@mfalkvidd when updating the example sketch you could maybe consider my version of the relay sketch which offers some nice additions: https://forum.mysensors.org/topic/6638/multiple-relays-motion-sketch-fully-customizable-optional-timer-manual-override
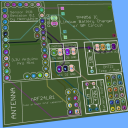
Posts made by HenryWhite
-
RE: π¬ Relay
-
RE: Mains voltage current sensor?
@maghac said in Mains voltage current sensor?:
so I can sense when they're done and alert someone to go empty them.
If you just want to do that, you could also use a vibration sensor to determine whether the dryer/ washing machine has finished or not.
-
RE: Multiple Relays + Motion sketch, fully customizable, optional timer, manual override
@AndreD said in Multiple Relays + Motion sketch, fully customizable, optional timer, manual override:
How to send the relay to the gateway every 30 seconds?
Why would you need that?
-
RE: Sensor dht+relay won't send data or present
@MasMat said in Sensor dht+relay won't send data or present:
@gohan you mean "delay(500)"? Will that not affect the radio function if it is waiting for a command for the relay for example?
That's what wait() is for (mysensors specific) , it will not interfere with the radio commumication.
-
RE: nRF24L01+ and without +. arduino pro micro?
@slowbo here you can find the documentation: https://www.mysensors.org/download/sensor_api_20
There's also a list of all available config options. -
RE: Multiple Relays + Motion sketch, fully customizable, optional timer, manual override
@markjgabb said in Multiple Relays + Motion sketch, fully customizable, optional timer, manual override:
awesome work...this sketch is brilliant....
what happens if you retrigger motion before timer runs out?
will it always handle a restart of the timer correctly?Yes, the timer will be reset.
-
RE: motion sensor and 2 relay code
I finalized my sketch, you can find it here: https://forum.mysensors.org/topic/6638/multiple-relays-motion-sketch-fully-customizable-optional-timer-manual-override
-
Multiple Relays + Motion sketch, fully customizable, optional timer, manual override
Hi!
I recently modified the relay example sketch so that it fits my needs; maybe some of you can make use of my little work too.
Here's what you can do / what the features are:
- Easily add as much Relays / FETs as you want (or as much as the arduino allows) by entering the according pin numbers in the RELAYS[ ]-Array
- You can trigger each relay from your controller
- You can add a motion sensor and specify which (MOTION_ACTIVATED_RELAYS[ ]) of your relays should be triggered through motion and for how long (ON_TIMES[ ])
- The state of the motion sensor will be reported to your controller
- When a relay is changed through motion, the change will also be reported to your controller
- You can send a manual override from your controller, so that the motion will still be reported, but none of the relays will be triggered through motion
/** The MySensors Arduino library handles the wireless radio link and protocol between your home built sensors/actuators and HA controller of choice. The sensors forms a self healing radio network with optional repeaters. Each repeater and gateway builds a routing tables in EEPROM which keeps track of the network topology allowing messages to be routed to nodes. Created by Henrik Ekblad <henrik.ekblad@mysensors.org> Copyright (C) 2013-2015 Sensnology AB Full contributor list: https://github.com/mysensors/Arduino/graphs/contributors Documentation: http://www.mysensors.org Support Forum: http://forum.mysensors.org This program is free software; you can redistribute it and/or modify it under the terms of the GNU General Public License version 2 as published by the Free Software Foundation. ******************************* REVISION HISTORY Version 1.0 - Henrik Ekblad Version 1.1 - HenryWhite DESCRIPTION Example sketch showing how to control physical relays. This example will remember relay state after power failure. Optional attachment of motion sensor to control the relays is possible. Notes: -- The Child-IDs of the attached relays range from 1 up to (1-(NUMBER_OF_RELAYS)) -- Make sure to adjust the potentiometer for triggertime on your motion sensor as leftmost as possible, because the countdown will not start until the motion sensor reports back a "0" (no movement) */ //----------------------- Library Configuration --------------------- //#define MY_DEBUG // uncomment to enable debug prints to serial monitor //#define MY_REPEATER_FEATURE // uncomment to enable repeater functionality for this node //#define MY_NODE_ID 20 // uncomment to define static node ID // Enable and uncomment attached radio type #define MY_RADIO_NRF24 //#define MY_RADIO_RFM69 //#define MY_TRANSPORT_WAIT_READY_MS 1 // uncomment this to enter the loop() and setup()-function even when the node cannot be registered to gw //----------------------- Relay and Motion Sensor Configuration ----------------------- #define MOTION // un-comment to enable motion sensing #define NUMBER_OF_RELAYS 2 // Total number of attached relays. Must be equal to total number of elements in array below! const int RELAYS[] = {3, 5}; // digital pins of attached relays const int MOTION_ACTIVATED_RELAYS[] = {1, 0}; // 1 to trigger the relay through motion, 0 to not trigger. Array length must correspond to RELAYS[] array. const long ON_TIMES[] = {300, 0}; // Specify for each element in MOTION_ACTIVATED_RELAYS, how long the specified relay should be active in seconds. #define RELAY_ON 1 // GPIO value to write to turn on attached relay #define RELAY_OFF 0 // GPIO value to write to turn off attached relay #define MOTION_PIN 2 // The digital input pin of the motion sensor #define MOTION_CHILD_ID 0 // Set the child id of the motion sensor bool ack = 1; // set this to 1 if you want destination node to send ack back to this node //----------------------- DO NOT CHANGE ----------------------------- #include <MySensors.h> MyMessage motion_msg(MOTION_CHILD_ID, V_TRIPPED); // Initialize motion message unsigned long trigger_millis[NUMBER_OF_RELAYS]; // Used for the timer bool lastTripped = 0; // Used to store last motion sensor value bool manual_override = 0; // if this gets set to 1 (e.g. by a switch or a command from the gateway), motion triggering of relays is deactivated MyMessage relay_msg; // Initialize relay message void before() { int i; for (int sensor = 1, i = 0; sensor <= NUMBER_OF_RELAYS; sensor++, i++) { // set relay pins to output mode pinMode(RELAYS[i], OUTPUT); // Restore relay to last known state (using eeprom storage) digitalWrite(RELAYS[i], loadState(sensor) ? RELAY_ON : RELAY_OFF); } // set motion pin to output mode, if MOTION is defined #ifdef MOTION pinMode(MOTION_PIN, INPUT); #endif } void setup() { #ifdef MOTION // give the motion sensor some time to settle Serial.println("Starting up. Please wait 8 seconds..."); delay(8000); #endif } void presentation() { // Send the sketch version information to the gateway and Controller sendSketchInfo("Relay/Motion", "1.0"); // Register all sensors to gw (they will be created as child devices) for (int sensor = 1; sensor <= NUMBER_OF_RELAYS; sensor++) { present(sensor, S_BINARY, "Relay", ack); } #ifdef MOTION present(MOTION_CHILD_ID, S_MOTION, "Motion Sensor", ack); #endif } void loop() { #ifdef MOTION if (!manual_override) { // Read digital motion value bool tripped = digitalRead(MOTION_PIN) == HIGH; if (lastTripped != tripped) { Serial.print("New Motion State: "); Serial.println(tripped); // Send tripped value to gw send(motion_msg.set(tripped ? "1" : "0")); lastTripped = tripped; // Change relay states, send new state to gw and store state in eeprom if (tripped == 1) { for (int i = 0; i < NUMBER_OF_RELAYS; i++) { if (MOTION_ACTIVATED_RELAYS[i] == 1) { digitalWrite(RELAYS[i], RELAY_ON); relay_msg_constructor(i + 1, V_STATUS); send(relay_msg.set(RELAY_ON)); trigger_millis[i] = millis(); Serial.print("Relay "); Serial.print(RELAYS[i]); Serial.println(" turned on"); saveState(i, 1); } } } } for (int i = 0; i < NUMBER_OF_RELAYS; i++) { if (tripped == 1 and MOTION_ACTIVATED_RELAYS[i] == 1 and trigger_millis[i] != 0) { trigger_millis[i] = millis(); } if ((trigger_millis[i] + ON_TIMES[i] * 1000 < millis()) and MOTION_ACTIVATED_RELAYS[i] == 1 and trigger_millis[i] != 0) { digitalWrite(RELAYS[i], RELAY_OFF); relay_msg_constructor(i + 1, V_STATUS); send(relay_msg.set(RELAY_OFF)); Serial.print("Relay "); Serial.print(RELAYS[i]); Serial.println(" turned off"); saveState(i, 0); trigger_millis[i] = 0; } } } else { bool tripped = digitalRead(MOTION_PIN) == HIGH; if (lastTripped != tripped) { Serial.print("New Motion State: "); Serial.println(tripped); // Send tripped value to gw send(motion_msg.set(tripped ? "1" : "0")); lastTripped = tripped; } for (int i = 0; i < NUMBER_OF_RELAYS; i++) { if (MOTION_ACTIVATED_RELAYS[i] == 1 and trigger_millis[i] != 0) { trigger_millis[i] = 0; // reset running timers } } } #endif } void receive(const MyMessage &message) { // Handle incoming relay commands if (message.type == V_STATUS) { // Change relay state if (RELAYS[message.sensor - 1]) { digitalWrite(RELAYS[message.sensor - 1], message.getBool() ? RELAY_ON : RELAY_OFF); // Store state in eeprom saveState(message.sensor - 1, message.getBool()); // Write some debug info Serial.print("Incoming change for sensor:"); Serial.print(message.sensor); Serial.print(", New status: "); Serial.println(message.getBool()); } } // Handle incoming manual override/bypass of motion sensor if (message.type == V_ARMED and message.sensor == 0) { manual_override = message.getBool(); Serial.print("Manual Override: "); Serial.println(manual_override); } } void relay_msg_constructor(int sensor, uint8_t type) { relay_msg.setSensor(sensor); relay_msg.setType(type); }
Additional Notes:
- The Child-IDs of the attached relays range from 1 up to (1-(NUMBER_OF_RELAYS))
- The default Child-ID for the motion sensor is 0
- Make sure to adjust the potentiometer for triggertime on your motion sensor as leftmost as possible, because the countdown-timer will not start until the motion sensor reports back a "0" (no movement)
-
RE: motion sensor and 2 relay code
@gohan said in motion sensor and 2 relay code:
Just remove the FOR cycle in the loop function
It's much easier than that: Just set NUMBER_OF_MOTION_RELAYS to 0
-
RE: motion sensor and 2 relay code
This is my sketch for relays + motion sensor:
You can specify everything in the "#define"-section of the code.
Features:- multiple Relays
- optional motion sensor
- specify which relays should be triggered through the motion sensor
- child ID of the motion sensor: 0
- The Child-IDs of the attached relays range from 1 up to (1-(NUMBER_OF_RELAYS))
A few things are missing at the moment (e.g. report the state of the motion-activated relays to the controller), but it should work out of the box.
/** The MySensors Arduino library handles the wireless radio link and protocol between your home built sensors/actuators and HA controller of choice. The sensors forms a self healing radio network with optional repeaters. Each repeater and gateway builds a routing tables in EEPROM which keeps track of the network topology allowing messages to be routed to nodes. Created by Henrik Ekblad <henrik.ekblad@mysensors.org> Copyright (C) 2013-2015 Sensnology AB Full contributor list: https://github.com/mysensors/Arduino/graphs/contributors Documentation: http://www.mysensors.org Support Forum: http://forum.mysensors.org This program is free software; you can redistribute it and/or modify it under the terms of the GNU General Public License version 2 as published by the Free Software Foundation. ******************************* REVISION HISTORY Version 1.0 - Henrik Ekblad Version 1.1 - HenryWhite DESCRIPTION Example sketch showing how to control physical relays. This example will remember relay state after power failure. Optional attachment of motion sensor to control the relays is possible. Note: The Child-IDs of the attached relays range from 1 up to (1-(NUMBER_OF_RELAYS)) */ //----------------------- Library Configuration --------------------- #define MY_DEBUG // uncomment to enable debug prints to serial monitor //#define MY_REPEATER_FEATURE // uncomment to enable repeater functionality for this node //#define MY_NODE_ID 20 // uncomment to define static node ID // Enable and uncomment attached radio type #define MY_RADIO_NRF24 //#define MY_RADIO_RFM69 //#define MY_TRANSPORT_WAIT_READY_MS 1 // uncomment this to enter the loop() and setup()-function even when the node cannot be registered to gw //----------------------- Relay Configuration ----------------------- const int RELAYS[] = {6, 3, 5}; // digital pins of attached relays #define NUMBER_OF_RELAYS 3 // Total number of attached relays #define RELAY_ON 1 // GPIO value to write to turn on attached relay #define RELAY_OFF 0 // GPIO value to write to turn off attached relay //----------------------- Motion Sensor Configuration --------------- #define MOTION // un-comment to enable motion sensing #define MOTION_PIN 2 // The digital input pin of the motion sensor const int MOTION_RELAY_PINS[] = {5}; // The digital input pins of the relays, which should be triggered through motion #define NUMBER_OF_MOTION_RELAYS 1 // Total number of relays, which should be triggered through motion #define MOTION_CHILD_ID 0 // Set the child id of the motion sensor //----------------------- DO NOT CHANGE ----------------------------- #include <MySensors.h> MyMessage msg(MOTION_CHILD_ID, V_TRIPPED); // Initialize motion message bool lastTripped = 0; void before() { int i; for (int sensor = 1, i = 0; sensor <= NUMBER_OF_RELAYS; sensor++, i++) { // set relay pins to output mode pinMode(RELAYS[i], OUTPUT); // Restore relay to last known state (using eeprom storage) digitalWrite(RELAYS[i], loadState(sensor) ? RELAY_ON : RELAY_OFF); } // set motion pin to output mode, if MOTION is defined #ifdef MOTION pinMode(MOTION_PIN, INPUT); #endif } void setup() { } void presentation() { // Send the sketch version information to the gateway and Controller sendSketchInfo("Relay/Motion", "1.0"); // Register all sensors to gw (they will be created as child devices) for (int sensor = 1; sensor <= NUMBER_OF_RELAYS; sensor++) { present(sensor, S_BINARY); } #ifdef MOTION present(MOTION_CHILD_ID, S_MOTION); #endif } void loop() { #ifdef MOTION // Read digital motion value bool tripped = digitalRead(MOTION_PIN) == HIGH; if (lastTripped != tripped) { Serial.print("New Motion State: "); Serial.println(tripped); // Change relay states and store state in eeprom for (int i = 0; i < NUMBER_OF_MOTION_RELAYS; i++) { digitalWrite(MOTION_RELAY_PINS[i], tripped ? RELAY_ON : RELAY_OFF); saveState(i, tripped); } // Send tripped value to gw send(msg.set(tripped ? "1" : "0")); lastTripped = tripped; } #endif } void receive(const MyMessage &message) { // We only expect one type of message from controller. But we better check anyway. if (message.type == V_STATUS) { // Change relay state digitalWrite(RELAYS[message.sensor - 1], message.getBool() ? RELAY_ON : RELAY_OFF); // Store state in eeprom saveState(message.sensor - 1, message.getBool()); // Write some debug info Serial.print("Incoming change for sensor:"); Serial.print(message.sensor); Serial.print(", New status: "); Serial.println(message.getBool()); } }
-
RE: [SOLVED] No Serial prints for loop(), using MySensors 2.1.1
@korttoma thanks! It works now.
