@dbemowsk Any progress I want to do this as well?
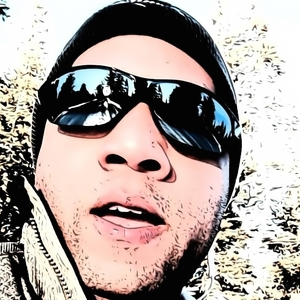
Posts made by DrJeff
-
RE: RGB LED strip
@maghac Oh yes by the way the code above works great just added some code for Motion sensor and Now I have a much better light.
/** * This program is free software; you can redistribute it and/or * modify it under the terms of the GNU General Public License * version 2 as published by the Free Software Foundation. * * LED STRIP sketch for Mysensors ******************************* * * REVISION HISTORY * 1.0 * Based on the example sketch in mysensors * 1.1 * fadespeed parameter (send as V_VAR1 message) * HomeAssistant compatible (send status to ack) * 1.2 * OTA support * 1.3 * Power-on self test * 1.4 * Bug fix * 1.5 * Other default values * 1.6 * Repeater feature * 1.7 * Multitasking. Alarm, Relax and normal modes. */ #define MY_OTA_FIRMWARE_FEATURE #define MY_REPEATER_FEATURE #define MY_NODE_ID 31 // added all the motion stuff DrJeff September 10, 2017 #define MOTION_1 3 // The digital input you attached your motion sensor. (Only 2 and 3 generates interrupt!) #define MOTION_ID 90 // Id of the sensor child uint8_t lastMotion_1 = 0; unsigned long previousMillis1 = 0; unsigned long motionDelay1 = 10000; // interval at which to keep motion sensor trippped (milliseconds). Used to prevent too frequent updates to Vera. /// #define MY_RADIO_NRF24 #define MY_DEBUG #include <MySensors.h> #define CHILD_ID_LIGHT 1 #define SN "LED Strip" #define SV "1.7" MyMessage lightMsg(CHILD_ID_LIGHT, V_LIGHT); MyMessage rgbMsg(CHILD_ID_LIGHT, V_RGB); MyMessage dimmerMsg(CHILD_ID_LIGHT, V_DIMMER); // added all the motion stuff DrJeff September 10, 2017 MyMessage motion(MOTION_ID, V_TRIPPED); // int current_r = 255; int current_g = 255; int current_b = 255; int target_r = 255; int target_g = 255; int target_b = 255; int save_r; int save_g; int save_b; float delta_r = 0.0; float delta_g = 0.0; float delta_b = 0.0; char rgbstring[] = "ffffff"; int on_off_status = 0; int dimmerlevel = 100; int fadespeed = 20; unsigned long last_update = 0; int tick_length = 10; int fade_step = 0; int program_timer; int program_cycle; #define REDPIN 6 #define GREENPIN 4 #define BLUEPIN 5 #define LIGHT_NORMAL 0 #define LIGHT_FADING 1 #define PROGRAM_NORMAL 0 #define PROGRAM_ALARM 1 #define PROGRAM_RELAX 2 int light_mode = LIGHT_NORMAL; int program_mode = PROGRAM_NORMAL; #define RELAX_SPEED 50 #define MAX_CYCLES_RELAX 7 const int program_param_RELAX[MAX_CYCLES_RELAX][3] = { {255, 32, 0}, {255, 32, 16}, {255, 16, 32}, {255, 128, 0}, {255, 32, 00}, {255, 32, 32}, {255, 0, 32} }; void setup() { // Fix the PWM timer. Without this the LEDs will flicker. TCCR0A = _BV(COM0A1) | _BV(COM0B1) | _BV(WGM00); // Output pins pinMode(REDPIN, OUTPUT); pinMode(GREENPIN, OUTPUT); pinMode(BLUEPIN, OUTPUT); // added all the motion stuff DrJeff September 10, 2017 pinMode(MOTION_1, INPUT); // } void presentation() { // Send the Sketch Version Information to the Gateway sendSketchInfo(SN, SV); present(CHILD_ID_LIGHT, S_RGB_LIGHT); // added all the motion stuff DrJeff September 10, 2017 present(MOTION_ID, S_MOTION); // } void selftest() { on_off_status = 1; current_r = 255; current_g = 0; current_b = 0; set_hw_status(); wait(100); current_r = 0; current_g = 255; set_hw_status(); wait(100); current_g = 0; current_b = 255; set_hw_status(); wait(100); current_r = 255; current_g = 255; set_hw_status(); wait(100); on_off_status = 0; } void loop() { static bool first_message_sent = false; if ( first_message_sent == false ) { selftest(); set_hw_status(); send_status(1, 1, 1); first_message_sent = true; } // added all the motion stuff DrJeff September 10, 2017//loop for motion unsigned long currentMillis = millis(); if(currentMillis - previousMillis1 > motionDelay1) { uint8_t motionDetect1 = digitalRead(MOTION_1); if(motionDetect1 != lastMotion_1) { Serial.print("Motion 1 = "); Serial.println(motionDetect1); // gw.send(motionStatus_1.set(motionDetect1 ? "1" : "0")); // Send tripped value to gw send(motion.set(motionDetect1)); if(motionDetect1 == 1) { previousMillis1 = currentMillis; //"Tripped" delay } else { previousMillis1 = currentMillis - motionDelay1 + 1000; //"Not tripped" delay for 1 second to stop rapid "not tripped" and "tripped" updates to Vera } lastMotion_1 = motionDetect1; } } //end of motion loop unsigned long now = millis(); if (now - last_update > tick_length) { last_update = now; if (light_mode == LIGHT_FADING) { calc_fade(); } if (program_mode > PROGRAM_NORMAL) { handle_program(); } } set_hw_status(); } void receive(const MyMessage &message) { int val; if (message.type == V_RGB) { Serial.print( "V_RGB: " ); Serial.println(message.data); long number = (long) strtol( message.data, NULL, 16); // Save old value strcpy(rgbstring, message.data); // Split it up into r, g, b values int r = number >> 16; int g = number >> 8 & 0xFF; int b = number & 0xFF; init_fade(fadespeed, r, g, b); send_status(0, 0, 1); } else if (message.type == V_LIGHT || message.type == V_STATUS) { Serial.print( "V_LIGHT: " ); Serial.println(message.data); val = atoi(message.data); if (val == 0 or val == 1) { on_off_status = val; send_status(1, 0, 0); } } else if (message.type == V_DIMMER || message.type == V_PERCENTAGE) { Serial.print( "V_DIMMER: " ); Serial.println(message.data); val = atoi(message.data); if (val >= 0 and val <=100) { dimmerlevel = val; send_status(0, 1, 0); } } else if (message.type == V_VAR1 ) { Serial.print( "V_VAR1: " ); Serial.println(message.data); val = atoi(message.data); if (val >= 0 and val <= 2000) { fadespeed = val; } } else if (message.type == V_VAR2 ) { Serial.print( "V_VAR2: " ); Serial.println(message.data); val = atoi(message.data); if (val == PROGRAM_NORMAL) { stop_program(); } else if (val == PROGRAM_ALARM || val == PROGRAM_RELAX) { init_program(val); } } else { Serial.println( "Invalid command received..." ); return; } } void set_rgb(int r, int g, int b) { analogWrite(REDPIN, r); analogWrite(GREENPIN, g); analogWrite(BLUEPIN, b); } void init_program(int program) { program_mode = program; program_cycle = 0; save_rgb(); if (program == PROGRAM_ALARM) { light_mode = LIGHT_NORMAL; current_r = 255; current_g = 255; current_b = 255; program_timer = 50; } else if (program == PROGRAM_RELAX) { program_timer = 300; init_fade(fadespeed, program_param_RELAX[program_cycle][0], program_param_RELAX[program_cycle][1], program_param_RELAX[program_cycle][2]); } } void handle_program() { program_timer--; if (program_mode == PROGRAM_ALARM) { if (program_timer == 0) { program_timer = 50; if (program_cycle == 0) { program_cycle = 1; current_r = 0; current_g = 0; current_b = 0; } else { program_cycle = 0; current_r = 255; current_g = 255; current_b = 255; } } } else if (program_mode == PROGRAM_RELAX) { if (light_mode == LIGHT_NORMAL) { program_cycle = (program_cycle+1) % MAX_CYCLES_RELAX; Serial.print("Next cycle step "); Serial.println(program_cycle); init_fade(fadespeed * RELAX_SPEED, program_param_RELAX[program_cycle][0], program_param_RELAX[program_cycle][1], program_param_RELAX[program_cycle][2]); } } } void stop_program() { restore_rgb(); light_mode = LIGHT_NORMAL; program_mode = PROGRAM_NORMAL; } void save_rgb() { save_r = current_r; save_g = current_g; save_b = current_b; } void restore_rgb() { current_r = save_r; current_g = save_g; current_b = save_b; } void init_fade(int t, int r, int g, int b) { Serial.print( "Init fade" ); light_mode = LIGHT_FADING; target_r = r; target_g = g; target_b = b; fade_step = t; delta_r = (target_r - current_r) / float(fade_step); delta_g = (target_g - current_g) / float(fade_step); delta_b = (target_b - current_b) / float(fade_step); } void calc_fade() { if (fade_step > 0) { fade_step--; current_r = target_r - delta_r * fade_step; current_g = target_g - delta_g * fade_step; current_b = target_b - delta_b * fade_step; } else { Serial.println( "Normal mode" ); light_mode = LIGHT_NORMAL; } } void set_hw_status() { int r = on_off_status * (int)(current_r * dimmerlevel/100.0); int g = on_off_status * (int)(current_g * dimmerlevel/100.0); int b = on_off_status * (int)(current_b * dimmerlevel/100.0); set_rgb(r, g, b); } void send_status(int send_on_off_status, int send_dimmerlevel, int send_rgbstring) { if (send_rgbstring) send(rgbMsg.set(rgbstring)); if (send_on_off_status) send(lightMsg.set(on_off_status)); if (send_dimmerlevel) send(dimmerMsg.set(dimmerlevel)); }
-
RE: RGB LED strip
@maghac Wow! I guess I haven't been around here for a while.
