Hi!
I've try with developpement librairies and it's the same.
For information this is the sketch i'm using:
// Enable debug prints
#define MY_DEBUG
// Enable and select radio type attached
#define MY_RADIO_NRF24
//#define MY_RADIO_RFM69
//#define MY_RS485
#include <SPI.h>
#include <MySensors.h>
#include <DHT.h>
#include <DallasTemperature.h>
#include <OneWire.h>
#define MAX_ATTACHED_DS18B20 6
#define ONE_WIRE_BUS 4
#define DHT1_DATA_PIN 2
#define DHT2_DATA_PIN 3
#define CHILD_ID_DHT1HUM 6
#define CHILD_ID_DHT1TEMP 7
#define CHILD_ID_DHT2HUM 9
#define CHILD_ID_DHT2TEMP 8
#define SENSOR_TEMP_OFFSET 0
// Sleep time between sensor updates (in milliseconds)
// Must be >1000ms for DHT22 and >2000ms for DHT11
static const uint64_t UPDATE_INTERVAL = 3000;
unsigned long SLEEP_TIME = 30000;
bool metric = true;
bool receivedConfig = false;
int numSensors=0;
OneWire oneWire(ONE_WIRE_BUS); // Setup a oneWire instance to communicate with any OneWire devices (not just Maxim/Dallas temperature ICs)
DallasTemperature sensors(&oneWire); // Pass the oneWire reference to Dallas Temperature.
MyMessage msgDallas(0,V_TEMP);
MyMessage msgDHT1Temp(CHILD_ID_DHT1TEMP,V_TEMP);
MyMessage msgDHT1Hum(CHILD_ID_DHT1HUM,V_HUM);
MyMessage msgDHT2Hum(CHILD_ID_DHT2HUM,V_HUM);
MyMessage msgDHT2Temp(CHILD_ID_DHT2TEMP,V_TEMP);
DHT dht;
void before()
{
// Startup up the OneWire library
sensors.begin();
}
void setup()
{
// requestTemperatures() will not block current thread
sensors.setWaitForConversion(false);
}
void presentation()
{
// Send the sketch version information to the gateway
sendSketchInfo("DualDHTandDallas", "1.1");
// Fetch the number of attached temperature sensors
numSensors = sensors.getDeviceCount();
// Present all sensors to controller
for (int i=0; i<numSensors && i<MAX_ATTACHED_DS18B20; i++) {
present(i, S_TEMP);
}
present(CHILD_ID_DHT1TEMP,S_TEMP);
present(CHILD_ID_DHT1HUM,S_HUM);
present(CHILD_ID_DHT2HUM,S_HUM);
present(CHILD_ID_DHT2TEMP,S_TEMP);
metric = getConfig().isMetric;
}
void loop()
{
// Fetch temperatures from Dallas sensors
sensors.requestTemperatures();
// query conversion time and sleep until conversion completed
int16_t conversionTime = sensors.millisToWaitForConversion(sensors.getResolution());
// sleep() call can be replaced by wait() call if node need to process incoming messages (or if node is repeater)
sleep(conversionTime);
// Read temperatures and send them to controller
for (int i=0; i<numSensors && i<MAX_ATTACHED_DS18B20; i++) {
// Fetch and round temperature to one decimal
float temperature = static_cast<float>(static_cast<int>((getConfig().isMetric?sensors.getTempCByIndex(i):sensors.getTempFByIndex(i)) * 10.)) / 10.;
// Send in the new temperature
send(msgDallas.setSensor(i).set(temperature,1));
#ifdef MY_DEBUG
Serial.print("Dallas");
Serial.print(i);