Could have saved me hours of troubleshooting if I knew that prior -
RE: [SOLVED] No Serial prints for loop(), using MySensors 2.1.1
I found the problem, but not the solution.
First, I added
#define MY_TRANSPORT_WAIT_READY_MS 1
to the sketch.
Now the loop()-Function gets executed.
Problem: My node isn't registered by the gateway:New Motion State: 1 80949 !MCO:SND:NODE NOT REG 81582 TSF:MSG:READ,0-0-20,s=255,c=3,t=8,pt=1,l=1,sg=0:0 81588 TSF:MSG:FPAR OK,ID=0,D=1 82950 TSM:FPAR:OK 82952 TSM:ID 82952 TSM:ID:OK 82954 TSM:UPL 82958 TSF:MSG:SEND,20-20-0-0,s=255,c=3,t=24,pt=1,l=1,sg=0,ft=0,st=OK:1 84967 TSM:UPL 84969 TSF:MSG:SEND,20-20-0-0,s=255,c=3,t=24,pt=1,l=1,sg=0,ft=0,st=OK:1
My Gateway is running on an old version of MySensors, I believe it's 1.5.4
Do I have to update the gateway? I thought I could leave it at 1.5.4. -
[SOLVED] No Serial prints for loop(), using MySensors 2.1.1
Hi,
I'm getting all the debug messages if I define #MY_DEBUG, but none of my Serial prints in the loop functions are shown.
However, Serial prints in the receive function are shown.Example sketch:
// Enable debug prints to serial monitor #define MY_DEBUG // Enable and select radio type attached #define MY_RADIO_NRF24 //#define MY_RADIO_RFM69 // Enable repeater functionality for this node #define MY_REPEATER_FEATURE #define MY_NODE_ID 20 #include <MySensors.h> #define RELAY_1 5 // Arduino Digital I/O pin number for first relay (second on pin+1 etc) #define NUMBER_OF_RELAYS 1 // Total number of attached relays #define RELAY_ON 1 // GPIO value to write to turn on attached relay #define RELAY_OFF 0 // GPIO value to write to turn off attached relay void before() { for (int sensor=1, pin=RELAY_1; sensor<=NUMBER_OF_RELAYS; sensor++, pin++) { // Then set relay pins in output mode pinMode(pin, OUTPUT); // Set relay to last known state (using eeprom storage) digitalWrite(pin, loadState(sensor)?RELAY_ON:RELAY_OFF); } } void setup() { } void presentation() { // Send the sketch version information to the gateway and Controller sendSketchInfo("Relay", "1.0"); for (int sensor=1, pin=RELAY_1; sensor<=NUMBER_OF_RELAYS; sensor++, pin++) { // Register all sensors to gw (they will be created as child devices) present(sensor, S_BINARY); } } void loop() { Serial.println("This doesn't print :("); delay(1000); } void receive(const MyMessage &message) { // We only expect one type of message from controller. But we better check anyway. if (message.type==V_STATUS) { Serial.println("This does print!"); } }
It would be really nice if someone could help me with this problem.
-
RE: Shielded nRF24 - better Range?
@Igor-AntoliΔ said in Shielded nRF24 - better Range?:
@HenryWhite That is nice to hear that you ordered 2 items, however now I am confused because you said before that you thik aboit "E01-ML01DP5 Ebyte 20dBm 2100m SPI NRF24L01+PA+LNA", not these red ones (without PA and without external antenna).
the red ones are intended for the nodes, I'm still thinking of getting the item in my first post for my gateway.
-
RE: Shielded nRF24 - better Range?
@xlibor I just ordered 2 of them, will report back if they solved my range problem
-
RE: π¬ Window Sensor with Sensebender (high WAF)
Nice idea!
You can get the window alarm even cheaper: https://www.aliexpress.com/item/Wireless-Small-Independent-Door-Alarm-Sensors-Protection-Door-Window-Magnetic-Burglar-Alarm-Systems-Security-Device-Home/32785764947.html?spm=2114.13010608.0.0.HStmEr -
Shielded nRF24 - better Range?
Hi!
I have significant range problems within my apartment (thick walls I think). I'm currently using the PA+LNA version (wrapped in aluminium foil) as gateway and the standard nRF's as senders/receivers.
However, I just stumbled upon these modules, which are shielded by design: https://www.aliexpress.com/item/1-piece-Long-Range-E01-ML01DP5-Ebyte-20dBm-2100m-SPI-NRF24L01-PA-LNA-2-4GHz-RF/32756789147.html?spm=2114.13010208.99999999.262.GYIB1G
The question is: When I swap my current PA+LNA gateway with one of these shielded modules, can there be an improvement in the range to the other modules?
-
Do repeater nodes increase message delay?
Hi!
although my apartment is not that big, I need at least 2 repeater nodes to cover every room. However, when I use the repeater nodes, everything is delayed 1-2 seconds.
For example: I have multiple motion detectors - the ones which are connected to the gateway over a repeater take 1-2 seconds longer until their "triggered"-message reaches the gateway.
Is this normal?
How can I reduce this delay?I'm using Arduino Pro Minis (5V) for my repeaters. Nothing else runs on them.
-
RE: Rechargeable Lithium Ion Sensor Custom PCB
@toufou said:
You use a motion sensor as i want. You make a reference to a hack to use it with 3.3V instead of 5V. Have you a link to see that?
Yes, here's a picture (the only thing you have to do is solder vcc to the HIGH-pad of your motion sensor):
-
RE: Rechargeable Lithium Ion Sensor Custom PCB
@toufou said:
Hi,
Thanks for your reply.
In the first post, i see schematics, brd and gerber file, but i don't see .ino file to flash arduino pro mini.....You can adapt the example sketches MySensors offers, it shouldn't be hard. Also the sketch will depend on what you plan to use the board for. (Motion, Switch, Temperature,...)
-
RE: Rechargeable Lithium Ion Sensor Custom PCB
@toufou said:
Hi HenryWhite,
Great job, very nice.
I have some questions:
- Why do you sent input voltage to A0? is it for voltage supervision?
- Is it possible to have your sketch file?
Thanks for your sharing,
TouFouHi, yes it's for measuring the battery level.