Thanks
-
RE: How To: Make a Simple/Cheap Scene Controller (with video)
Never mind part of the code was commented out. I wasn't sure because I just started to use OTA. But it's all good now its working fine. Here it is:
#define MY_DEBUG #define MY_RADIO_NRF24 #define MY_OTA_FIRMWARE_FEATURE #include <SPI.h> #include <MySensors.h> #include <Keypad.h> #define NODE_ID AUTO // or set to AUTO if you want gw to assign a NODE_ID for you. #define SN "Scene Controller" #define SV "2.0" #define KEYPAD_CHILD_ID 95 MyMessage scene(KEYPAD_CHILD_ID, V_SCENE_ON); //scene ON MyMessage scene2(KEYPAD_CHILD_ID, V_SCENE_OFF); //scene OFF const byte ROWS = 4; //four rows const byte COLS = 1; //one column char keys[ROWS][COLS] = { {'1'}, {'2'}, {'3'}, {'4'} }; byte rowPins[ROWS] = {6, 7, 4, 5}; //connect to the row pinouts of the keypad byte colPins[COLS] = {8}; //connect to the column pinouts of the keypad Keypad keypad = Keypad( makeKeymap(keys), rowPins, colPins, ROWS, COLS ); byte lastState; void setup() { sendSketchInfo(SN, SV); present(KEYPAD_CHILD_ID, S_SCENE_CONTROLLER); keypad.addEventListener(keypadEvent); } void loop() { char key = keypad.getKey(); } void keypadEvent(KeypadEvent key) { switch (keypad.getState()) { case PRESSED: lastState = 1; break; case HOLD: lastState = 2; break; case RELEASED: int keyInt = key - '0'; //Quick way to convert Char to Int so it can be sent to controller if (lastState == 2) { keyInt = keyInt + 4; //If button is held, add 4. If using more than 4 buttons this number will need to be changed send(scene2.set(keyInt)); } else { send(scene.set(keyInt)); } break; } }
-
RE: How To: Make a Simple/Cheap Scene Controller (with video)
Not getting the Hold on buttons working, everything else works. Any ideas why the long press doesn't work?
-
RE: How To: Make a Simple/Cheap Scene Controller (with video)
@hek
Yes I read but was soooo lazy! I just wanted it already doneI just update one of my old sensors it was a simple single relay. My others are way more complicated! I will give this a try.
-
RE: How To: Make a Simple/Cheap Scene Controller (with video)
@petewill Have you updated this code to 2.0 yet? What is different?
-
RE: 💬 Building a Raspberry Pi Gateway
The line above
./examples_linux/mysGatewaymsyGateway -h
needs to be corrected to
./examples_linux/mysGateway -h
Thanks for all the hard work! Awesome! -
RE: Wateringsystem
@Lars65
Good times!
Yes I meant "saw" thanks for the good laugh. Did you finish yet? -
RE: Wateringsystem
Put your pants back on! No working Naked!!
I laughed when I say the jeans in the picture. Have fun building! -
RE: 2.0 Gateway with 1.5 sensors
Running a newly upgraded 2.0 Gateway with old Nodes so far so good. I will be updating as I get to them.
-
RE: MySensors 2.0.0 Released
@tante-ju
Jumped onto my ESXi server with a windows VM windows setup and the problem you are describing for the mac went away. I have El Cap on my mac with the same type of problems. Don't have an answers but windows worked fine. Wasted a couple of hours trying to fix also. -
RE: Temps rounded to whole numbers?
@Yveaux said:
float temperature = round(gw.getConfig().isMetric ? sensors.getTempCByIndex(i) : sensors.getTempFByIndex(i)));
I will try this Thanks for the help.
-
Temps rounded to whole numbers?
Trying to get the DS18B20 to round to a whole number no decimal point. I'm using this and getting the temps down to .1 decimal point.
// Fetch and round temperature to one decimal float temperature = static_cast<float>(static_cast<int>((gw.getConfig().isMetric ? sensors.getTempCByIndex(i) : sensors.getTempFByIndex(i)) * 10.)) / 10.;
What do I do next to get a whole number only, I don't like all the minor changes flooding my controller.
complete node code is below.
-
RE: Problem with DS18b20
@lcarlier try moving your resistor to the same pins but closer to the DS18B20, right now it's not limiting the power to it. The row between the DS18B20 and the wires.
-
RE: MYSBootloader 1.3 pre-release & MYSController 1.0.0beta
@tekka , Time to try Thanks!
-
RE: MYSBootloader 1.3 pre-release & MYSController 1.0.0beta
@tekka Cool thanks, can I use Arduino as ISP to program bootloader?
-
RE: MYSBootloader 1.3 pre-release & MYSController 1.0.0beta
Does the gateway need the OTA firmware (flashed) written to it as well as the Nodes?
-
Reading Temps needs to be delayed without delay w/ Motion
Here is my sketch, so far it works but the temps read in decimals and fluctuate so much. How do I make the reads into integers and wait with out holding up motion status and switches? Thanks for the help!
https://codebender.cc/sketch:238531
Also can the 3 previosMillis and 3 MotionDelay be consolidated into 1 of each for all the motions or do each motion need it's own?
-
RE: SmartThings integration?
@blacey said:
A good friend of mine and talented engineer/architect recently purchased a SmartThings hub. Last weekend I ginned up an ESP8266/Node MCU gateway paired with a SenseBender Micro and gave it to him for SmartThings integration experimentation/plug-in development - I'm hopeful that something good will come of it
What else does he need! I just bought a SmartThings Hub
-
RE: Video How To - Phoney TV
@petewill
You are getting quite good at this Youtube thing, Very enjoyable and inspirational. Thanks again. -
RE: 110v-230v AC to Mysensors PCB board
@krizzziz
I have seen in some commercial wall switches thermal cutoff/fuses they are usually to the top of the offending (heat producing components) not necessarily directly touching. I have definitely added them to mine for peace of mind. Like this one:
http://i.imgur.com/rqOawGmm.jpgJust make sure you heat sink when soldering them into place and check them after installation.
-
RE: Multi Motion Detectors
Relay Pins not updating when switched locally any one know why this is in Vera UI5. I lose the On and Off status. The NODE is sending this :
35;2;1;0;2;1761607681 35;2;1;0;2;1761607680
So there is a problem with my code somewhere. I have another node that does this. Why?
The code is in the first post above.
Also where is there an example of a scene controller with regular buttons? Not using a library with a keypad.
-
RE: Multi Motion Detectors
@Dirk_H said:
message.sensor == 1
This was what I needed! Thanks
Now I have 3 Motion 2 switches, Now try to smash a DHT on here and fix the Vera reporting of changes made locally.
I updated the code above. -
Motion Sensor Pin 3 Only?
Can the motion sensor be used on other pins beside Pin 3 for the interrupt to work? I want to add 3 Motion sensors to 1 node.
Is there another way to write the code to use 3 Motion sensors?
Thanks
-
RE: Multi Motion Detectors
I need some help with this code, I can not find how to use 2 relays. I think I did it wrong here they are controlled in sync not separate. Also my motion is not working at all, is it the pin location for the interrupt? After this works I want 1 more Motion attached.
https://codebender.cc/sketch:178705
I Love this codebender.cc it is awesome!