Serial.print(" :");
Serial.println(temperature);
#endif
// sleep(SLEEP_TIME);
}
dht.setup(DHT1_DATA_PIN); // set data pin of DHT1 sensor
if (UPDATE_INTERVAL <= dht.getMinimumSamplingPeriod()) {
Serial.println("Warning: UPDATE_INTERVAL is smaller than supported by the sensor!");
}
// Sleep for the time of the minimum sampling period to give the sensor time to power up
// (otherwise, timeout errors might occure for the first reading)
sleep(dht.getMinimumSamplingPeriod());
// Force reading sensor, so it works also after sleep()
dht.readSensor(true);
// Get temperature from DHT library
float dht1temperature = dht.getTemperature();
if (isnan(dht1temperature)) {
Serial.println("Failed reading temperature from DHT!");
} else
if (!metric) {
dht1temperature = dht.toFahrenheit(dht1temperature);
}
dht1temperature += SENSOR_TEMP_OFFSET;
send(msgDHT1Temp.set(dht1temperature, 1));
#ifdef MY_DEBUG
Serial.print("DHT1TEMP: ");
Serial.println(dht1temperature);
#endif
sleep(UPDATE_INTERVAL);
// Get humidity from DHT library
float dht1humidity = dht.getHumidity();
if (isnan(dht1humidity)) {
Serial.println("Failed reading humidity from DHT");
}
else {
send(msgDHT1Hum.set(dht1humidity, 1));
#ifdef MY_DEBUG
Serial.print("DHT1HUM: ");
Serial.println(dht1humidity);
#endif
}
// Sleep for a while to save energy
sleep(UPDATE_INTERVAL);
dht.setup(DHT2_DATA_PIN); // set data pin of DHT2 sensor
if (UPDATE_INTERVAL <= dht.getMinimumSamplingPeriod()) {
Serial.println("Warning: UPDATE_INTERVAL is smaller than supported by the sensor!");
}
// Sleep for the time of the minimum sampling period to give the sensor time to power up
// (otherwise, timeout errors might occure for the first reading)
sleep(dht.getMinimumSamplingPeriod());
// Force reading sensor, so it works also after sleep()
dht.readSensor(true);
// Get temperature from DHT library
float dht2temperature = dht.getTemperature();
if (isnan(dht2temperature)) {
Serial.println("Failed reading temperature from DHT!");
} else
if (!metric) {
dht2temperature = dht.toFahrenheit(dht2temperature);
}
dht2temperature += SENSOR_TEMP_OFFSET;
send(msgDHT2Temp.set(dht2temperature, 1));
#ifdef MY_DEBUG
Serial.print("DHT2TEMP: ");
Serial.println(dht2temperature);
#endif
sleep(UPDATE_INTERVAL);
// Get humidity from DHT library
float dht2humidity = dht.getHumidity();
if (isnan(dht2humidity)) {
Serial.println("Failed reading humidity from DHT");
} else {
send(msgDHT2Hum.set(dht2humidity, 1));
#ifdef MY_DEBUG
Serial.print("DHT2HUM: ");
Serial.println(dht2humidity);
#endif
}
sleep(UPDATE_INTERVAL);
}
And in domoticz all childs have the same ID (#1)
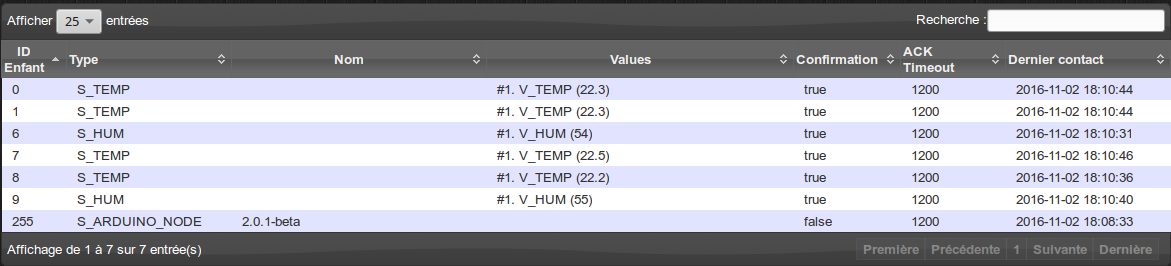
Noboby have an idea of the problem ?
[EDIT]
And i just discover an other "problem": the 2nd DHT22 humidity value is updated with the value of the 1st one...