Ok, i have managed to get a heatpump IR sketch working somewhat. I can see the node in 'hardware' and even the children but they do not appear in 'devices'... is there something fundamentally wrong in my sketch?
I am using Domoticz and a RPI controller.
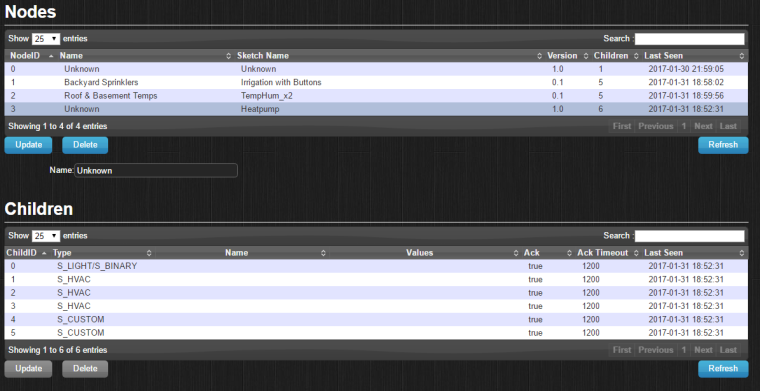
// Enable debug prints to serial monitor
#define MY_DEBUG
// Enable and select radio type attached
#define MY_RADIO_NRF24
#include <SPI.h>
#include <MySensors.h>
#include <MitsubishiHeatpumpIR.h>
#define POWER_ID 0
#define MODE_ID 1
#define FAN_ID 2
#define TEMP_ID 3
#define VDIR_ID 4
#define HDIR_ID 5
MyMessage powerMsg(POWER_ID, V_STATUS);
MyMessage modeMsg(MODE_ID, V_HVAC_FLOW_STATE);
MyMessage fanMsg(FAN_ID, V_PERCENTAGE);
MyMessage tempMsg(TEMP_ID, V_TEMP);
MyMessage vdirMsg(VDIR_ID, V_VAR1);
MyMessage hdirMsg(HDIR_ID, V_VAR2);
IRSenderPWM irSender(3); // IR led on Arduino digital pin 3, using Arduino PWM
HeatpumpIR *heatpumpIR = new MitsubishiFDHeatpumpIR();
//Some global variables to hold the states
int POWER_STATE;
int TEMP_STATE;
int FAN_STATE;
int MODE_STATE;
void setup()
{
// begin(incomingMessage, AUTO, false);
}
void presentation()
{
// Send the sketch version information to the gateway and Controller
sendSketchInfo("Heatpump", "1.0");
// Register all sensors to gw (they will be created as child devices)
present(POWER_ID, S_BINARY);
present(MODE_ID, S_HVAC);
present(FAN_ID, S_HVAC);
present(TEMP_ID, S_HVAC);
present(VDIR_ID, S_CUSTOM);
present(HDIR_ID, S_CUSTOM);
// Load our values on start
POWER_STATE = loadState(POWER_ID);
TEMP_STATE = loadState(TEMP_ID);
FAN_STATE = loadState(FAN_ID);
MODE_STATE = loadState(MODE_ID);
sendHeatpumpCommand();
}
void loop() {
}
void handlePowerMessage(bool newState) {
if (newState) {
POWER_STATE = POWER_ON;
}
else {
POWER_STATE = POWER_OFF;
}
saveState(POWER_ID, newState);
}
void handleModeMessge(int newMode) {
switch(newMode) {
case 0:
MODE_STATE = MODE_HEAT; break;
case 1:
MODE_STATE = MODE_COOL; break;
case 2:
MODE_STATE = MODE_AUTO; break;
case 3:
MODE_STATE = MODE_FAN; break;
case 4:
MODE_STATE = MODE_DRY; break;
}
MODE_STATE = newMode;
saveState(MODE_ID, newMode);
}
void handleFanMessage(int newFan) {
if (newFan > 5) newFan=5;
switch(newFan) {
case 0:
FAN_STATE = FAN_AUTO; break;
case 1:
FAN_STATE = FAN_1; break;
case 2:
FAN_STATE = FAN_2; break;
case 3:
FAN_STATE = FAN_3; break;
case 4:
FAN_STATE = FAN_4; break;
case 5:
FAN_STATE = FAN_5; break;
default:
FAN_STATE = FAN_AUTO; break;
}
FAN_STATE = newFan;
saveState(FAN_ID, newFan);
}
void handleTempMessage(int newTemp) {
TEMP_STATE = newTemp;
saveState(TEMP_ID, newTemp);
}
void sendHeatpumpCommand() {
Serial.println("Power = " + (String)POWER_STATE);
Serial.println("Mode = " + (String)MODE_STATE);
Serial.println("Fan = " + (String)FAN_STATE);
Serial.println("Temp = " + (String)TEMP_STATE);
heatpumpIR->send(irSender, POWER_STATE, MODE_STATE, FAN_STATE, TEMP_STATE, VDIR_AUTO, HDIR_AUTO);
}
void incomingMessage(const MyMessage &message) {
// We only expect one type of message from controller. But we better check anyway.
if (message.isAck()) {
Serial.println("This is an ack from gateway");
}
Serial.print("Incoming change for sensor:");
Serial.print(message.sensor);
Serial.print(", New status: ");
Serial.println(message.getBool());
switch(message.sensor) {
case POWER_ID: {
bool newState = message.getBool();
handlePowerMessage(newState);
break;
}
case MODE_ID: {
int newMode = message.getInt();
handleModeMessge(newMode);
break;
}
case FAN_ID: {
int newFan = message.getInt();
handleFanMessage(newFan);
break;
}
case TEMP_ID: {
int newTemp = message.getInt();
handleTempMessage(newTemp);
break;
}
}
sendHeatpumpCommand();
}