This is my project!!!!
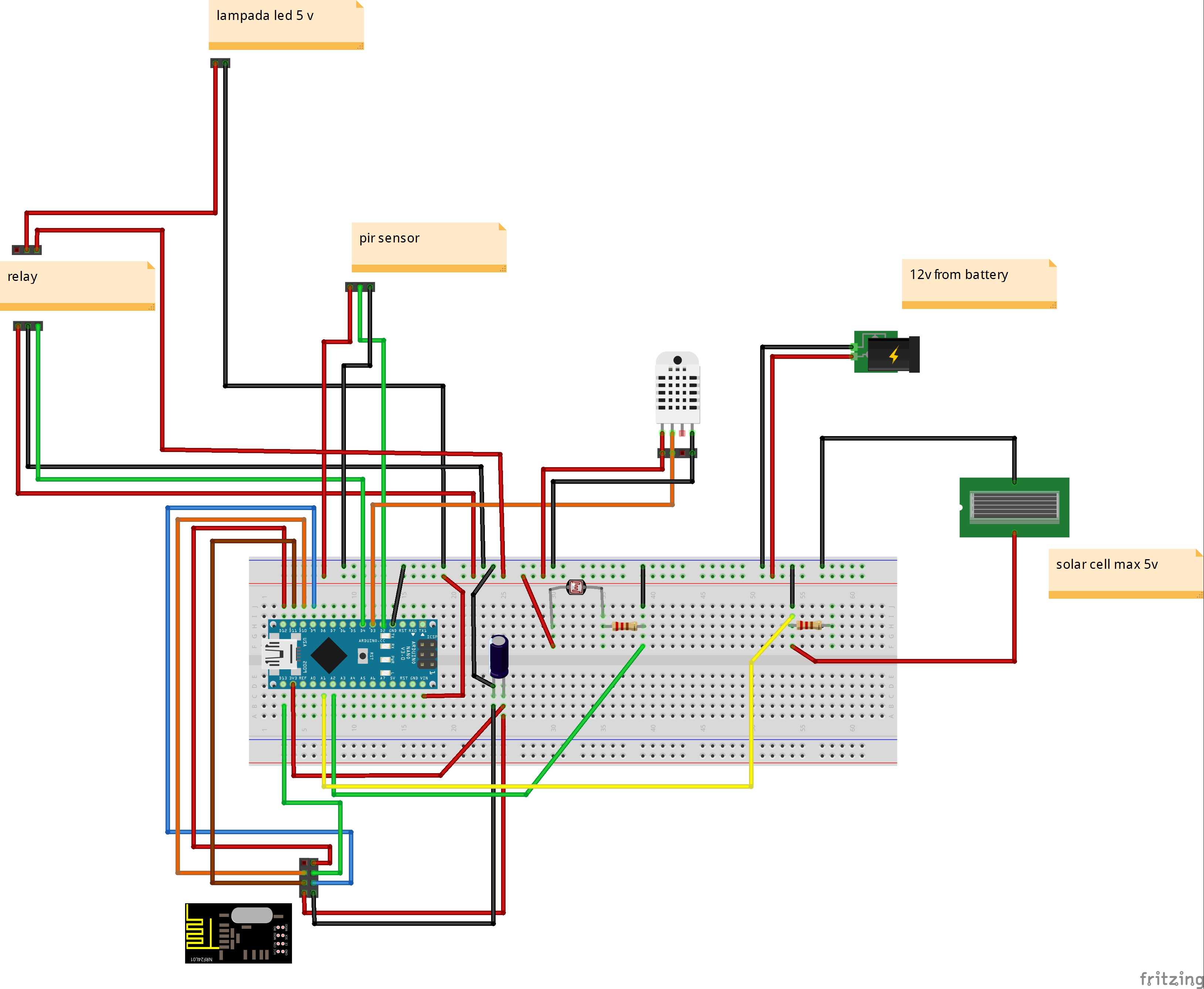
// Example sketch för a "light switch" where you can control light or something
// else from both vera and a local physical button (connected between digital
// pin 3 and GND).
// This node also works as a repeader for other nodes
#include <MySensor.h>
#include <SPI.h>
#include <DHT.h>
unsigned long SLEEP_TIME = 120000; // Sleep time between reports (in milliseconds)
//pin sensori
#define PIR_PIN 2 // The digital input you attached your motion sensor. (Only 2 and 3 generates interrupt!)