You can find the schematics and all the other files in my first post. -
RE: [Tutorial] openHAB with serial gateway
@TimO is it possible to send commands to actuators with this method? I have a LED Dimmer Actuator and don't know what I have to do in OpenHAB to send commands to this actuator over the serial gateway..
-
RE: Battery powered smoke sensor
@Poupoune1974 said:
Ok, fine, I'll give a try.
Thanks againNo problem!
(Don't forget a voltage divider to bring down the signal of the smoke detector to max. 3.3v) -
RE: Battery powered smoke sensor
@Poupoune1974 said:
Nice Work, especially the Box.
Could you give details about the wiring ? How do you read the smoke detector ? On piezo ?Most cheap smoke detectors use the same microchip as expensive ones, the only difference is that cheap smoke detectors only use certain pins. So I looked up the type number of the microchip in my smokedetector and took a look at the datasheet, which indicates where the I/O (Input/Output) pin of the microchip is located.
When you supply voltage (at least 1V) to this pin, the smoke detector gets triggered - and the other way round: when the smoke detector triggers, it supplies 9v to the pin. So you can solder a wire to this pin and to the GND pin and connect both with your arduino.
Done!
-
RE: Battery powered smoke sensor
Here's my try of mysensorizing a cheap smoke detector, including 3D-printed case:
Works flawlessly since 2 months ( I modified 3 smoke detectors at my home)
The designed node can read and trigger the smoke detector. However, it currently runs in read-only mode because of the power issues when the node is active all the time.
The next goal is to use the SmartSleep()-function of MySensors 2.0 with my Controller (OpenHAB), to realize reading&triggering of the smoke detector with a node's battery life of at least 6 months.If anyone knows details on how to implement the SmartSleep-Function, I would really appreciate some information!
-
RE: Rechargeable Lithium Ion Sensor Custom PCB
@dakipro here you go:
0_1470866547655_Gerber_rev0.3.zip
Be aware that this are the gerber files for the pcb service from elecrow. Other pcb services may have other specifications!
-
RE: Rechargeable Lithium Ion Sensor Custom PCB
@dakipro said:
Rev 0.3 would then be trully "rechargeable" battery sensor (as in the title), and rev 1.0 would be completelly different, or does it contain same pins as 0.3 (pluss relay which can simply be ignored?).
Rev 1.0 has nearly the same pins, except that you can only connect one switch (Rev 0.3: two switches) - but yes, you can simply ignore the relay, diode and transistor if you don't want to use a relay.
Nonetheless, if you are sure that you will never use the board with a relay, I suggest that you go with Rev. 0.3.And to order it, i usually/only need the .brd and sch files right?
It depends on the pcb manufacturer, but most of the time you will need the gerber files (which can be generated with the .sch file through the CAM-processor with the .cam file (which the specific board house provides).
PS: I ordered my boards from elecrow.
-
RE: Rechargeable Lithium Ion Sensor Custom PCB
@dakipro said:
I've been looking for some time for similar module/board,
(was too lazy to make it myself) and somehow i never found this board.
If I understood you are now on half the battery (after a six months) ?Did you had any tests with the Relay version on battery power?
I guess it will consume quite some power if it needs to keep relay on from the battery?
If it relay doesn't consume a lot of power then it could be very very useful to have it there (or if it is switched for short period of time and not that often)Project looks very good, it has all it needs for generic module, thank you for making it.
Yes, i expect the battery to last ~12 months with one motion sensor + temp/hum sensor installed.
When you want to use the relay, the board normally consumes a lot more power and the battery would not even last 1 month I think, because your board has to be active all the time to receive commands for the relay.If you do not insist on instant relay switching, you could implement the SmartSleep() functionality of mysensors. With this the battery should last way longer, maybe even as long as without relay.
By the way, I got myself a 3D printer and designed a casing for my usecase (motion sensor -- but the case should fit a variety of other components too):
-
RE: Rechargeable Lithium Ion Sensor Custom PCB
A little update in terms of battery lifetime: all nodes are still running, I think they will last at least for 10 months before you have to recharge them.
-
RE: openHAB binding
Thanks for the Binding! It would be very nice if you could implement SmartSleep, so that the node sends a heartbeat message to the Controller when waking up.
-
RE: livolo Glass Panel Touch Light Wall Switch + arduino 433Mhz
@chrlyra how much space is inside the switch? could there be enough room for an arduino+ nrf24 instead of the 433mhz transmitter?
would be great if you could post some photos of the backside (because thats where the electronics are i think?)
-
RE: Wakeup on Interrupt not working
Problem solved!
First, I forgot to activate the internal Pullup:
digitalWrite(DIGITAL_INPUT_SENSOR, HIGH);
Besides that, every signal greater than 0.5V is recognized as interrupt, BUT digitalRead only recognizes signals greater than 1.5V!When my external system triggers, it starts to slowly increase the voltage from 0.5V up to 3.3V. It reaches 1.5V after 5 seconds triggering. I cancelled the test every time after 3-4 seconds, so that's the problem why nothing worked.
-
RE: Wakeup on Interrupt not working
@NickB149 said:
Did you already try applying 5V on the interrupt pin manually?
Maybe the 2.5V is not enough to trigger the interrupt.I'm using a 3.3v arduino pro mini, so the 2.5v should be enough i think?
-
Wakeup on Interrupt not working
Hi,
I have an external system which outputs a signal (~2.5V) on one of its pins under certain circumstances. So I hooked up this output pin to the digital pin 3 on my arduino pro mini (and obviously GND from the external system to GND from the arduino), so that the arduino can tell me whether the external system triggered or not.
Now, when I don't put gw.sleep() in my code, everything works as aspected.
But when I put gw.sleep() in my code and tell it to wake on interrupt, it doesn not work (the arduino doesn't wake up when I trigger the external system)Does somebody has an idea why this is the case? Here's my code:
#include <MySensor.h> #include <SPI.h> //unsigned long SLEEP_TIME = 120000; // Sleep time between reports (in milliseconds) #define DIGITAL_INPUT_SENSOR 3 // The digital input you attached your sensor. (Only 2 and 3 generates interrupt!) #define INTERRUPT DIGITAL_INPUT_SENSOR-2 // Usually the interrupt = pin -2 (on uno/nano anyway) #define CHILD_ID 1 // Id of the sensor child MySensor gw; // Initialize motion message MyMessage msg(CHILD_ID, V_TRIPPED); void setup() { gw.begin(NULL,35,false,AUTO); //Henry Node-ID anpassen! // Send the sketch version information to the gateway and Controller gw.sendSketchInfo("SmokeRF", "1.0"); pinMode(DIGITAL_INPUT_SENSOR, INPUT); // sets the sensor digital pin as input // Register all sensors to gw (they will be created as child devices) gw.present(CHILD_ID, S_DOOR); } void loop() { if (digitalRead(DIGITAL_INPUT_SENSOR)==HIGH) { Serial.println("Alarm"); gw.send(msg.set(1)); // Send value to gw } // Sleep until interrupt comes in on sensor. Send update every two minute. gw.sleep(INTERRUPT,CHANGE,SLEEP_TIME); }
-
RE: Which Lab Power Supply?
@AWI said:
@HenryWhite Looks good. I did something similar (not completely finished) but went too cheap ;-(
Looks good too!