-
Multi Motion Detectors
Is it possible to run multiple motion detectors like I have here? It compiles but will it work? I will build and test but I want to know if it looks legit. I'm trying to run a Dallas temp sensor also where do I put the reading and sleep code in the loop? or separate?
/** Based On: http://www.mysensors.org/build/relay Modded By DrJeff This Node is for 2 relays controlled remotely, and 1 Relay controlled locally via switch, 2 Motion detectors (possibly 3 motion) */ #include <MySensor.h> #include <SPI.h> #include <Bounce2.h> #define RELAY_1 4 // Arduino Digital I/O pin number for relay #define NUMBER_OF_RELAYS 1 // Total number of attached relays #define TOP_SWITCH 5 // Arduino Digital I/O pin number for button #define BOTTOM_SWITCH 6 // Arduino Digital I/O pin number for button #define CHILD_ID 1 // Id of the sensor child #define RELAY_ON 0 #define RELAY_OFF 1 // added motion #define Motion_1 3 #define Motion_2 7 #define INTERRUPT Motion_1-2 #define INTERRUPT Motion_2-2 uint8_t lastMotion_1 = 0; uint8_t lastMotion_2 = 0; #define MOTION_CHILD_1 2 #define MOTION_CHILD_2 3 #define NODE_ID 35 unsigned long previousMillis = 0; // last time update //see http://stackoverflow.com/questions/10773425/performing-a-function-after-x-time for more details on this unsigned long motionDelay = 10000; // interval at which to keep motion sensor trippped (milliseconds). Used to prevent too frequent updates to Vera. Bounce debouncer = Bounce(); int oldValue=0; bool state; MySensor gw; MyMessage msg(CHILD_ID,V_LIGHT); MyMessage motionStatus_1(MOTION_CHILD_1, V_TRIPPED); MyMessage motionStatus_2(MOTION_CHILD_2, V_TRIPPED); void setup() { gw.begin(incomingMessage, NODE_ID, true); // Send the sketch version information to the gateway and Controller gw.sendSketchInfo("Closet Node", "1.0"); // Setup the button pinMode(TOP_SWITCH,INPUT); pinMode(BOTTOM_SWITCH,INPUT); // Activate internal pull-up digitalWrite(TOP_SWITCH,HIGH); digitalWrite(BOTTOM_SWITCH,HIGH); // After setting up the button, setup debouncer debouncer.attach(TOP_SWITCH); debouncer.interval(5); debouncer.attach(BOTTOM_SWITCH); debouncer.interval(5); // Register all sensors to gw (they will be created as child devices) gw.present(CHILD_ID, S_LIGHT); gw.present(MOTION_CHILD_1, S_MOTION); gw.present(MOTION_CHILD_2, S_MOTION); // Make sure relays are off when starting up //digitalWrite(RELAY_1, RELAY_OFF); // Then set relay pins in output mode pinMode(RELAY_1, OUTPUT); pinMode(Motion_1, INPUT); pinMode(Motion_2, INPUT); // Set relay to last known state (using eeprom storage) //removed next 2 lines no like remember last state defaults to on then controller thinks its off 2015-08-19 JW state = gw.loadState(CHILD_ID); digitalWrite(RELAY_1, state?RELAY_ON:RELAY_OFF); } /* * Example on how to asynchronously check for new messages from gw */ void loop() { gw.process(); debouncer.update(); // Get the update value int value = debouncer.read(); if (value != oldValue && value==0) { gw.send(msg.set(state?false:true), true); // Send new state and request ack back } oldValue = value; } void incomingMessage(const MyMessage &message) { // We only expect one type of message from controller. But we better check anyway. if (message.isAck()) { Serial.println("This is an ack from gateway"); } if (message.type == V_LIGHT) { // Change relay state state = message.getBool(); digitalWrite(RELAY_1, state?RELAY_ON:RELAY_OFF); // Store state in eeprom gw.saveState(CHILD_ID, state); // Write some debug info Serial.print("Incoming change for sensor:"); Serial.print(message.sensor); Serial.print(", New status: "); Serial.println(message.getBool()); } // Read digital motion value For first motion detector unsigned long currentMillis = millis(); if(currentMillis - previousMillis > motionDelay){ uint8_t motionDetect = digitalRead(Motion_1); if(motionDetect != lastMotion_1){ // Serial.print("motionDetect Value: "); // Serial.println(motionDetect); //gw.send(motionStatus_1.set(motionDetect)); // Send tripped value to gw gw.send(motionStatus_1.set(motionDetect?"1":"0")); // Send tripped value to gw if(motionDetect == 1){ previousMillis = currentMillis; //"Tripped" delay } else{ previousMillis = currentMillis - motionDelay + 1000; //"Not tripped" delay for 1 second to stop rapid "not tripped" and "tripped" updates to Vera } lastMotion_1 = motionDetect; } } // Read digital motion value For second motion detector // unsigned long currentMillis = millis(); if(currentMillis - previousMillis > motionDelay){ uint8_t motionDetect = digitalRead(Motion_2); if(motionDetect != lastMotion_2){ // Serial.print("motionDetect Value: "); // Serial.println(motionDetect); //gw.send(motionStatus_2.set(motionDetect)); // Send tripped value to gw gw.send(motionStatus_2.set(motionDetect?"1":"0")); // Send tripped value to gw if(motionDetect == 1){ previousMillis = currentMillis; //"Tripped" delay } else{ previousMillis = currentMillis - motionDelay + 1000; //"Not tripped" delay for 1 second to stop rapid "not tripped" and "tripped" updates to Vera } lastMotion_2 = motionDetect; } } }
Any comments suggestions welcomed please.
-
RE: How To - Doorbell Automation Hack
@drock1985 said:
I'll end up putting in one of these I figure, just haven't quite figured out what size smoothing capacitor I will need.
Add a cap .1uf input side of the 5V regulator and a 47-220uf on the regulated 5V . High freq and a low freq ripple.
That should do the trick same thing I was planning on doing. I bought the Ring Doorbell but still want to be notified of the actual ring into HA.
-
Button Actuated relay with Motion
I'm trying to make a Relay with a Switch and Motion. Here is my sketch it works from the controler fine (Vera). The problem if I make changes at the switch the controller does not update. Also I can not get the motion to work. I put the motion inside of a void section. I also tried the motion code inside of the loop also. Any help?
// Example sketch för a "light switch" where you can control light or something // else from both vera and a local physical button (connected between digital // pin 4 and GND). // This node also works as a repeater for other nodes // Added Motion detection // Modified by DrJeff On 10/18/15 #include <MySensor.h> #include <SPI.h> #include <Bounce2.h> #define RELAY_PIN 3 // Arduino Digital I/O pin number for relay #define BUTTON_PIN 4 // Arduino Digital I/O pin number for button #define LIGHT_CHILD_ID 1 // Id of the sensor child #define RELAY_ON 0 #define RELAY_OFF 1 #define MOTION_SENSOR 5 // The digital input you attached your motion sensor. (Only 2 and 3 generates interrupt!) #define INTERRUPT MOTION_SENSOR-2 // Usually the interrupt = pin -2 (on uno/nano anyway) #define MOTION_LIGHT_CHILD_ID 2 // Id of the sensor child #define NODE_ID 4 uint8_t lastMotion = 0; unsigned long previousMillis = 0; // last time update //see http://stackoverflow.com/questions/10773425/performing-a-function-after-x-time for more details on this unsigned long motionDelay = 10000; // interval at which to keep motion sensor trippped (milliseconds). Used to prevent too frequent updates to Vera. Bounce debouncer = Bounce(); int oldValue=0; bool state; MySensor gw; MyMessage msgLIGHT(LIGHT_CHILD_ID,V_LIGHT); MyMessage msgMOTION(MOTION_LIGHT_CHILD_ID, V_TRIPPED); void setup() { gw.begin(incomingMessage, NODE_ID, true); // Send the sketch version information to the gateway and Controller gw.sendSketchInfo("Relay, Motion, & Button", "1.1"); //Motion pinMode(MOTION_SENSOR, INPUT); // sets the motion sensor digital pin as input // Register all sensors to gw (they will be created as child devices) gw.present(MOTION_LIGHT_CHILD_ID, S_MOTION); // Setup the button pinMode(BUTTON_PIN,INPUT); // Activate internal pull-up digitalWrite(BUTTON_PIN,HIGH); // After setting up the button, setup debouncer debouncer.attach(BUTTON_PIN); debouncer.interval(5); // Register all sensors to gw (they will be created as child devices) gw.present(LIGHT_CHILD_ID, S_LIGHT); // Make sure relays are off when starting up digitalWrite(RELAY_PIN, RELAY_OFF); // Then set relay pins in output mode pinMode(RELAY_PIN, OUTPUT); // Set relay to last known state (using eeprom storage) state = gw.loadState(LIGHT_CHILD_ID); digitalWrite(RELAY_PIN, state?RELAY_ON:RELAY_OFF); } /* * Example on how to asynchronously check for new messages from gw */ void loop() { gw.process(); debouncer.update(); // Get the update value int value = debouncer.read(); if (value != oldValue && value==0) { gw.send(msgLIGHT.set(state?false:true), true); // Send new state and request ack back } oldValue = value; } void MotionStatus (){ // Read digital motion value unsigned long currentMillis = millis(); if(currentMillis - previousMillis > motionDelay){ uint8_t motionDetect = digitalRead(MOTION_SENSOR); if(motionDetect != lastMotion){ gw.send(msgMOTION.set(motionDetect?"1":"0")); if(motionDetect == 1){ previousMillis = currentMillis; } else{ previousMillis = currentMillis - motionDelay + 1000; } lastMotion = motionDetect; } } } void incomingMessage(const MyMessage &message) { // We only expect one type of message from controller. But we better check anyway. if (message.isAck()) { Serial.println("This is an ack from gateway"); } if (message.type == V_LIGHT) { // Change relay state state = message.getBool(); digitalWrite(RELAY_PIN, state?RELAY_ON:RELAY_OFF); // Store state in eeprom gw.saveState(LIGHT_CHILD_ID, state); // Write some debug info Serial.print("Incoming change for sensor:"); Serial.print(message.sensor); Serial.print(", New status: "); Serial.println(message.getBool()); } }
I'm sure I'm doing something wrong just not sure what and were. Thanks for the help.