#define HUMIDITY_TEMPERATURE_PIN 3 // sensore temperatura umidita
#define RELAY_PIN 4 // relay pin
int BATTERY_SENSE_PIN = A1; // Pin carica batteria o pannello solare
int FOTORESIST_SENSE_PIN = A2; // Pin fotoresistenza
//interupt per sleep arduino
#define INTERRUPT PIR_PIN-2 // Usually the interrupt = pin -2 (on uno/nano anyway)
//id per vera
#define CHILD_ID_RELE 1 // Id relay
#define CHILD_ID_HUM 2 // id temperatura
#define CHILD_ID_TEMP 3 // id umidita
#define CHILD_ID_PIR 4 // Id pir
#define CHILD_ID_LIGHT 5 // Id luminosita (fotoresistenza)
//definizione per nodo vera
#define NODE_ID 10
#define SN "meteo station"
#define SV "1.4"
//variabili
bool state_relay; //stato relay
float batt_valore; //dichiaro la variabile valore che memorizzerà il valore della batteria dal pin analogico
float batt_volt; //volt batteria
float batt_charged_percent; //percentuale carica batteria
float last_batt_charged_percent; //percentuale carica batteria precedente
float batt_min_voltage = 0.5; //tensione minima batteria
float batt_max_voltage = 5; //tensione massima batteria
float fotoresistenza_valore; //dichiaro la variabile valore che memorizzerà il valore della fotoresistenza dal pin analogico
float last_fotoresistenza_valore; //dichiaro la variabile valore precedente
int lux_vera; //valore luminosita da inviare a vera
MySensor gw;
// sensore temperatura umidita
DHT dht_int;
float lastTemp_int = -1;
float lastHum_int = -1;
boolean metric = true;
MyMessage msgRelay(CHILD_ID_RELE,V_LIGHT);
MyMessage msgHum(CHILD_ID_HUM, V_HUM);
MyMessage msgTemp(CHILD_ID_TEMP, V_TEMP);
MyMessage msgPir(CHILD_ID_PIR, V_TRIPPED);
MyMessage msgLux(CHILD_ID_LIGHT, V_LIGHT_LEVEL);
void setup()
{
gw.begin(incomingMessage, NODE_ID, false);
gw.sendSketchInfo(SN, SV);
//Sensore umidita temperatura
dht_int.setup(HUMIDITY_TEMPERATURE_PIN);
gw.present(CHILD_ID_RELE, S_LIGHT); //light vera
gw.present(CHILD_ID_HUM, S_HUM); //umidity vera
gw.present(CHILD_ID_TEMP, S_TEMP); // temp vera
gw.present(CHILD_ID_PIR, S_MOTION); // motion vera
gw.present(CHILD_ID_LIGHT, S_LIGHT_LEVEL); //light level (fotoresistenza)
metric = gw.getConfig().isMetric;
