:TSP OK mysgw: TSM:INIT:GW MODE mysgw: TSM:READY:ID=0,PAR=0,DIS=0 mysgw: MCO:BGN:STP mysgw: MCO:BGN:INIT OK,TSP=1 mysgw: TSF:MSG:READ,70-70-255,s=255,c=3,t=7,pt=0,l=0,sg=0: mysgw: TSF:MSG:BC mysgw: TSF:MSG:FPAR REQ,ID=70 mysgw: TSF:PNG:SEND,TO=0 mysgw: TSF:CKU:OK mysgw: TSF:MSG:GWL OK mysgw: Received SIGINT
My other sensors are working fine, just the new one isn't .
-
RE: Mini Pro + DHT12 + NRF24L01+ SMD
@mfalkvidd sure i can, but how? is this you want to see an extra log direct from the gateway? where will i find this log?
as i said i'm not an it technican just an hobbyist, a little DIY'er....