Hello Everyone
Recently I'm fighting with LED dimmer (rookie in programming)
I got to the point where (with help of @Boots33 - Thank You) I have two different sketches both work OK with saving the last dim status but..... only for 1 LED stripe
Tried to implement an array as declaration of additional LED pins (there is a topic on the forum https://forum.mysensors.org/topic/4808/dimmable-led-actuator/6 ) but using this method... I finished with..
1st sketch switching on/off crazy (one button in Domoticz switching a group of lights , some of them not working at all etc.. - >in summarry a mess !)
2nd I didnt even get to the point of few leds - because there is already an array which is a little confusing for me
Q1: Could somebody please have a look on both and advice which one is potentially (better written , less giving problems with multiple pins, I should focus on ?) and help me with declaration of extra pins ?? ( basically I'm trying to use all the PWM pins from Arduino Mega)
Q2: There is already an array in 2nd sketch for the dimmer but I am not sure how it works and why somebody went this way (definition of maxdimlevels and dimlevels array) ??? is it possible to get rid of it ?
Configuration - RPi, Domoticz, Uno Gateway + nrF24, Sensor node nrf24 connected to Mega , mosfets in breadboard (not soldered at all - so no damage) , external 12V for Mosfet Ledstripes -->7,5V Arduino , for testing purpose 6 led stripes connected to PWM pins
(at present disconnected from GND all except 1st one - stripes are very bright and going crazy when not declared in Arduino)
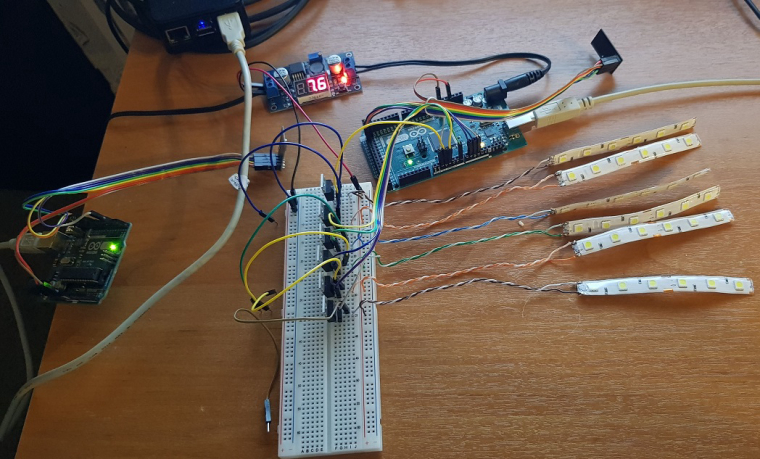
1st sketch
// Enable debug prints to serial monitor
#define MY_DEBUG
// Enable and select radio type attached
#define MY_RADIO_NRF24
//#define MY_RADIO_RFM69
#define MY_RF24_CE_PIN 49
#define MY_RF24_CS_PIN 53
#include <MySensors.h>
#define SN "Dimmable_LED"
#define SV "1.1"
#define LED_PIN 3 // Arduino pin attached to MOSFET Gate pin
#define FADE_DELAY 10 // Delay in ms for each percentage fade up/down (10ms = 1s full-range dim)
static int16_t currentLevel = 0; // Current dim level...
int dimmerSetting = 10 ;
MyMessage dimmerMsg(0, V_PERCENTAGE);
MyMessage lightMsg(0, V_STATUS);
/***
* Dimmable LED initialization method
*/
void setup()
{
// Pull the gateway's current dim level - restore light level upon sendor node power-up
//request( 0, V_PERCENTAGE );
}
void presentation()
{
// Register the LED Dimmable Light with the gateway
present( 0, S_DIMMER );
sendSketchInfo(SN, SV);
}
/***
* Dimmable LED main processing loop
*/
void loop()
{
}
void receive(const MyMessage &message) {
switch (message.type) {
case V_STATUS: // message is from the switch
if(message.getBool()){
fadeToLevel( dimmerSetting ); // turn light on at current dimmer level
}
else fadeToLevel( 0 ); // fade light to 0 (off)
break;
case V_PERCENTAGE: // message is from the dimmer
dimmerSetting = message.getInt(); // get the new dimmer setting from the message
fadeToLevel( dimmerSetting ); // fade to the new dimmer setting
send(dimmerMsg.set(dimmerSetting)); // send switch state to controller , no ack requested
break;
}
}
/***
* This method provides a graceful fade up/down effect
*/
void fadeToLevel( int toLevel )
{
int delta = ( toLevel - currentLevel ) < 0 ? -1 : 1;
while ( currentLevel != toLevel ) {
currentLevel += delta;
analogWrite( LED_PIN, (int)(currentLevel / 100. * 255) );
wait( FADE_DELAY );
}
}
2nd sketch based on MySensors generic dimmablelight (modified by me a little using gesture sensor sketch)
/**
* The MySensors Arduino library handles the wireless radio link and protocol
* between your home built sensors/actuators and HA controller of choice.
* The sensors forms a self healing radio network with optional repeaters. Each
* repeater and gateway builds a routing tables in EEPROM which keeps track of the
* network topology allowing messages to be routed to nodes.
*
* Created by Henrik Ekblad <henrik.ekblad@mysensors.org>
* Copyright (C) 2013-2015 Sensnology AB
* Full contributor list: https://github.com/mysensors/Arduino/graphs/contributors
*
* Documentation: http://www.mysensors.org
* Support Forum: http://forum.mysensors.org
*
* This program is free software; you can redistribute it and/or
* modify it under the terms of the GNU General Public License
* version 2 as published by the Free Software Foundation.