// Then set relay pins in output mode
pinMode(RELAY_PIN, OUTPUT);
//PIR
pinMode(PIR_PIN, INPUT);
state_relay = 0;
//GestisciRelay();
digitalWrite(RELAY_PIN, LOW);
gw.send(msgRelay.set(state_relay));
}
void loop()
{
gw.process();
//sensore temperatura umidita
delay(dht_int.getMinimumSamplingPeriod());
float temperature_int = dht_int.getTemperature();
if (isnan(temperature_int))
{
lastTemp_int = -1;
Serial.println("Failed reading temperature from DHT");
}
else if (temperature_int != lastTemp_int)
{
lastTemp_int = temperature_int;
if (!metric)
{
temperature_int = dht_int.toFahrenheit(temperature_int);
}
gw.send(msgTemp.set(temperature_int, 1));
Serial.print("T int: ");
Serial.println(temperature_int);
}
float humidity_int = dht_int.getHumidity();
if (isnan(humidity_int))
{
lastHum_int = -1;
Serial.println("Failed reading humidity from DHT");
}
else if (humidity_int != lastHum_int)
{
lastHum_int = humidity_int;
gw.send(msgHum.set(humidity_int, 1));
Serial.print("H int: ");
Serial.println(humidity_int);
}
//sensore temperatura umidita
//fotoresistenza
for(int i=0;i<150;i++)
{
fotoresistenza_valore += analogRead(FOTORESIST_SENSE_PIN); //read the input voltage from battery or solar panel
delay(2);
}
fotoresistenza_valore = fotoresistenza_valore / 150;
Serial.print ("fotoresistenza: ");
Serial.println(fotoresistenza_valore);
if (fotoresistenza_valore != last_fotoresistenza_valore)
{
lux_vera = (int) fotoresistenza_valore;
gw.send(msgLux.set(lux_vera));
last_fotoresistenza_valore = fotoresistenza_valore;
}
//fotoresistenza
//pir relay
// Read digital motion value
boolean tripped = digitalRead(PIR_PIN) == HIGH;
Serial.println("pir:");
Serial.println(tripped);
gw.send(msgPir.set(tripped?"1":"0")); // Send tripped value to gw
//accende la luce con il buio
if (fotoresistenza_valore < 200) //poca luce
{
if (tripped == 1)
{
state_relay = 1;
}
else
{
state_relay = 0;
}
}
//accende la luce con il buio
GestisciRelay();
//pir relay
//battery
for(int i=0;i<150;i++)
{
batt_valore += analogRead(BATTERY_SENSE_PIN); //read the input voltage from battery or solar panel
delay(2);
}
batt_valore = batt_valore / 150;