-
RE: How To: Make a Simple/Cheap Scene Controller (with video)
@Fred-LaR WOW!
You just perked up my intrest got to try this one! -
RE: How To - Doorbell Automation Hack
@TD22057 This was the route I was heading with adding the doorbell to my HA, but @petewill is awesome in doing this with the video. Me and my daughter where just trying to decide what would be the best way to hack the bell. We added a Ring.com Doorbell (trial period) and trying to decide if we like it or not. The notifications are 2 way so that is nice with video and audio. But they do not have an API. I think I will try to sniff out the Motion detection or use it from my notifications to tie into my HA.
-
RE: 110v-230v AC to Mysensors PCB board
@bjornhallberg said:
Maybe we could find some sort of small fuse holder instead?
I know its probably not the best thing to do but I have just soldered on leads, bare wire. I just tin the metal first and scuff it a little. I have done the same thing to batteries with no ill effect.The key is get in and off quickly!
-
RE: 110v-230v AC to Mysensors PCB board
@bjornhallberg said:
was out of stock on Ebay. As was the 0.2A version. Someone is stocking up
oops! You wanted some?
just kidding, let me check I have a line on parts I will see if they have some.
-
RE: Roller Shutter
@scalz
I'm following this post closely I like the idea, I have some motors controlled via RF but I don't like the controllers or the reliability. I will see how to retrofit them with your controller after you have tested and did all the hard work!I believe I can just Hijack the motor wires and use the relays and the boards for all the logic. I like what you have setup but I will have to see your sketch first.
I have some old Hunter Douglass motorized shutters that use IR remote I hacked them with an reed relay on a node that acts as a push button. Just like pushing the button on the motor. Unfortunately the new shutters I got don't have a manual push button or I would do the same.
-
RE: 110v-230v AC to Mysensors PCB board
@Yveaux
I believe those are for the serial connection that usually are pointing up, or back for programing? It looks like they are just rendered as down in the 3D image? But I am curious how the clearance for the components on the other side (HLK) is when they are soldered, does it need some space off the board? -
RE: How To: Make a Simple/Cheap Scene Controller (with video)
Another stellar video @petewill ! Thanks! Now time to 3D print a box and add batteries for your night stand.
-
RE: Pool Pressure Sensor
@Dwalt
Yes I plan on monitoring pressure rises. I assumed the accuracy would not be perfect, I'm not concerned with perfection just changes. After I set a baseline then the automation begins. Currently I use a spreadsheet to keep track of when the filter is cleaned (reading the mechanical gauge PSI) and that is missed sometimes manual entering data is not my forte. Then after checking the gauge to see increase in pressure I know it's time to clean the filter. If I wrote the data into the spreadsheet then I know how long till it needs it again, well sort of. The automation part will be sending alerts when necessary action is required not some spreadsheet Voodoo even though adding the data automatically to the spreadsheet will be nice. I already have the rest of the pool automated Lights, Pumps(5), Pump speeds, Valves for Solar, Waterfalls, spill overs, water fill lines, and the list continues. I even have some temp sensors and hysteresis for gas heater and solar heating. All this currently runs on an Arduino with a serial connection via XBee into my Mac with XTension Home Automation Software from http://machomeautomation.com. I have been rallying James over there to add XTension as controller for MySensors and I think he is almost ready to do it!
psiRead = map(psiRead, 102, 921, 0, 100);
I think I get how this works so I will be trying to implement this. what would be the advantage to the code above and this way?
On sending this just as a number I think you are smart about that, for now it goes to Vera but hopefully soon XTension. -
RE: Safe In-Wall AC to DC Transformers??
@ceech can't look at the files right now is this a dimmer switch that is transformerless?
-
Pool Pressure Sensor
Ok Time to build a sensor to measure my pool filter pressure. This is what I will be using.
http://www.amazon.com/dp/B00RCPDPRQConnect Pressure Transducer to filter with a little plumbing.
Now time to make the board and set the code. I need a little help with the code I have some sample code I found online here.
void loop() { float sensorVoltage = analogRead(0); // read the sensor voltage int psi = ((sensorVoltage-95)/204)*50; //change to *25 for 100PSI Transducer I think Serial.println (psi); delay (1000); }
I think the Transducer I have is 25 psi per 1V.
But how to send
Serial.println (psi);
into MySensors I need the help that, type of unit? Same as the barometer?Thanks in advance for any help!
-
RE: ESP8266 WiFi gateway port for MySensors
@hek Yes I tried those but it's probably the driver install it was acting a little finicky so it was quicker to flip over to the VM.
-
RE: ESP8266 WiFi gateway port for MySensors
Built Mine
works great the only problem I had was uploading the code from a Mac OSX Mavericks so I opened a Win7 VM I keep for these type of problems and all is good. Thanks! @Yveaux
My Gateway has shrunk...
-
RE: ESP8266 WiFi gateway port for MySensors
Can this be built with esp8266-03? I got a couple of those sitting here waiting to get used? It looks like I can swap Pin 4 for Pin 16 (or 18 in this picture) Is there something I'm missing?
-
RE: RGB-3D only showing 1 dimmer, not 3 in Vera
@timropp Also remember if your gateway is using a long range antenna make sure it has enough power I have had that problem also. You may get some inclusion but not others.
-
RE: ESP8266 WiFi gateway port for MySensors
@Yveaux said:
@DrJeff said:
@Yveaux No you did not scare me away
A, damn ! Maybe next project then?
The problem lies mainly in the vast amount of ESP variants and how to wire them to be able to download and to connect the radio.
Sorry it was supposed to be "No you didn't scare", me auto correct!
I'm going to build this for sure!
-
RE: ESP8266 WiFi gateway port for MySensors
@Yveaux No you did not scare me away but I'm in loss of time to do this. I look forward to setting my esp gateway up in a week or so.