*
*******************************
*
* REVISION HISTORY
* Version 1.0 - January 30, 2015 - Developed by GizMoCuz (Domoticz)
*
* DESCRIPTION
* This sketch provides an example how to implement a Dimmable Light
* It is pure virtual and it logs messages to the serial output
* It can be used as a base sketch for actual hardware.
* Stores the last light state and level in eeprom.
*
*/
// Enable debug prints
#define MY_DEBUG
// Enable and select radio type attached
#define MY_RADIO_NRF24
//#define MY_RADIO_RFM69
#define MY_RF24_CE_PIN 49
#define MY_RF24_CS_PIN 53
#include <MySensors.h>
#define CHILD_ID_LIGHT 1
#define EPROM_LIGHT_STATE 1
#define EPROM_DIMMER_LEVEL 2
#define LIGHT_OFF 0
#define LIGHT_ON 1
#define SN "DimableLightg"
#define SV "1.0"
#define LED_PIN 3 // Arduino pin attached to MOSFET Gate pin
#define MAXDIMLEVELS 100
//#define FADE_DELAY 10 // Delay in ms for each percentage fade up/down (10ms = 1s full-range dim)
int16_t LastLightState=LIGHT_OFF;
int16_t LastDimValue=MAXDIMLEVELS;
int dimlevels[ MAXDIMLEVELS ] = // PWM values used for translating home automation dimmer levels. This gives smoother transations
{ 0, 1, 2, 3, 4, 5, 6, 7, 8, 9,
10, 11, 12, 13, 14, 15, 16, 17, 18, 19,
20, 21, 22, 23, 24, 25, 26, 27, 28, 29,
30, 31, 32, 35, 39, 42, 46, 49, 52, 56,
59, 62, 66, 69, 73, 76, 79, 83, 86, 89,
93, 96, 100, 103, 106, 110, 113, 116, 120, 123,
126, 130, 133, 137, 140, 144, 147, 150, 154, 157,
160, 164, 167, 171, 174, 177, 181, 184, 187, 191,
194, 197, 201, 204, 208, 211, 215, 218, 221, 225,
228, 231, 235, 238, 242, 245, 246, 250, 251, 255 };
MyMessage lightMsg(CHILD_ID_LIGHT, V_LIGHT);
MyMessage dimmerMsg(CHILD_ID_LIGHT, V_DIMMER);
void setup()
{
//Retreive our last light state from the eprom
int LightState=loadState(EPROM_LIGHT_STATE);
if (LightState<=1) {
LastLightState=LightState;
int DimValue=loadState(EPROM_DIMMER_LEVEL);
if ((DimValue>0)&&(DimValue<=100)) {
//There should be no Dim value of 0, this would mean LIGHT_OFF
LastDimValue=DimValue;
}
}
//Here you actualy switch on/off the light with the last known dim level
SetCurrentState2Hardware();
Serial.println( "Node ready to receive messages..." );
}
void presentation()
{
//Send the Sketch Version Information to the Gateway
sendSketchInfo(SN, SV);
present(CHILD_ID_LIGHT, S_DIMMER );
}
void loop()
{
}
void receive(const MyMessage &message)
{
if (message.type == V_LIGHT) {
Serial.println( "V_LIGHT command received..." );
int lstate= atoi( message.data );
if ((lstate<0)||(lstate>1)) {
Serial.println( "V_LIGHT data invalid (should be 0/1)" );
return;
}
LastLightState=lstate;
saveState(EPROM_LIGHT_STATE, LastLightState);
if ((LastLightState==LIGHT_ON)&&(LastDimValue==0)) {
//In the case that the Light State = On, but the dimmer value is zero,
//then something (probably the controller) did something wrong,
//for the Dim value to 100%
LastDimValue=100;
saveState(EPROM_DIMMER_LEVEL, LastDimValue);
}
//When receiving a V_LIGHT command we switch the light between OFF and the last received dimmer value
//This means if you previously set the lights dimmer value to 50%, and turn the light ON
//it will do so at 50%
} else if (message.type == V_DIMMER) {
Serial.println( "V_DIMMER command received..." );
int dimvalue= atoi( message.data );
if ((dimvalue<0)||(dimvalue>100)) {
Serial.println( "V_DIMMER data invalid (should be 0..100)" );
return;
}
if (dimvalue==0) {
LastLightState=LIGHT_OFF;
} else {
LastLightState=LIGHT_ON;
LastDimValue=dimvalue;
saveState(EPROM_DIMMER_LEVEL, LastDimValue);
}
} else {
Serial.println( "Invalid command received..." );
return;
}
//Here you set the actual light state/level
SetCurrentState2Hardware();
}
void SetCurrentState2Hardware()
{
if (LastLightState==LIGHT_OFF) {
analogWrite( LED_PIN, dimlevels[0] );
Serial.println( "Light state: OFF" );
} else {
analogWrite( LED_PIN, dimlevels[ LastDimValue - 1 ] );
Serial.print( "Light state: ON, Level: " );
Serial.println( LastDimValue );
}
//Send current state to the controller
SendCurrentState2Controller();
}
void SendCurrentState2Controller()
{
if ((LastLightState==LIGHT_OFF)||(LastDimValue==0)) {
send(dimmerMsg.set((int16_t)0));
} else {
send(dimmerMsg.set(LastDimValue));
}
}