A huge (0.3 V) noise/ripple on the output.... Are you able to measure the ripple with the converter you used? And what kind of transformer did you use?
I can't measure it, because I don't have an oscilloscope (yet)
For powering the module, I used an old 12V laptop power supply. -
RE: Which Lab Power Supply?
Just buy a LTC3780 (link), exchange the pots, hook up a volt/amp display and there you have it: your own lab power supply which can deliver 1V-30V // 0A - 8A, 10A peak.
Here's mine:
-
RE: [Tutorial] openHAB with serial gateway
It just took me 2 hours to realize that you should not use
import java.util.*
when you want to use timer operations like
if (timer=! null) { ... }
Without importing java.util.* , everything works as expected. Someone has an idea why this is the case? Can you reproduce my problem?
-
RE: 110v-230v AC to Mysensors PCB board
@Sefi-Ninio said:
I am assuming that besides adding the second relay, no other components need to be added - no resistors, capacitors, varistors etc.
Is my assumption correct?Thanks!
You need at least a second transistor.
-
RE: 110v-230v AC to Mysensors PCB board
@Cliff-Karlsson said:
Another question about the thermal fuse. I might be wrong on this, but I guess its purpose is to break the power if the HLK overheats. But If placed in the intended place would not the PCB need to be on fire to have the thermal fuse be warmed up to 73 deg C?
I guess you're supposed to glue the thermal fuse to the hlk and run wires from this point to the designated footprint of the fuse on the pcb.
-
RE: NRF24L01+ range of only few meters
@Anduril said:
@HenryWhite yeah I have caps at all my nrfs. Will try with a different power source, as mine is attached to my pc usb as well. At least for testing it should work on batteries.
If that doesn't help try exchanging the Gateway Radio module.
-
RE: NRF24L01+ range of only few meters
@ericvdb said:
the 3.3V on the arduino boards suck. Do not use that, instead use the 5V with your own 3.3V regulator.
I'm using 7 nodes with nRF directly connected to my 3.3v pro mini's vcc. Never had any problems with range, since I'm using the nRF Version with antenna on my gateway.
@Anduril are you using capacitors? Try exchanging the nRF which is attached to your gateway.
-
RE: Is Raspberry a Solid Controller?
I can only speak from OpenHAB, but the New raspberry 2 should have enough computation power.
If you want to store the operating system on an sd card, be prepared to buy every few months (or year, depending on writing activity on the card) a new sd card.It's way better to install the Controller Software on a hdd or ssd. You can modify the raspberry to use a hard drive instead of the sd card.
-
RE: 110v-230v AC to Mysensors PCB board
@toabhishekverma https://en.m.wikipedia.org/wiki/Flyback_diode
in short: It is needed to protect the NPN transistor from damage.
The circuit will work without diode, But eventually damage the transistor. -
RE: 110v-230v AC to Mysensors PCB board
@GertSanders said:
@Yveaux No, also still waiting for the order to arrive. But a coil resistance of 20-25 Ohm means driving the coil with a FET. Not directly from a atmega328 pin.
Correct. Here's how I wired the songle relay on my Lithium Ion Sensor PCB:
@GertSanders said:
@Porky6666 : only switches 3A, SONGLE relay can switch 10A.
I wouldn't trust both of them when it comes to switching high amp devices over 3A. I think there is a reason no german electronics shop sells songle relays
Plus, relays from an electronics shop in your country might cost the same than the songle relays or will just be a tiny bit more expensive.
At least this is true for 5V relays. 3V relays are hard to find (at least in germany)
-
RE: Rechargeable Lithium Ion Sensor Custom PCB
@alexsh1 said:
Just out of curiosity, what do you do when the battery is flat? Take out the node and charge it? Or charging is automated?
You don't have to take anything apart. Just plug in a micro usb loading cable in the Tp4056 and the battery will be charged. Plus, you never need to shutdown the node, charging works simultaneously.
-
RE: Rechargeable Lithium Ion Sensor Custom PCB
@alexsh1 said:
@HenryWhite Did you make any estimates how long your node is running on the fully charged battery?
I'm just testing it
The Version without relay (Rev. 0.3) should be good for at least two months, assuming you want to read temp/humidity every 60 seconds and you have a 2600mAh (smartphone) battery.
I think you can improve the battery life much further, for example with the following:- a better sketch, e.g. bigger sleep time
- removing the power LED on the arduino
- higher battery capacity
The DHT22 is also drawing much current, i think i will replace it in a next version with a Si7021.
PS: The version with relay could never run very long, because you need to keep the node awake in order to send a command to switch the relay. But even if you use the relay version, you could just attach a bigger battery.
-
RE: MySensors connected over power, is this possible?
@hek said:
Using something like this:
https://www.sparkfun.com/products/retired/9185
Unfortunately seems to be retired.
-
RE: Newbie starting his HA project
@rsachoc said:
I think, because I have the NRF2401 connected to my sensebender, that I might have fried it? Thinking 5v would have broken it?
Maybe! But maybe not.
I'm using a FTDI adapter with jumper for my 3.3v/5v pro mini's, and sometimes I forgot to move the jumper to 3V when connecting my 3V pro mini's. Nevertheless, the nrf24l01 "survived" this stupidness every time.
Also, I'm still confused as to how I connect the FTDI adaptor to the sensebender. mfalkvidd mentioned that I need to go "Vcc to Vcc, GND to GND, RxD to TxD and TxD to RxD", howeverthe sensbender pins don't seem to line up this way?
edit just to be clear, the sensebender goes GND GND VCC RXD TXD DTR while the FTDI goes GND DTR RXI TXO PWR CTS GND, so I'm confused how the pins line up, if that makes any sense?
here's how you connect the sensebender to your ftdi:
--Your_FTDI:Sensebender--
GND:GND
CTS:GND
PWR:VCC
TXO:RXD
RXI:TXD
DTR:DTR -
RE: Humidity Sketch w/ DHT22: readings not reliable
@lxz said:
I have the same. For the temperature I always thought that there's less variation in values.
well, i just realised that, goddammit
Since only every 10th reading or so is a failure, i can live with that I think.
@AWI said:
I can confirm that 3.3v in combination with some DHT22 sensors is not a stable solution. I switched to si7021/ sht21 completely (more reliable/ lower consumption/ etc.). other experiences
thanks for the hint. I think I will switch to the si7021 in my pcb design, it seems to be almost the same price as the dht22.