Serial.print ("batt_valore: ");
Serial.println(batt_valore);
batt_volt = (batt_valore / 1024) * batt_max_voltage;
Serial.print ("batt_volt: ");
Serial.println(batt_volt);
////////////////////////////////////////////////
//The map() function uses integer math so will not generate fractions
// so I multiply battery voltage with 10 to convert float into a intiger value
// when battery voltage is 6.0volt it is totally discharged ( 6*10 =60)
// when battery voltage is 7.2volt it is fully charged (7.2*10=72)
// 6.0v =0% and 7.2v =100%
//batt_charged_percent = batt_volt*10;
//batt_charged_percent = map(batt_volt*10, 60 , 72, 0, 100);
batt_charged_percent = batt_volt * 10;
batt_charged_percent = map(batt_volt * 10, batt_min_voltage * 10 , batt_max_voltage * 10, 0, 100);
//batt_charged_percent = (batt_volt / batt_max_voltage) * 100;
Serial.print ("batt_charged_percent: ");
Serial.println(batt_charged_percent);
if (last_batt_charged_percent != batt_charged_percent)
{
gw.sendBatteryLevel(batt_charged_percent);
last_batt_charged_percent = batt_charged_percent;
}
//battery
delay(50);
// Sleep until interrupt comes in on motion sensor. Send update every two minute.
gw.sleep(INTERRUPT, CHANGE, SLEEP_TIME);
}
void incomingMessage(const MyMessage &message) {
// We only expect one type of message from controller. But we better check anyway.
if (message.isAck()) {
Serial.println("This is an ack from gateway");
}
if (message.type == V_LIGHT) {
// Change relay state_relay
state_relay = message.getBool();
GestisciRelay();
// Write some debug info
Serial.print("Incoming change for sensor:");
Serial.print(message.sensor);
Serial.print(", New status: ");
Serial.println(message.getBool());
}
}
void GestisciRelay()
{
//Serial.print(" GestisciRelay state_relay:");
//Serial.println(state_relay);
if (state_relay == 0)
{
digitalWrite(RELAY_PIN, LOW);
gw.send(msgRelay.set(state_relay));
//Serial.println("SPENTO RELAY");
}
else
{
digitalWrite(RELAY_PIN, HIGH);
gw.send(msgRelay.set(state_relay));
//Serial.println("ACCESO RELAY");
}
}
On vera
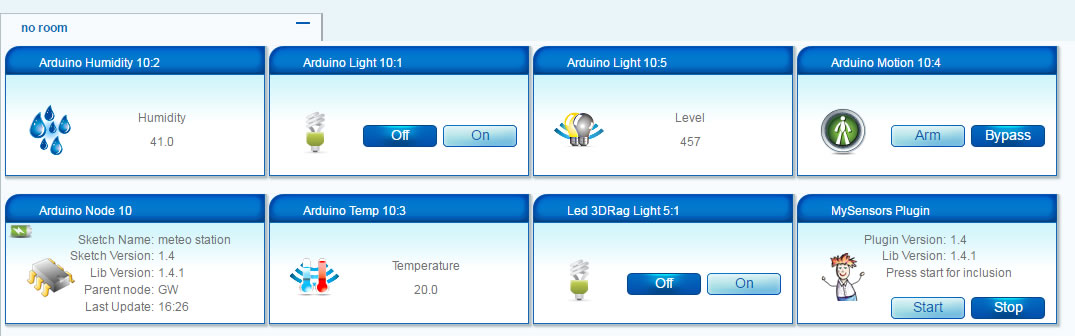
On board
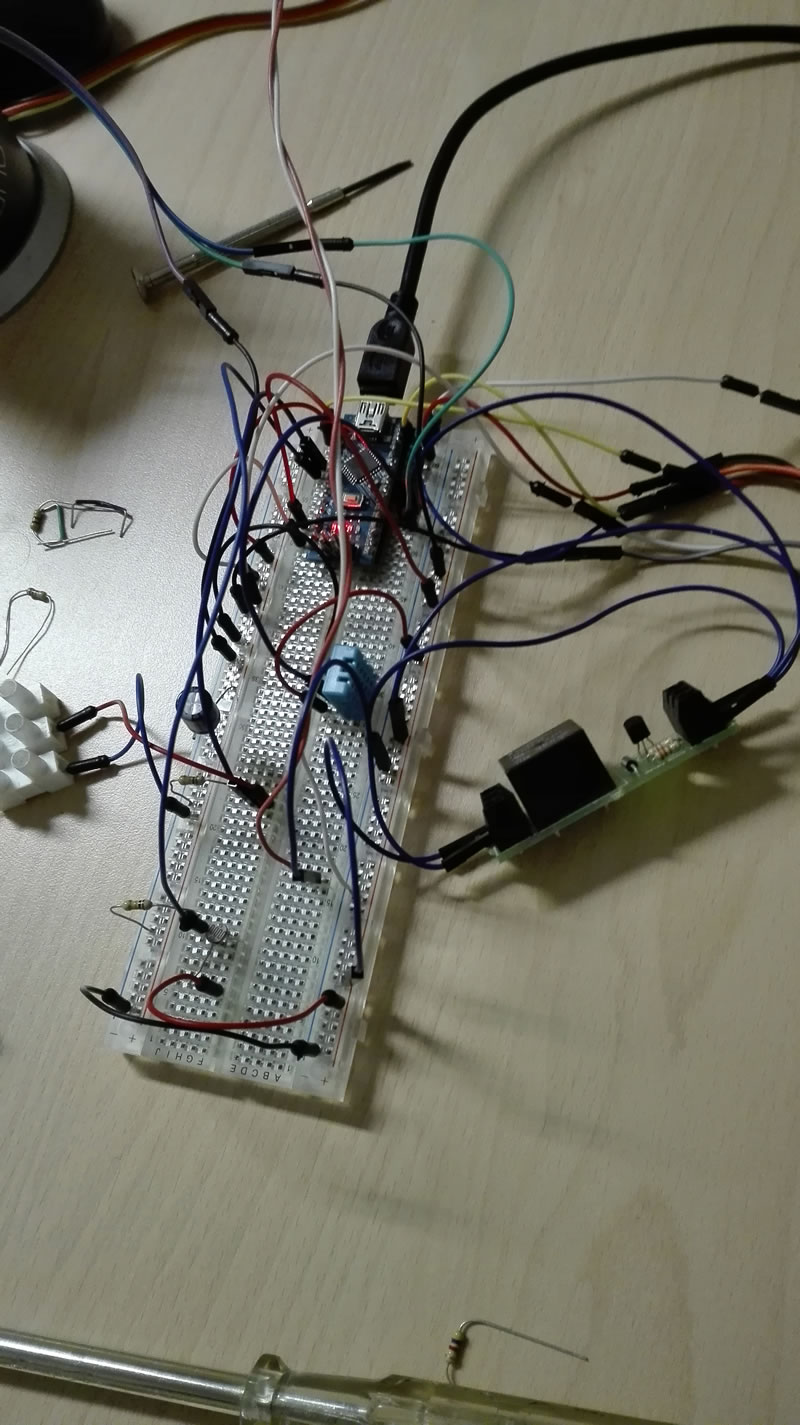