-
Serial Monitor of the gateway shows change but no change on the vera
On the serial monitor output I see motion being changed. But I can not see the change being made on the vera.
send: 31-31-0-0 s=255,c=3,t=11,pt=0,l=16,sg=0,st=ok:RGB Led strip 3D send: 31-31-0-0 s=255,c=3,t=12,pt=0,l=2,sg=0,st=ok:v1 send: 31-31-0-0 s=6,c=0,t=4,pt=0,l=17,sg=0,st=ok:present RED light send: 31-31-0-0 s=4,c=0,t=4,pt=0,l=19,sg=0,st=ok:present GREEN light send: 31-31-0-0 s=5,c=0,t=4,pt=0,l=18,sg=0,st=ok:present BLUE light send: 31-31-0-0 s=0,c=0,t=3,pt=0,l=19,sg=0,st=ok:present Show button send: 31-31-0-0 s=3,c=0,t=1,pt=0,l=14,sg=0,st=ok:present Motion Load from eeprom RED: 0% Load from eeprom GREEN: 0% Load from eeprom BLUE: 0% Send eeprom value to controler send: 31-31-0-0 s=6,c=1,t=3,pt=1,l=1,sg=0,st=ok:0 send: 31-31-0-0 s=4,c=1,t=3,pt=1,l=1,sg=0,st=ok:0 send: 31-31-0-0 s=5,c=1,t=3,pt=1,l=1,sg=0,st=ok:0 send: 31-31-0-0 s=0,c=1,t=2,pt=2,l=2,sg=0,st=ok:0 Ready to receive messages... send: 31-31-0-0 s=3,c=1,t=1,pt=1,l=1,sg=0,st=ok:1 send: 31-31-0-0 s=3,c=1,t=1,pt=1,l=1,sg=0,st=ok:0 send: 31-31-0-0 s=3,c=1,t=1,pt=1,l=1,sg=0,st=ok:1 send: 31-31-0-0 s=3,c=1,t=1,pt=1,l=1,sg=0,st=ok:0 send: 31-31-0-0 s=3,c=1,t=1,pt=1,l=1,sg=0,st=ok:1 send: 31-31-0-0 s=3,c=1,t=1,pt=1,l=1,sg=0,st=ok:0
As seen after Ready to receive messages... that is the motion sensor working but in Vera it shows no difference here is the code used it is a mix of @hek RGB code mixed with a motion sensor. The idea of the motion sensor is just to report if there is motion. It is a presence sensor for the room if I want it to turn on the the LED it will be scripted separate from the arduino code.
/** * The MySensors Arduino library handles the wireless radio link and protocol * between your home built sensors/actuators and HA controller of choice. * The sensors forms a self healing radio network with optional repeaters. Each * repeater and gateway builds a routing tables in EEPROM which keeps track of the * network topology allowing messages to be routed to nodes. * * Created by Henrik Ekblad <henrik.ekblad@mysensors.org> * Copyright (C) 2013-2015 Sensnology AB * Full contributor list: https://github.com/mysensors/Arduino/graphs/contributors * * Documentation: http://www.mysensors.org * Support Forum: http://forum.mysensors.org * * This program is free software; you can redistribute it and/or * modify it under the terms of the GNU General Public License * version 2 as published by the Free Software Foundation. * ******************************* * * REVISION HISTORY * Version 1.0 - Created by vil1driver * * DESCRIPTION * RGB led strip controled with three dimmers + one On/Off for run/stop rgb color cycle :p * */ #define SN "RGB Led strip 3D" #define SV "v1" // Load mysensors library #include <MySensor.h> // Load Serial Peripheral Interface library #include <SPI.h> // Arduino pin attached to MOSFET Gate pin #define RED_PIN 4 #define GREEN_PIN 5 #define BLUE_PIN 6 // added motion #define Motion_PIN 3 #define INTERRUPT Motion_PIN-2 uint8_t lastMotion = 0; unsigned long previousMillis = 0; // last time update //see http://stackoverflow.com/questions/10773425/performing-a-function-after-x-time for more details on this unsigned long motionDelay = 10000; // interval at which to keep motion sensor trippped (milliseconds). Used to prevent too frequent updates to Vera. MySensor gw; // Define message name and type to send sensor info MyMessage RedStatus(RED_PIN, V_DIMMER); MyMessage GreenStatus(GREEN_PIN, V_DIMMER); MyMessage BlueStatus(BLUE_PIN, V_DIMMER); MyMessage Status(1, V_DIMMER); MyMessage rgbShowState(0, V_LIGHT); MyMessage MotionStatus(Motion_PIN, S_MOTION); // Serial.print translate sensor id to sensor name char color[][6] = {"","","","RED","","GREEN","BLUE"}; // Vars for rgbShow function int redval = 0; int greenval = 0; int blueval = 0; long time=0; int isShow; void setup() { //motion setup //pinMode(Motion_PIN, INPUT); // Initializes the sensor node (Callback function for incoming messages, node id, is repeater) gw.begin(incomingMessage, AUTO, true); // Present sketch (name, version) gw.sendSketchInfo(SN, SV); // Register sensors (id, type, description, ack back) gw.present(RED_PIN, S_DIMMER, "present RED light", false); gw.present(GREEN_PIN, S_DIMMER, "present GREEN light", false); gw.present(BLUE_PIN, S_DIMMER, "present BLUE light", false); gw.present(0, S_LIGHT, "present Show button", false); // motion present gw.present(Motion_PIN, S_MOTION, "present Motion", false); // Define pin mode (pin number, type) pinMode(RED_PIN, OUTPUT); pinMode(GREEN_PIN, OUTPUT); pinMode(BLUE_PIN, OUTPUT); pinMode(Motion_PIN, INPUT); // Correct saved RGB value for first start gw.saveState(RED_PIN, constrain((int8_t)gw.loadState(RED_PIN), 0, 100)); gw.saveState(GREEN_PIN, constrain((int8_t)gw.loadState(GREEN_PIN), 0, 100)); gw.saveState(BLUE_PIN, constrain((int8_t)gw.loadState(BLUE_PIN), 0, 100)); // Get value from eeprom and write to output analogWrite(RED_PIN, 255 * gw.loadState(RED_PIN) / 100); analogWrite(GREEN_PIN, 255 * gw.loadState(GREEN_PIN) / 100); analogWrite(BLUE_PIN, 255 * gw.loadState(BLUE_PIN) / 100); // Write some debug info Serial.print("Load from eeprom RED: "); Serial.print(gw.loadState(RED_PIN)); Serial.println("%"); Serial.print("Load from eeprom GREEN: "); Serial.print(gw.loadState(GREEN_PIN)); Serial.println("%"); Serial.print("Load from eeprom BLUE: "); Serial.print(gw.loadState(BLUE_PIN)); Serial.println("%"); // Send RGB value to controler (request ack back: true/false) Serial.println("Send eeprom value to controler"); gw.send( RedStatus.set(gw.loadState(RED_PIN)), false ); gw.send( GreenStatus.set(gw.loadState(GREEN_PIN)), false ); gw.send( BlueStatus.set(gw.loadState(BLUE_PIN)), false ); // Correct RGB show state for first start and load it (set to 'On' at first start) gw.saveState(0, constrain((int8_t)gw.loadState(0), 0, 1)); isShow=gw.loadState(0); // Send RGB show state to controler (request ack back: true/false) gw.send( rgbShowState.set(isShow), false); if (isShow==1){Serial.println("RGB show running..."); } Serial.println("Ready to receive messages..."); } void loop() { // Process incoming messages (like config and light state from controller) gw.process(); // Run RGB show if is set if (isShow==1) { rgbShow(); analogWrite(RED_PIN, redval); analogWrite(GREEN_PIN, greenval); analogWrite(BLUE_PIN, blueval); } // Read digital motion value unsigned long currentMillis = millis(); if(currentMillis - previousMillis > motionDelay){ uint8_t motionDetect = digitalRead(Motion_PIN); if(motionDetect != lastMotion){ // Serial.print("motionDetect Value: "); // Serial.println(motionDetect); gw.send(MotionStatus.set(motionDetect)); // Send tripped value to gw if(motionDetect == 1){ previousMillis = currentMillis; //"Tripped" delay } else{ previousMillis = currentMillis - motionDelay + 1000; //"Not tripped" delay for 1 second to stop rapid "not tripped" and "tripped" updates to Vera } lastMotion = motionDetect; } } } void incomingMessage(const MyMessage &message) { if (message.isAck()) { Serial.println("Got ack from gateway"); } if (message.type == V_LIGHT) { // Incoming on/off command sent from controller ("1" or "0") int lightState = message.getString()[0] == '1'; // if receive RGB Show On commands, start the show if (message.sensor==0 && lightState==1){ rgbShowOn(); } // if receive RGB Show Off commands, stop the show else if (message.sensor==0 && lightState==0){ rgbShowOff(); } // if receive RGB switch On command else if (lightState==1) { // Write some debug info Serial.print("Incoming change for "); Serial.print(color[message.sensor]); Serial.println(": On"); Serial.print("Load from eeprom: "); if ( gw.loadState(message.sensor) == 