Another solution would be to power the DHT22 directly with 3.7V from my Lithium Battery instead the 3.3V from arduino's VCC. -
RE: Humidity Sketch w/ DHT22: readings not reliable
@barduino said:
Ok, lets see if I can replicate your situation
Would you post the sketch and the log form serial?
for the serial output, see above
default sketch:
/** * The MySensors Arduino library handles the wireless radio link and protocol * between your home built sensors/actuators and HA controller of choice. * The sensors forms a self healing radio network with optional repeaters. Each * repeater and gateway builds a routing tables in EEPROM which keeps track of the * network topology allowing messages to be routed to nodes. * * Created by Henrik Ekblad <henrik.ekblad@mysensors.org> * Copyright (C) 2013-2015 Sensnology AB * Full contributor list: https://github.com/mysensors/Arduino/graphs/contributors * * Documentation: http://www.mysensors.org * Support Forum: http://forum.mysensors.org * * This program is free software; you can redistribute it and/or * modify it under the terms of the GNU General Public License * version 2 as published by the Free Software Foundation. * ******************************* * * REVISION HISTORY * Version 1.0 - Henrik EKblad * * DESCRIPTION * This sketch provides an example how to implement a humidity/temperature * sensor using DHT11/DHT-22 * http://www.mysensors.org/build/humidity */ #include <SPI.h> #include <MySensor.h> #include <DHT.h> #define CHILD_ID_HUM 0 #define CHILD_ID_TEMP 1 #define HUMIDITY_SENSOR_DIGITAL_PIN 3 unsigned long SLEEP_TIME = 60000; // Sleep time between reads (in milliseconds) MySensor gw; DHT dht; float lastTemp; float lastHum; boolean metric = true; MyMessage msgHum(CHILD_ID_HUM, V_HUM); MyMessage msgTemp(CHILD_ID_TEMP, V_TEMP); int node_id = 22; void setup() { gw.begin(); dht.setup(HUMIDITY_SENSOR_DIGITAL_PIN); // Send the Sketch Version Information to the Gateway gw.sendSketchInfo("Humidity", "1.0"); // Register all sensors to gw (they will be created as child devices) gw.present(CHILD_ID_HUM, S_HUM); gw.present(CHILD_ID_TEMP, S_TEMP); metric = gw.getConfig().isMetric; } void loop() { delay(dht.getMinimumSamplingPeriod()); float temperature = dht.getTemperature(); if (isnan(temperature)) { Serial.println("Failed reading temperature from DHT"); } else if (temperature != lastTemp) { lastTemp = temperature; if (!metric) { temperature = dht.toFahrenheit(temperature); } gw.send(msgTemp.set(temperature, 1)); Serial.print("T: "); Serial.println(temperature); } float humidity = dht.getHumidity(); if (isnan(humidity)) { Serial.println("Failed reading humidity from DHT"); } else if (humidity != lastHum) { lastHum = humidity; gw.send(msgHum.set(humidity, 1)); Serial.print("H: "); Serial.println(humidity); } gw.sleep(SLEEP_TIME); //sleep a bit }
-
RE: Humidity Sketch w/ DHT22: readings not reliable
guys, as written above, i'm already using the official temperature/humidity sketch..
So there is agw.wait(dht.getMinimumSamplingPeriod());
before the reading.
I tried to increase this period to static 2,5 seconds (default interval should be 2s according to datasheet), but sadly that didn't help.Here's what the serial monitor shows (strange, isn't it?):
-
Humidity Sketch w/ DHT22: readings not reliable
Hi!
When using the official temperature/humidity sketch with a 10k resistor between data and 3.3V VCC, the readings are not stable; I have lots of
"Failed reading temperature from DHT"
"Failed reading humidity from DHT"It seems that the temperature reading is successfull only every 4-5th time, while the humidity reading works every 1-2nd time.
I'm using an 8mhz arduino pro mini 3.3V.
Any ideas why this happens? Could a capacitor solve this problem? -
RE: Newbie starting his HA project
@rsachoc Well, you could
-
buy a thinner soldering tip if yours is to big (make sure the tips fit to your soldering iron)
-
try to desolder the bridge and solder it to the right place
-
break the bridge with pliers and just solder the lower two solder points together. this would be possible even with a thicker soldering iron.
If you want to switch continously between 5v and 3.3v, you should consider buying another ftdi adapter with jumper pins (or try to solder 3 pinheaders to the three solder pads)
-
RE: Newbie starting his HA project
@rsachoc said:
Here's where I'm struggling - I bought an FTDI adaptor, which I thought was 5v and 3.3v, but from what I can see it's 5v.
picture of the adaptor?
some adapters have a jumper or solder bridge to switch between 5V and 3.3V -
Old sketch for battery measurement
Hi @hek and other admins,
the linked sketch for Battery Measurement ( http://www.mysensors.org/build/battery#measuring-and-reporting-battery-level ) is not up-to-date; it won't compile when using the new MySensors library's header files.
Could you please update this? I think especially for beginners this could be confusing. -
RE: My MYSX Multisensors board
@scalz said:
3d printer...
OT: btw I decided to build my own 3D printer too, since you put that idea into my head and i can't wait until i can afford an ultimaker..
-
RE: Rechargeable Lithium Ion Sensor Custom PCB
@m26872 said:
Nice, but I think R3 =330k would give you a better battery voltage monitoring divider ratio (Vmax 4.43V). Maybe a little higher total resistance would limit current loss though (even 10M+3M would work).
Maybe something useful here.Good point!
Didn't thought about that.
-
RE: Rechargeable Lithium Ion Sensor Custom PCB
I just received my ordered PCBs (Revision 0.1) from Elecrow. The PCBs are very well made for that low price! (I got 14PCBs for 14$)
It fits nicely in a 75x75mm electric box (not the prettiest housing, but very cheap and easily processable for drilling holes for the connections; plus you can paint it to make it more beautiful
)
Since it is revision 0.1, I had to cut one pcb trace (V+ from POWER to Arduino's V_IN) and connect V+ from POWER manually with Arduino's RAW input.
Soldering directly to the battery went better than I thought!
-
RE: Rechargeable Lithium Ion Sensor Custom PCB
Here it is: Revision 1.0
Changes:
- Now with additional relay, properly connected using 2N2222A transistor, flyback diode, 1kΞ© resistor and terminal block for easy attaching of "switchable things" to the relay
- proper alignment of DHT22
Schematics (Rev 1.0)
Board view (Rev 1.0)
3D board view (Rev 1.0)
Build files
Eagle files: 0_1453762986505_Sensorboard_v1.0.zip
Gerber files: 0_1453763061782_Sensorboard_v1.0_Gerber.zip -
RE: 110v-230v AC to Mysensors PCB board
@TD22057 said:
@petewill said:
I don't remember where I read this but it was advised not to use resettable fuses with the HLK. Basically it was said that if the fuse were to blow the HLK would have already been stressed to the point where it may be unsafe and it's best to replace the whole device. I wish I could remember where I read that but I'm not sure...
I'm 90% certain that was from the guy who did the power tests of the HLK. I believe he was saying that these small PSU's don't handle heat or spikes well and if the fuse goes, you should assume the PSU is unsafe as well and replace it.
Here it is I guess: http://lygte-info.dk/review/Power Mains to 5V 0.6A Hi-Link HLK-PM01 UK.html
Quote: "I would place a fuse or fusible resistor before the converter, the fuse is not supposed to be replaceable, when it blows it is time to replace the converter."
-
RE: Where do you buy your Hardware?
I think you only have these two options for most modules (arduino, sensors, etc.):
- buy it cheap and wait long
or - buy it expensive and get it within a few days
From my experience I can tell you that items on aliexpress will mostly arrive 3-4 weeks after purchase (I'm living in germany too). I ordered at least 150 different items there over the past few years.
- buy it cheap and wait long
-
RE: Another beginner project
@Astrofotograf said:
So you add the 433 MHz modules directly to the rapberry GPIO pins and use the arduino just for the sensors?
Besides pilight there's also rcswitch-pi, basically rcswitch for the raspberry pi.
-
RE: Where next with making prototype more permanent?
@stubtoe said:
I saw that the other day. It is pretty neat and given me a few ideas.
I suppose I was also asking (although not very well worded!) where's a good resource when you want to design and manufacture your own bespoke PCB? Is there a good book anyone can recommend?
For Designing a PCB, you can watch tutorials on YouTube (search e.g. for "eagle tutorial" if you want to design your board with eagle. I started two weeks ago and after one day of watching tutorials I built my own board in eagle
A good address for cheap pcb manufacturers is http://pcbshopper.com/
-
RE: Another beginner project
@Astrofotograf nice, didn't knew they were that cheap nowadays. How are you going to get the (binary) code from these 433mhz switches? Do you have any useful links/tutorials for that?
-
RE: Another beginner project
@Astrofotograf said:
can't wait until the parts arrive..
Hi! Which "Funklichtschalter (Sender)" are you going to use?
-
RE: Rechargeable Lithium Ion Sensor Custom PCB
I just added revision 0.3 to the first post:
@HenryWhite said:
Rev 0.3: swapped footprints of DHT22 and prototyping area to prevent false temp measurements when charging the battery; connected TP4056 OUT to arduino's RAW instead of VCC_IN (so the internal voltage regulator is used)
sensorboard_v0.3.sch
sensorboard_v0.3.brd -
RE: Rechargeable Lithium Ion Sensor Custom PCB
@flopp said:
@HenryWhite
Wow, what are your low/max limit?I must admit i didn't understand that
At 2% battery state (according to what is shown in the android UI) the s5 battery still outputs 3.43V (measured with multimeter and compared to android app "Ampere")
Even at 1% the voltage is still above 3.3V:
But: I just discovered that the galaxy s5's battery is a 3.85V li-ion battery with a charging voltage of 4.4V instead of 4.2V..
But even then, the 3.8V S4 battery which I'm going to use for my sensors will approximately reach less than 3.3V not before the battery is under 5% charging state I think.
-
RE: Rechargeable Lithium Ion Sensor Custom PCB
Again, to prove my point that you don't need to go below 3.3V (at least with modern smartphone batteries), I just measured my Galaxy S5's battery at 4% battery state. The measured voltage is 3.65V.
-
RE: Rechargeable Lithium Ion Sensor Custom PCB
@Heizelmann you're right, the module gets pretty warm.
Here I found some more measurements for the Tp4056: http://lygte-info.dk/review/Review Charger TP4056 UK.htmlAlthough the board is designed to bend the DHT22 over the prototyping area so that it is horizontal and not vertical aligned, I think I could swap positions of the prototyping area and the mounting point for the DHT22.
To keep the Tp4056 cooler, you could add a small heatsink to it or replace the resistor so that it charges with lower current.
-
RE: Rechargeable Lithium Ion Sensor Custom PCB
@scalz said:
I am building my 3d printer actually because I want custom things (not too much ugly)
I thought about that too, but then I saw how much calibration, try&error etc. you need to assemble your own 3D printer. And after that it's still a hassle to get prints with good quality.
Because of that I will just save money for the ultimaker 2..@scalz said:
I was talking about how to wire between the batt and the board. So you will solder wire on it?
Yes, I will solder the contacts of the battery directly to the tp4056 module. Because you don't have to replace the battery (until it breaks) this method should be fine.
-
RE: Rechargeable Lithium Ion Sensor Custom PCB
@scalz said:
How do you plan to hook the battery to your board?
As smartphone batteries are usually fully enclosed, I will just stick the battery (I will use the galaxy s4 batteries) under the pcb.
The whole package should fit nicely in a 75x75x40 distribution box, which will only cost a few cents in your local hardware store.
Only downside of the boxes is that they are a bit ugly
But since I don't have a 3D printer yet i will go with that solution and maybe paint the boxes black or white. -
RE: Rechargeable Lithium Ion Sensor Custom PCB
@flopp said:
Now I see, there are pads for battery and pads for out. Looks good
Correct. The old Tp4056 modules do not have the OUT terminals (and therefore won't fit on the pcb I designed in this thread)
@akumar said:
On a low power node this could work.
Why should it only work on a low power node?
-
RE: Rechargeable Lithium Ion Sensor Custom PCB
@akumar It's the same problem as with all lipos. But you have to consider that it will take a really long time until the battery hits 3.4V or less. There are even smartphone batteries with 3000+ mAh which are very small.
Also it seems to be good for battery lifetime when the voltage does not drop that much compared to using a step-up converter below 3.4V.
-
RE: Rechargeable Lithium Ion Sensor Custom PCB
@mfalkvidd said:
Power on RAW pin results in the regulator being in use.
Power on Vcc bypasses the regulator.indeed. seems like I mixed up something there
I will update the eagle files when i have time to. -
RE: Rechargeable Lithium Ion Sensor Custom PCB
@akumar said:
make sure the linear voltage regulator on the arduino will be able to provide the 3.3v if battery voltage drops below 3.3v (so from 3.3v to 2.8v).
That's not necessary - for this case you have the battery measurement circuit as mentioned in my previous post.
-
RE: Safe In-Wall AC to DC Transformers??
Here's some more valuable information about the HLK: http://lygte-info.dk/review/Power Mains to 5V 0.6A Hi-Link HLK-PM01 UK.html
-
RE: Rechargeable Lithium Ion Sensor Custom PCB
@akumar said:
The TP4056 board will protect the cell if voltage drops bellow 2.8v. The arduino and nrf24 module should be fine at those values. This might not be the case for all sensors...
That's right, but for this case (which will only happen once a year or less (depending on configuration and used lipo battery) you have the battery measurement supported through mysensors onboard, which can tell you if the voltage drops below a certain level.
Also make sure to get the new tp4056 because the old and common one does not have a battery protection circuit!
@akumar said:
However when charging or at 4.2V the nrf24 module will exceed its 3.6V max input power.
No, the nRF24 is hooked up to VCC of the arduino, which has an internal voltage regulator. So the VCC output of the arduino should always be max. 3.3V, as long as the input is below 12V.
-
RE: Rechargeable Lithium Ion Sensor Custom PCB
@flopp said:
Can I use your files and modify it?
Yes, absolutely.
But I don't think you need a voltage regulator. At least on the arduino I ordered there's already an onboard regulator which is rated for up to 12V DC input. -
RE: Rechargeable Lithium Ion Sensor Custom PCB
@Heizelmann said:
What I tested is only this antenna modification. For me it really helps. May be this can be taken into account for the layout.
I plan to use this mod too. I'm curious if the additional wire(s) has/have to be straight or if you can twist it/them. If you use a single straight wire, it should already be compatible with the layout, the wire would be above the arduino.
-
RE: Rechargeable Lithium Ion Sensor Custom PCB
@AWI: I will think about it
@Heizelmann said:
I think, on Rev 0.2 the download link for the board is wrong, it is the same as for Rev 0.1.
I think it is also imported that the antenna will not be covered by the shield pcb (see http://forum.mysensors.org/topic/1109/sensor-shield-for-arduino-pro-mini-3-3v-with-boost-up-regulator)
yeah the link is the same, i will update it. Did you made measurements to compare the range of the nRF when mounted onto the board and mounted sticking out? I'm not sure but I don't think this would make any difference, as there are no routes under the antenna or even a ground fill on the board.