0) { // Pick up last saved dimmer level from the eeprom analogWrite(message.sensor, 255 * gw.loadState(10*message.sensor) / 100); // Save loaded value to current gw.saveState(message.sensor, gw.loadState(10*message.sensor)); Serial.print(gw.loadState(10*message.sensor)); Serial.println("%"); // Send value to controler Serial.println("Send value to controler"); gw.send(Status.setSensor(message.sensor).set(gw.loadState(10*message.sensor)),false); } else { // Pick up last saved dimmer level from the eeprom analogWrite(message.sensor, 255 * gw.loadState(message.sensor) / 100); Serial.print(gw.loadState(message.sensor)); Serial.println("%"); // Send value to controler Serial.println("Send value to controler"); gw.send(Status.setSensor(message.sensor).set(gw.loadState(message.sensor)),false); } // Stop the show if it's running if (isShow==1){ rgbShowStop(message.sensor); } } // if recieve switch Off command else if (lightState==0) { // Write output to 0 (Off) analogWrite(message.sensor, 0); // Save old value to eeprom if it'was not zero if ( gw.loadState(message.sensor) != 0 ) { gw.saveState(10*message.sensor, constrain((int8_t)gw.loadState(message.sensor), 0, 100)); } // Save new value to eeprom gw.saveState(message.sensor, 0); // Write some debug info Serial.print("Incoming change for "); Serial.print(color[message.sensor]); Serial.print(": "); Serial.println("Off"); Serial.print("Store old value: "); Serial.print(gw.loadState(10*message.sensor)); Serial.println("%"); // Send value to controler Serial.println("Send value to controler"); gw.send(Status.setSensor(message.sensor).set(gw.loadState(message.sensor)),false); // Stop the show if it's running if (isShow==1){ rgbShowStop(message.sensor); } } } else if (message.type == V_DIMMER) { uint8_t incomingDimmerStatus = message.getByte(); // limits range of sensor values to between 0 and 100 incomingDimmerStatus = constrain((int8_t)incomingDimmerStatus, 0, 100); // Change Dimmer level analogWrite(message.sensor, 255 * incomingDimmerStatus / 100); //Save value to eeprom gw.saveState(message.sensor, incomingDimmerStatus); // Write some debug info Serial.print("Incoming change for "); Serial.print(color[message.sensor]); Serial.print(": "); Serial.print(incomingDimmerStatus); Serial.println("%"); // Send value to controler Serial.println("Send value to controler"); gw.send(Status.setSensor(message.sensor).set(gw.loadState(message.sensor)),false); // Stop the show if it's running if (isShow==1){ rgbShowStop(message.sensor); } } } void rgbShow() { time = millis(); redval = 128+250*cos(2*PI/300000*time); greenval = 128+250*cos(2*PI/300000*time-222); blueval = 128+250*cos(2*PI/300000*time-111); // limits range of sensor values to between 0 and 255 redval = constrain(redval, 0, 255); greenval = constrain(greenval, 0, 255); blueval = constrain(blueval, 0, 255); } void rgbShowOn() { // define show On isShow=1; // Save state gw.saveState(0, 1); // Write some debug info Serial.println("Show must go on"); } void rgbShowOff() { // define show Off isShow=0; // Save state gw.saveState(0, 0); // Save RGB value to eeprom gw.saveState(RED_PIN, 100 * redval / 255); gw.saveState(GREEN_PIN, 100 * greenval / 255); gw.saveState(BLUE_PIN, 100 * blueval / 255); // Write some debug info Serial.println("Stop the show"); // Send actual RGB value and state to controler and request ack back (true/false) Serial.println("Send eeprom value to controler"); gw.send( RedStatus.set(gw.loadState(RED_PIN)), false ); gw.send( GreenStatus.set(gw.loadState(GREEN_PIN)), false ); gw.send( BlueStatus.set(gw.loadState(BLUE_PIN)), false ); gw.send( rgbShowState.set(0), false); } void rgbShowStop(int sensor) { // define show Off isShow=0; // Save state gw.saveState(0, 0); // Write some debug info Serial.println("Stop the show"); // Send actual RGB value and state to controler and request ack back (true/false) Serial.println("Send eeprom value to controler"); if (sensor != RED_PIN) { gw.saveState(RED_PIN, 100 * redval / 255); gw.send( RedStatus.set(gw.loadState(RED_PIN)), false ); } if (sensor != GREEN_PIN) { gw.saveState(GREEN_PIN, 100 * greenval / 255); gw.send( GreenStatus.set(gw.loadState(GREEN_PIN)), false ); } if (sensor != BLUE_PIN) { gw.saveState(BLUE_PIN, 100 * blueval / 255); gw.send( BlueStatus.set(gw.loadState(BLUE_PIN)), false ); } gw.send( rgbShowState.set(0), false); } /* void motion() { // Read digital motion value boolean tripped = digitalRead(Motion_PIN) == HIGH; Serial.println(tripped); gw.send(MotionStatus.set(tripped?"1":"0")); // Send tripped value to gw // Sleep until interrupt comes in on motion sensor. Send update every two minute. gw.sleep(INTERRUPT,CHANGE, SLEEP_TIME); } */
Any Ideas of where I'm going wrong?
-
RE: Update the Vera Plugin??
Ok Solved my gateway is an Ethernet gateway and for debugging I was using USB to power the radio was too underpowered to function properly. Now Inclusion is working!
-
RE: Update the Vera Plugin??
@korttoma Thanks,
was trying to get a RGB light working but thought maybe it was the plugin I guess not, I will keep searching. -
Update the Vera Plugin??
How is the plugin updated I installed the old files on top of the existing. Do I need to delete the files first? I still see the plugin as version 1.4 and the Lib Version as 1.5.
Or am I doing this all wrong? -
RE: ESP8266 WiFi gateway port for MySensors
@Yveaux said:
diff of the files should help a lot: https://www.diffchecker.com/eeo5ahzn).
Thanks for the heads up on this service Diff checker I didn't know of this site I will be using it! Thanks
-
RE: Safe In-Wall AC to DC Transformers??
@ceech Yes I like the idea of course the board would be housed inside of a box, my idea is to incorporate the box with a daughter board with 2 push buttons on and off like Zwave switches use. Yes please send me, if you are worried I will use safely I was taught to work on all power 120v - 480v as if it is live so that you remain alive!
-
RE: Safe In-Wall AC to DC Transformers??
So basically it is like any other consumer Zwave switch or X10 just box it up (3D printer on stand by) with 2 micro buttons with a rocker face and I want 10.
But sorry I digressed from the OP in my excitement.
-
RE: Safe In-Wall AC to DC Transformers??
@ceech said:
Yes, it shouldn't be touched
Nice! just label board !LIVE!
Not safe due to no fuse? Do share, making your own boards with solder mask?
-
RE: My upcoming project
Can you integrate this into my Toto washlet so that it can turbo wash if I have been there to long!
Luv it!
-
RE: 110v-230v AC to Mysensors PCB board
@Oitzu
I seem to get all this working outside of MYSensors but I have very limited cut and paste ability in code.So what I was wondering do you have the sketch for controlling the dimmer and just firing the triac integrated with MYSensors yet?
-
RE: 110v-230v AC to Mysensors PCB board
@Oitzu said:
my badly soldered dimmer.
No way that is just like mine!
It's Perfect!
Just Kidding I need to get my dimmers working properly also. You got the zerocross working right? 220v 110v? share sketch? will yours work locally with gateway off?
-
RE: Relay with actuator standalone functionality with no Gateway
@BulldogLowell Thanks I will give it a try!
-
Relay with actuator standalone functionality with no Gateway
In the sample code for the actuator and relay how hard is it to make the code work even if the gateway is off. That is the button/switch is still controlling the load locally. Optimally I want the code to handle a switch that is just toggling the relay even if the remote controller has changed the status of the light. The Idea is that locally the switch will still just function like a normal switch if the gateway is lost, or down.