-
RE: Rechargeable Lithium Ion Sensor Custom PCB
Updated to Rev 0.2:
- SMD capacitor footprint added (see first post)
- made sure no route is under the nRF antenna to prevent interference
-
RE: Rechargeable Lithium Ion Sensor Custom PCB
@mfalkvidd said:
@HenryWhite said:
Is there any reason the cap should be placed on the board instead of the nRF itself? If this is the case I could add footprints for a SMD cap under the nRF
Just to make soldering easier. I soldered 15 caps to 15 nRFs today so I'm a bit fed-up, that's all
I think I will add a footprint for a SMD cap under the nRF, there's plenty of unused space anyway
-
RE: Rechargeable Lithium Ion Sensor Custom PCB
@mfalkvidd said:
Great work!
This might be a stupid question but why haven't you added a slot for soldering the capacitor for the nrf?At first I thought about it, but then I realized you can just solder the capacitor directly onto the nRF which will make everything only a few mm higher, so I don't have to waste space on the board itself.
Best thing would be to use a SMD capacitor to solder onto the nRF VCC and GND pins - it won't take more space than without the SMD cap.Is there any reason the cap should be placed on the board instead of the nRF itself? If this is the case I could add footprints for a SMD cap under the nRF
-
RE: Rechargeable Lithium Ion Sensor Custom PCB
If you can provide a valid eagle footprint library for these kind of pro mini clone I could make a version for that clone too
-
RE: Rechargeable Lithium Ion Sensor Custom PCB
@MikeF said:
Which 'flavour' of Pro Mini are you going with?.
Whoops, sorry. Forgot to point that out. It is the pinout version most of the chinese clones use, for example: http://www.aliexpress.com/item/1pcs-lot-Pro-Mini-328-Mini-3-3V-8M-ATMEGA328-ATMEGA328P-AU-3-3V-8MHz-for/32313595044.html
-
Rechargeable Lithium Ion Sensor Custom PCB
Hi!
Since a bunch of people already made a custom MySensors PCB, I decided to get used to Eagle and create my own board to fit my needs
The key difference compared to other custom PCBs is the Lithium Ion Charging Circuit with micro USB connector. So you can just grab a flat smartphone battery with 1000 mAh or more and stick it under the PCB! This way you never have to worry about changing batteries, and even if your configuration consumes a lot of power, you can quickly recharge your sensor through the micro USB connector of the Li-Ion Charging Module.The board is currently only designed to work with the Arduino Pro Mini 3.3V version.
Version 0.1 is tested, I received the PCBs on 01/26/2016. Everything works fine (good radio range), there is only one little problem with the connection of the battery (but it is fixable; see post 52 in this thread for a fix)
So here are the specifications and features of my board:
- designed for cheap 3.3V Arduino Pro mini clones from china, for example this one
- 5x5cm
- uses jumper bridge to simply connect and disconnect the battery
- easy attaching of motion sensor (use 3.3V mod), DHT22 for Temp and Humidity Measurement and one switch (for example button- or reedswitch)
- small prototyping area on the backside
- uses TP4056 Li-Ion single cell Micro USB 1A charging circuit with battery protection (disconnects the power automatically if voltage drops below 2.4V
------> make sure you get the NEW version of the TP4056 with battery protection circuit!
remember to charge your li-ion 1-cell rechargeable battery with maximum 1C, so if you want to use a battery below 1000 mAh you need to make modifications to the module or the charging current will be way to high, which could result in an explosion (worst case) - I suggest to use the board with a smartphone battery, as they usually are very small and flat and have a high capacity for small money. I will use the galaxy s4 battery because it fits nearly perfect under the board and has 2600 mAh.
- Now with additional relay, properly connected using 2N2222A transistor, flyback diode, 1kΞ© resistor and terminal block for easy attaching of "switchable things" to the relay
Schematics (Rev 1.0)
Board view (Rev 1.0)
3D board view (Rev 1.0)
Build files
Rev 1.0:
- Now with additional relay, properly connected using 2N2222A transistor, flyback diode, 1kΞ© resistor and terminal block for easy attaching of "switchable things" to the relay
- proper alignment of DHT22
Eagle files: 0_1453762986505_Sensorboard_v1.0.zip
Gerber files: 0_1453763061782_Sensorboard_v1.0_Gerber.zipRev 0.3: swapped footprints of DHT22 and prototyping area to prevent false temp measurements when charging the battery; connected TP4056 OUT to arduino's RAW instead of VCC_IN (so the internal voltage regulator is used)
sensorboard_v0.3.sch
sensorboard_v0.3.brdRev 0.2: added footprint for 47uF SMD capacitor standard 1210 (i guess many other smd caps will fit too)
sensorboard_v0.2.sch
sensorboard_v0.2.brdRev. 0.1
untitled.sch untitled.brd -
RE: Detecting if a persons is laying in his/her bed
@TheoL said:
@HenryWhite Thank you verry much. I think I can mount it in a pillow. I'm currently working on a IOT alarm clock and I'll be incorperating one of these in the final solution/ That way the alarmclock knows if the person is in bed when an alarm is triggered and will not start the alarm if no person is present in the bed. For most part this is not a mysensors project. So I'm not sure if I should post the project on this forum.
If you believe it or not, I had this idea also a few weeks ago and I am currently thinking about trying it
The problem is that the FSR402 is only for max. 10kg.
I think the pillow would not be the best solution because if you move your head you can easily get out of range of the force sensor (unless you use multiple force sensors in the "pillow-area") -
RE: Detecting if a persons is laying in his/her bed
Hi theol, i think this is exactly what you're looking for: http://ebay.com/itm/220916323198
-
RE: [Tutorial] openHAB with serial gateway
@TimO thank you so much! it works now. Any idea why i had to assign a static id? i thought the gateway should tell the node a dynamic id automatically..
-
RE: [Tutorial] openHAB with serial gateway
When I look at the serial monitor output for my motion sensor, it clearly gives me a "0" or "1" depending if movement is detected or not.
But when I look at the serial monitor of my gateway, it only receives the same message no matter if motion started (motion sensor serial monitor: 1) or ended (motion sensor serial monitor: 0)
-
RE: [Tutorial] openHAB with serial gateway
@Dave-Dan i tried it, but the motion sensor is still sending only zero's.... even if it is not triggered.
I got these motion sensors: http://www.amazon.de/Pyroelectrische-Infrarot-Bewegung-Sensor-Detektor/dp/B008AESDSY/ -
RE: [Tutorial] openHAB with serial gateway
Can someone help me? I want to integrate a motion sensor in OpenHAB but I don't know how to distinguish between the motion start and end. here's my openhab console log:
-
RE: Serial Gateway on Arduino Pro Mini - "Gateway setup complete" not showing up
thanks, it worked!
can you explain to me why this worked? -
Serial Gateway on Arduino Pro Mini - "Gateway setup complete" not showing up
Hi,
I uploaded the sketch for the serial gateway to my 5v arduino pro mini with connected 3.3v regulator and the NRF24L01+ Radio.
But when I look at the serial monitor, it just gives me "connecting at 9600" and nothing else. Shouldn't there be a "Gateway startup complete" ?