/** * The MySensors Arduino library handles the wireless radio link and protocol * between your home built sensors/actuators and HA controller of choice. * The sensors forms a self healing radio network with optional repeaters. Each * repeater and gateway builds a routing tables in EEPROM which keeps track of the * network topology allowing messages to be routed to nodes. * * Created by Henrik Ekblad <henrik.ekblad@mysensors.org> * Copyright (C) 2013-2015 Sensnology AB * Full contributor list: https://github.com/mysensors/Arduino/graphs/contributors * * Documentation: http://www.mysensors.org * Support Forum: http://forum.mysensors.org * * This program is free software; you can redistribute it and/or * modify it under the terms of the GNU General Public License * version 2 as published by the Free Software Foundation. * ******************************* * * REVISION HISTORY * Version 1.0 - Henrik Ekblad * * DESCRIPTION * Example sketch for a "light switch" where you can control light or something * else from both HA controller and a local physical button * (connected between digital pin 3 and GND). * This node also works as a repeader for other nodes * http://www.mysensors.org/build/relay */ #include <MySensor.h> #include <SPI.h> #include <Bounce2.h> #define RELAY_PIN 4 // Arduino Digital I/O pin number for relay #define BUTTON_PIN 3 // Arduino Digital I/O pin number for button #define CHILD_ID 1 // Id of the sensor child #define RELAY_ON 1 #define RELAY_OFF 0 Bounce debouncer = Bounce(); int oldValue=0; bool state; MySensor gw; MyMessage msg(CHILD_ID,V_LIGHT); void setup() { gw.begin(incomingMessage, AUTO, true); // Send the sketch version information to the gateway and Controller gw.sendSketchInfo("Relay & Button", "1.0"); // Setup the button pinMode(BUTTON_PIN,INPUT); // Activate internal pull-up digitalWrite(BUTTON_PIN,HIGH); // After setting up the button, setup debouncer debouncer.attach(BUTTON_PIN); debouncer.interval(5); // Register all sensors to gw (they will be created as child devices) gw.present(CHILD_ID, S_LIGHT); // Make sure relays are off when starting up digitalWrite(RELAY_PIN, RELAY_OFF); // Then set relay pins in output mode pinMode(RELAY_PIN, OUTPUT); // Set relay to last known state (using eeprom storage) state = gw.loadState(CHILD_ID); digitalWrite(RELAY_PIN, state?RELAY_ON:RELAY_OFF); } /* * Example on how to asynchronously check for new messages from gw */ void loop() { gw.process(); debouncer.update(); // Get the update value int value = debouncer.read(); if (value != oldValue && value==0) { gw.send(msg.set(state?false:true), true); // Send new state and request ack back } oldValue = value; } void incomingMessage(const MyMessage &message) { // We only expect one type of message from controller. But we better check anyway. if (message.isAck()) { Serial.println("This is an ack from gateway"); } if (message.type == V_LIGHT) { // Change relay state state = message.getBool(); digitalWrite(RELAY_PIN, state?RELAY_ON:RELAY_OFF); // Store state in eeprom gw.saveState(CHILD_ID, state); // Write some debug info Serial.print("Incoming change for sensor:"); Serial.print(message.sensor); Serial.print(", New status: "); Serial.println(message.getBool()); } }
-
RE: Video How To - Monitor your Smoke/Carbon Monoxide Alarm
@petewill Thanks for another Video and also the variation to the smoke detector setup I have 3 smoke detectors laying around (from the store) and I was trying to figure out how to tap them for there signal. (working on hardwired ones, I already have the wireless unit done Thanks to @ServiceXp). The use of the Interconnect was on my mind but too many projects and tooooo busy at work so thanks! I also love the use of the pulse signal to get the Co2 separate brilliant.
-
RE: Parking Sensor
@hek Cool I figured you would come up with a practicle use for that LED ring!
-
RE: my first weather station
@Andy-Pep said:
yes its going to be a learning curve ..but after finding mysensors my mind has woken up
Got to love Sites like this one that challenge your brain and you have fun with physical items to show what you have been doing!
-
RE: Safe In-Wall AC to DC Transformers??
@rvendrame I'm right with you on all of this have been trying to do this for a couple months with no time right now. Actually it has been longer I was originally building all this for another system using XBees and then found it better to use esp8266 then said better yet use Mysensors so here we are! Transformer less is key at least I think so! Only problem no off the self builds.
-
RE: Safe In-Wall AC to DC Transformers??
I'm seriously thinking it skipping all of these and build a transformerless circuit directly to the board like a true in wall switch, Pete do you want to share this project. I've been playing with this for a little while but haven't had time to put it all together. I can point you to all the places but a board will need to be designed for the switch, that's almost where I'm at!
-
RE: sensors in boxes
@GuyP Yes we always got to deal with real life! On the board I assume the red lines are wires due to the single sided board?
-
RE: sensors in boxes
@GuyP Cool thanks, Time to etch some boards, have you used this method/setup for other nodes? Just trying to get some ideas
-
RE: sensors in boxes
@GuyP Can you post the pdf images of the boards, I want to etch some I like the use of 328P chip, The only problem the png file is scaled wrong too large.
-
RE: How to contribute code to the MySensors project
@hek said:
This is where the project started: http://forum.micasaverde.com/index.php/topic,16170.0.html
WOW your vision stayed the same! Cool
-
RE: MySensors Sensebender Micro 3DP Case
Nice! What is your print time? What printer do you have?
-
RE: Trying to do a 3 dimmer Led controller with motion
@rvendrame Do share your circuit I would love to compare. I found the inmojo circuit to work well. My only missing piece is I want to power the circuit with s tranformerless power supply.
-
RE: Trying to do a 3 dimmer Led controller with motion
@rvendrame said:
3 dimmers (using timers). If you implement timers, you can use any pin. (and it also works with AC-Triacs --- Be careful!!!) .
What is the caution for? Just that I'm working with 120V or is there something I'm Missing here? Will it go up in flames based on the timer circuit?
I love the way you did this I was trying to do the A/C dimmer (asked in another thread), The hardware was pretty simple but the code was sooooo hard to figure out I will give this a shot! I will also use this with my LED dimmer you have fixed two of my problems with, wait three you got IR in here also! Yippe!
You Rock! @rvendrame
-
RE: Irrigation Controller (up to 16 valves with Shift Registers)
@tbowmo Time for a Shower, Can you say body sprays!
-
Trying to do a 3 dimmer Led controller with motion
This is based on the main page of the builds dimmable LED but I want to use 3 Dimmers for RGB LED strip. All on full brightness equalls white! But the code is giving me some trouble this is what I have so far but only 1 Dimmer and Motion show up in my Vera, the 2 other dimmers are not showing or working any help would be nice.
/*** * This program is free software; you can redistribute it and/or * modify it under the terms of the GNU General Public License * version 2 as published by the Free Software Foundation. * * DESCRIPTION * This sketch provides a 3 Color Dimmable LED Light using PWM and based Henrik Ekblad * <henrik.ekblad@gmail.com> Vera Arduino Sensor project. * And Based on Bruce Lacey, inspired by Hek's MySensor's example sketches. * * The circuit uses a MOSFET for Pulse-Wave-Modulation to dim the attached LED or LED strip. * The MOSFET Gate pin is connected to Arduino pin 3,5,6 (LED_PIN), the MOSFET Drain pin is connected * to the LED negative terminal and the MOSFET Source pin is connected to ground. * * * REVISION HISTORY * Version 1.0 - February 15, 2014 - Bruce Lacey * Version 1.1 - August 13, 2014 - Converted to 1.4 (hek) * Version 1.2 - Hacked up by Jeff Wooding (DrJeff) * ***/ #define SN "DimmableColorLED" #define SV "1.2" #define NODE_ID AUTO //change to a number to assign a specific ID #include <MySensor.h> #include <SPI.h> #define RLED_PIN_CHILD 0 //ID of the RED LED child #define GLED_PIN_CHILD 1 //ID of the GREEN LED child #define BLED_PIN_CHILD 2 //ID of the BLUE LED child #define MOTION_CHILD 3 //ID of the MOTION child #define RLED_PIN 3 // Arduino pin attached to MOSFET Gate pin #define GLED_PIN 5 // Arduino pin attached to MOSFET Gate pin #define BLED_PIN 6 // Arduino pin attached to MOSFET Gate pin #define MOTION_PIN 4 // Arduino pin tied to trigger pin on the motion sensor. #define FADE_DELAY 10 // Delay in ms for each percentage fade up/down (10ms = 1s full-range dim) //MySensor gw(0,9); MySensor gw; //Don't need to define pins unless they are changing from the default static int currentLevel = 0; // Current dim level... MyMessage dimmerMsg(0, V_DIMMER); MyMessage lightMsg(0, V_LIGHT); //added 1,2 MyMessage dimmerMsg1(1, V_DIMMER); MyMessage lightMsg1(1, V_LIGHT); MyMessage dimmerMsg2(2, V_DIMMER); MyMessage lightMsg2(2, V_LIGHT); //motion sensor MyMessage motionMsg(MOTION_CHILD, V_TRIPPED); uint8_t lastMotion = 0; unsigned long previousMillis = 0; // last time update //see http://stackoverflow.com/questions/10773425/performing-a-function-after-x-time for more details on this unsigned long motionDelay = 10000; // interval at which to keep motion sensor trippped (milliseconds). Used to prevent too frequent updates to Vera. boolean metric = true; /*** * Dimmable LED initialization method */ void setup() { Serial.println( SN ); gw.begin( incomingMessage ); // Register the LED Dimmable Light with the gateway gw.present( RLED_PIN_CHILD, S_DIMMER ); gw.present( GLED_PIN_CHILD, S_DIMMER ); gw.present( BLED_PIN_CHILD, S_DIMMER ); gw.present( MOTION_CHILD, S_MOTION ); gw.sendSketchInfo(SN, SV); metric = gw.getConfig().isMetric; // Pull the gateway's current dim level - restore light level upon sendor node power-up gw.request( RLED_PIN_CHILD, V_DIMMER ); gw.request( GLED_PIN_CHILD, V_DIMMER ); gw.request( BLED_PIN_CHILD, V_DIMMER ); } /*** * Dimmable LED main processing loop */ void loop() { gw.process(); //motion sensor code unsigned long currentMillis = millis(); if(currentMillis - previousMillis > motionDelay){ uint8_t motionDetect = digitalRead(MOTION_PIN); if(motionDetect != lastMotion){ // Serial.print("motionDetect Value: "); // Serial.println(motionDetect); gw.send(motionMsg.set(motionDetect)); // Send tripped value to gw if(motionDetect == 1){ previousMillis = currentMillis; //"Tripped" delay } else{ previousMillis = currentMillis - motionDelay + 1000; //"Not tripped" delay for 1 second to stop rapid "not tripped" and "tripped" updates to Vera } lastMotion = motionDetect; } } } /*** * This method provides a graceful fade up/down effect */ void fadeToLevel( int toLevel ) { int delta = ( toLevel - currentLevel ) < 0 ? -1 : 1; while ( currentLevel != toLevel ) { currentLevel += delta; analogWrite( RLED_PIN, (int)(currentLevel / 100. * 255) ); delay( FADE_DELAY ); } while ( currentLevel != toLevel ) { currentLevel += delta; analogWrite( GLED_PIN, (int)(currentLevel / 100. * 255) ); delay( FADE_DELAY ); } while ( currentLevel != toLevel ) { currentLevel += delta; analogWrite( BLED_PIN, (int)(currentLevel / 100. * 255) ); delay( FADE_DELAY ); } } void incomingMessage(const MyMessage &message) { if (message.type == V_LIGHT || message.type == V_DIMMER) { // Retrieve the power or dim level from the incoming request message int requestedLevel = atoi( message.data ); // Adjust incoming level if this is a V_LIGHT variable update [0 == off, 1 == on] requestedLevel *= ( message.type == V_LIGHT ? 100 : 1 ); // Clip incoming level to valid range of 0 to 100 requestedLevel = requestedLevel > 100 ? 100 : requestedLevel; requestedLevel = requestedLevel < 0 ? 0 : requestedLevel; Serial.print( "Changing level to " ); Serial.print( requestedLevel ); Serial.print( ", from " ); Serial.println( currentLevel ); fadeToLevel( requestedLevel ); // Inform the gateway of the current DimmableLED's SwitchPower1 and LoadLevelStatus value... gw.send(lightMsg.set(currentLevel > 0 ? 1 : 0)); //added gw.send(lightMsg1.set(currentLevel > 0 ? 1 : 0)); gw.send(lightMsg2.set(currentLevel > 0 ? 1 : 0)); // hek comment: Is this really nessesary? gw.send( dimmerMsg.set(currentLevel) ); //added gw.send( dimmerMsg1.set(currentLevel) ); gw.send( dimmerMsg2.set(currentLevel) ); } }
I would also like to add a Rotary encoder to this but I think I'm tapped out on the PWM pins to make it work. Any Ideas? Another thought would be to use a separate board/pro mini and dim it remotely?
-
RE: Irrigation Controller (up to 16 valves with Shift Registers)
@BulldogLowell Sorry For not giving the credit where it is due! Major Props @BulldogLowell !
-
RE: Irrigation Controller (up to 16 valves with Shift Registers)
@Sparkman I like the sensors, I just built some and was dbating the way to make smaller, what kind of battery life do those moisture sensors have?
@petewill Time to change my current 16 valve arduino sprinklers to a little smarter version like yours! I love this community.
You have been coming on with some awesome videos and contributions! -
RE: AC Dimmer with OpenHab
@FotoFieber I got a circuit working, But I don't know how to write the code to dim, and work remotely. I used BT137 Triacs instead of TIC206 because of availability. The main issue I had with a 110V/60Hz was the resistor pulling the zerocross Pin high, It would not work but when I removed the resistor all functional?! The schematic I used it was from Inmojo here it is http://bit.ly/1C1xCOS
It works well the only adjustments needed is how low and how high you dim the circuit. Now the code part is what I need. I will give the above code a spin, It looks like the dimmer mirror code from @petewill. -
RE: 'MySensoring' a Kidde Smoke Detector. (Completed)
@ServiceXp You're so smart
I didn't even think about the alarms being interconnected, thanks for saving my extra radios, Arduinos, and wasted time much appreciated. The only thing I might do is change the radio to the hardwired alarm.
-
RE: 'MySensoring' a Kidde Smoke Detector. (Completed)
Ok all is Good! I just used the octocoupler minus the perf board,also as a side note I changed the resistor you showed to a 4.7K to keep the current of the speaker from being drowned out, I also added a power header.
and here is the Modded One!
Thanks again @ServiceXp for all the hard work you did to get us this mod. Now 9 more to go! But I will wait and test this one out for a couple of months or maybe I should just build 5 more for now. Any Ideas on how to make this run on external power until power is lost?
-
RE: 'MySensoring' a Kidde Smoke Detector. (Completed)
@ServiceXp One last question I think, are you picking up the speaker on the center wire it is @ 4V7 and drops down to -3 or so. where did you find 3V3 speaker connection?
Thanks Almost done! -
RE: 'MySensoring' a Kidde Smoke Detector. (Completed)
@ServiceXp Cool I just tested it and was ready build my first unit. I will post after I do it tonight! Have you tried to sniff the Jumpers for signals(like the CO2 or Smoke)? You said you are using the built in battery low detection are you talking about the warning that the batteries need to be replaced locally or remote? Oh Nevermind it will alarm so you will know remote and audible siren and voice local.
The 3V3 off the board proves to be clean enough? I read somewhere that that might be a problem.
Thanks
-
RE: 'MySensoring' a Kidde Smoke Detector. (Completed)
@ServiceXp Are you grabbing the 4V8 and inputting to 3V3 arduino RAW pin? Or are you grabbing the power off J3 Pin1 and 2 at 3V3? Did you make your Arduino less Power hungry i.e. remove LEDs, Regulator, and such? How is the battery life so far? Sorry lots of questions I want to build this for my whole house like 10 of them! But gotta start from some where. I'm thinking most of them are A/C powered in my house so a 5V power supply with a regulator for the detector would work also. Please Let me know how yours is working so far.
Thanks! Great Job! -
RE: How can I tell if my arduino mini pro is 3.3V or 5v?
@BulldogLowell Thanks your determining way is the best way to check a Pro mini with no USB! You can just use the programming cable connected to RAW and check the VCC it will be slightly less like 4V7 or 3V2 but close enough.
-
RE: Backlit Dimmable LED Mirror with Motion Sensor
@henno That is too funny I was at a hotel and they had LEDs under the cabinets with motion sensors to light the floor. So when I came home I knew I saw some guy doing something similar to a mirror that I'm gonna steal for under my bed to walk at night without bumping my toes! Thanks Pete!
-
Newb trying to build a 3 unit dimmer with control
I want to build a sensor with 3 dimmers controlled via 2 switches each for a total of 6 switches (one up and another down) is this possible also? I also want to have temp and humidity on there via DHT11, almost forgot Motion sensor with HRC-501 too. I setup a sketch with Motion and Dimmer without switches for 1 dimmer and it worked! I truely need some direction on how to do this. How I got to this point was to hodgepodge together two example scripts and it worked. I'm sure there is a example on the forum somewhere but I can not find so any help would be great. I'm still trying to understand the child thing on the sensors, when I add a relay and dimmer I lose the dimming function I think because the relay uses the light name?
I love these sensors they integrate better than I expected. I have been looking at these for about 1-2years or even longer! Thinking I was gonna add them but thought that the way I was doing it before was easy enough and robust enough. I was using XBees and I like the distance possible with these radios, what sparked my interest was I was seriously trying to get ESP8266 to do what I wanted and I ended up back here so the fun begins! -
RE: AC Dimmer with OpenHab
@quocanhcgd Can you share the A/C dimmer design? I'm trying to do the same. Found many examples but building a circuit with a triac and zerocrossing.
Thanks,
Jeff -
RE: ArduinoProMini to nRF24L patch PCB with battery holder and Switch
I was just looking at this board on OSHpark last night and was wondering who did it, kudos I might have to do some surface mount soldering, time to build my pickup tool. Your project and another I uploaded to OSHpark required surface mount and I was holding off til now!
Thanks,
Jeff